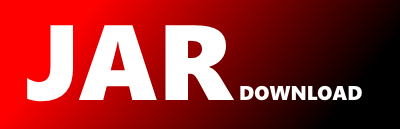
fi.evolver.utils.format.FormatUtils Maven / Gradle / Ivy
package fi.evolver.utils.format;
import static fi.evolver.utils.NullSafetyUtils.denull;
public class FormatUtils {
private static final char[] HEX_ARRAY = "0123456789ABCDEF".toCharArray();
private FormatUtils() {}
public static String truncate(String value, int maxLength) {
if (value == null)
return null;
return value.length() <= maxLength ? value : value.substring(0, Math.max(maxLength, 0));
}
public static String truncatePretty(String value, int maxLength) {
return truncatePretty(value, maxLength, "...", "?");
}
public static String truncatePretty(String value, int maxLength, String suffix, String defaultValue) {
String result = denull(value, defaultValue);
if (result == null)
return null;
if (result.length() > maxLength)
result = truncate(truncate(value, maxLength - suffix.length()) + denull(suffix, "..."), maxLength);
return result;
}
/**
* Safe String.format, which handles invalid arguments etc gracefully.
*
* @param message Base message.
* @param args Arguments for the message.
* @return Formatted result or null, if message was null.
*/
public static String format(String message, Object... args) {
if (message == null)
return null;
if (args == null || args.length == 0)
return message;
try {
return String.format(message, args);
}
catch (RuntimeException e) {
return message + ": " + args;
}
}
public static String toHex(byte[] bytes) {
char[] hexChars = new char[bytes.length * 2];
for (int j = 0; j < bytes.length; j++) {
int v = bytes[j] & 0xFF;
hexChars[j * 2] = HEX_ARRAY[v >>> 4];
hexChars[j * 2 + 1] = HEX_ARRAY[v & 0x0F];
}
return new String(hexChars);
}
public static String padLeft(String word, int length, char c) {
if (word == null)
return null;
StringBuilder builder = new StringBuilder();
for (int i = length - word.length(); i > 0; i--) {
builder.append(c);
}
builder.append(word);
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy