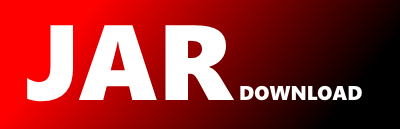
fi.evolver.utils.collection.CollectionUtils Maven / Gradle / Ivy
package fi.evolver.utils.collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
public class CollectionUtils {
private CollectionUtils() { }
/**
* Null safe method for getting the first element of a list.
*
* @param list The list.
* @return The first element of the list if available.
*/
public static Optional first(List list) {
return Optional.ofNullable(list)
.filter(l -> !l.isEmpty())
.map(l -> l.get(0));
}
/**
* Null safe method for getting the last element of a list.
*
* @param list The list.
* @return The last element of the list if available.
*/
public static Optional last(List list) {
return Optional.ofNullable(list)
.filter(l -> !l.isEmpty())
.map(l -> l.get(l.size() - 1));
}
public static boolean isNullOrEmpty(Collection> collection) {
return collection == null || collection.isEmpty();
}
/**
* Null-safe way to check if a collection contains elements.
* @param collection the collection to check
* @return true if the collection contains elements, false if it is null or empty
*/
public static boolean hasElements(Collection> collection) {
return !isNullOrEmpty(collection);
}
/**
* Creates a list with the elements of the first parameter in iteration order except for any elements found in the
* second parameter.
*/
public static List minus(List extends T> original, Collection extends T> excluded) {
List result = new ArrayList<>(original);
result.removeAll(excluded);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy