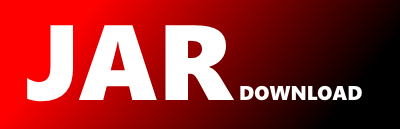
fi.evolver.utils.timing.TimingUtils Maven / Gradle / Ivy
package fi.evolver.utils.timing;
public class TimingUtils {
private static final ThreadLocal watchHolder = new ThreadLocal<>();
private static final int MAX_DEPTH = 10;
private TimingUtils() { }
private static GroupingStopWatch getWatch() {
GroupingStopWatch watch = watchHolder.get();
if (watch == null) {
watch = new GroupingStopWatch();
watchHolder.set(watch);
}
return watch;
}
public static GroupingStopWatch destroyTimer() {
GroupingStopWatch watch = getWatch();
watch.end();
watchHolder.remove();
return watch;
}
public static AutoCloser createTimer() {
watchHolder.remove();
return new AutoCloser(getWatch(), 0);
}
public static AutoCloser begin(String task, String... subTasks) {
GroupingStopWatch watch = getWatch();
int depth = watch.getDepth();
if (depth < MAX_DEPTH)
watch.begin(task);
for (String subTask: subTasks) {
if (depth >= MAX_DEPTH)
break;
watch.begin(subTask);
}
return new AutoCloser(watch, depth);
}
public static class AutoCloser implements AutoCloseable {
private final GroupingStopWatch watch;
private final int targetDepth;
public AutoCloser(GroupingStopWatch watch, int targetDepth) {
this.watch = watch;
this.targetDepth = targetDepth;
}
@Override
public void close() {
while (watch.getDepth() > targetDepth)
watch.end();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy