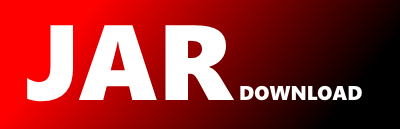
fi.otavanopisto.kuntaapi.server.rest.model.Service Maven / Gradle / Ivy
package fi.otavanopisto.kuntaapi.server.rest.model;
import fi.otavanopisto.kuntaapi.server.rest.model.LocalizedValue;
import java.util.ArrayList;
import java.util.List;
import io.swagger.annotations.*;
import java.util.Objects;
public class Service {
private String id = null;
private List shortDescriptions = new ArrayList();
private List descriptions = new ArrayList();
private List serviceUserInstructions = new ArrayList();
private List names = new ArrayList();
private List alternativeNames = new ArrayList();
private List classIds = new ArrayList();
private List electronicChannelIds = new ArrayList();
private List phoneChannelIds = new ArrayList();
private List printableFormChannelIds = new ArrayList();
private List serviceLocationChannelIds = new ArrayList();
private List webpageChannelIds = new ArrayList();
/**
* Unique identifier representing a specific service.
**/
public Service id(String id) {
this.id = id;
return this;
}
@ApiModelProperty(example = "null", value = "Unique identifier representing a specific service.")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
/**
**/
public Service shortDescriptions(List shortDescriptions) {
this.shortDescriptions = shortDescriptions;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getShortDescriptions() {
return shortDescriptions;
}
public void setShortDescriptions(List shortDescriptions) {
this.shortDescriptions = shortDescriptions;
}
/**
**/
public Service descriptions(List descriptions) {
this.descriptions = descriptions;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getDescriptions() {
return descriptions;
}
public void setDescriptions(List descriptions) {
this.descriptions = descriptions;
}
/**
**/
public Service serviceUserInstructions(List serviceUserInstructions) {
this.serviceUserInstructions = serviceUserInstructions;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getServiceUserInstructions() {
return serviceUserInstructions;
}
public void setServiceUserInstructions(List serviceUserInstructions) {
this.serviceUserInstructions = serviceUserInstructions;
}
/**
* Name of the service.
**/
public Service names(List names) {
this.names = names;
return this;
}
@ApiModelProperty(example = "null", value = "Name of the service.")
public List getNames() {
return names;
}
public void setNames(List names) {
this.names = names;
}
/**
* Name of the service.
**/
public Service alternativeNames(List alternativeNames) {
this.alternativeNames = alternativeNames;
return this;
}
@ApiModelProperty(example = "null", value = "Name of the service.")
public List getAlternativeNames() {
return alternativeNames;
}
public void setAlternativeNames(List alternativeNames) {
this.alternativeNames = alternativeNames;
}
/**
* List of service classes
**/
public Service classIds(List classIds) {
this.classIds = classIds;
return this;
}
@ApiModelProperty(example = "null", value = "List of service classes")
public List getClassIds() {
return classIds;
}
public void setClassIds(List classIds) {
this.classIds = classIds;
}
/**
**/
public Service electronicChannelIds(List electronicChannelIds) {
this.electronicChannelIds = electronicChannelIds;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getElectronicChannelIds() {
return electronicChannelIds;
}
public void setElectronicChannelIds(List electronicChannelIds) {
this.electronicChannelIds = electronicChannelIds;
}
/**
**/
public Service phoneChannelIds(List phoneChannelIds) {
this.phoneChannelIds = phoneChannelIds;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getPhoneChannelIds() {
return phoneChannelIds;
}
public void setPhoneChannelIds(List phoneChannelIds) {
this.phoneChannelIds = phoneChannelIds;
}
/**
**/
public Service printableFormChannelIds(List printableFormChannelIds) {
this.printableFormChannelIds = printableFormChannelIds;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getPrintableFormChannelIds() {
return printableFormChannelIds;
}
public void setPrintableFormChannelIds(List printableFormChannelIds) {
this.printableFormChannelIds = printableFormChannelIds;
}
/**
**/
public Service serviceLocationChannelIds(List serviceLocationChannelIds) {
this.serviceLocationChannelIds = serviceLocationChannelIds;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getServiceLocationChannelIds() {
return serviceLocationChannelIds;
}
public void setServiceLocationChannelIds(List serviceLocationChannelIds) {
this.serviceLocationChannelIds = serviceLocationChannelIds;
}
/**
**/
public Service webpageChannelIds(List webpageChannelIds) {
this.webpageChannelIds = webpageChannelIds;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getWebpageChannelIds() {
return webpageChannelIds;
}
public void setWebpageChannelIds(List webpageChannelIds) {
this.webpageChannelIds = webpageChannelIds;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Service service = (Service) o;
return Objects.equals(id, service.id) &&
Objects.equals(shortDescriptions, service.shortDescriptions) &&
Objects.equals(descriptions, service.descriptions) &&
Objects.equals(serviceUserInstructions, service.serviceUserInstructions) &&
Objects.equals(names, service.names) &&
Objects.equals(alternativeNames, service.alternativeNames) &&
Objects.equals(classIds, service.classIds) &&
Objects.equals(electronicChannelIds, service.electronicChannelIds) &&
Objects.equals(phoneChannelIds, service.phoneChannelIds) &&
Objects.equals(printableFormChannelIds, service.printableFormChannelIds) &&
Objects.equals(serviceLocationChannelIds, service.serviceLocationChannelIds) &&
Objects.equals(webpageChannelIds, service.webpageChannelIds);
}
@Override
public int hashCode() {
return Objects.hash(id, shortDescriptions, descriptions, serviceUserInstructions, names, alternativeNames, classIds, electronicChannelIds, phoneChannelIds, printableFormChannelIds, serviceLocationChannelIds, webpageChannelIds);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Service {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" shortDescriptions: ").append(toIndentedString(shortDescriptions)).append("\n");
sb.append(" descriptions: ").append(toIndentedString(descriptions)).append("\n");
sb.append(" serviceUserInstructions: ").append(toIndentedString(serviceUserInstructions)).append("\n");
sb.append(" names: ").append(toIndentedString(names)).append("\n");
sb.append(" alternativeNames: ").append(toIndentedString(alternativeNames)).append("\n");
sb.append(" classIds: ").append(toIndentedString(classIds)).append("\n");
sb.append(" electronicChannelIds: ").append(toIndentedString(electronicChannelIds)).append("\n");
sb.append(" phoneChannelIds: ").append(toIndentedString(phoneChannelIds)).append("\n");
sb.append(" printableFormChannelIds: ").append(toIndentedString(printableFormChannelIds)).append("\n");
sb.append(" serviceLocationChannelIds: ").append(toIndentedString(serviceLocationChannelIds)).append("\n");
sb.append(" webpageChannelIds: ").append(toIndentedString(webpageChannelIds)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy