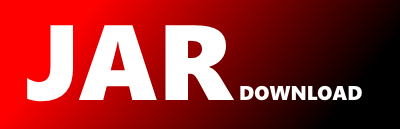
fi.evolver.utils.arg.EnumArg Maven / Gradle / Ivy
package fi.evolver.utils.arg;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class EnumArg> extends Arg {
private final Class type;
private final Method parseMethod;
private final List options;
public EnumArg(Class type, String name) {
this(type, name, true, null);
}
public EnumArg(Class type, String name, T defaultValue) {
this(type, name, false, defaultValue);
}
private EnumArg(Class type, String name, boolean required, T defaultValue) {
super(type, name, required, defaultValue);
this.type = type;
this.options = listOptions(type);
this.parseMethod = getValueOfMethod(type);
}
@Override
public String getType() {
return String.class.getName();
}
public List getOptions() {
return options;
}
@Override
protected T convert(InputStream in) throws IOException {
String value = readUtf8Value(in);
try {
return type.cast(parseMethod.invoke(null, value));
}
catch (IllegalArgumentException e) {
throw new IllegalArgumentException(String.format("Invalid value '%s' for enum %s", value, type.getSimpleName()));
}
catch (IllegalAccessException | InvocationTargetException e) {
throw new IllegalArgumentException("Could not get valueOf method for enum class " + type.getSimpleName(), e);
}
}
private List listOptions(Class type) {
try {
return Arrays.stream((Enum>[])type.getMethod("values").invoke(null))
.map(Enum::name)
.collect(Collectors.toList());
}
catch (NoSuchMethodException | SecurityException | IllegalAccessException | InvocationTargetException e) {
throw new IllegalArgumentException("Could not list possible values for enum class " + type.getSimpleName(), e);
}
}
private Method getValueOfMethod(Class type) {
try {
return type.getMethod("valueOf", String.class);
}
catch (NoSuchMethodException | SecurityException e) {
throw new IllegalArgumentException("Could not get valueOf method for enum class " + type.getSimpleName(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy