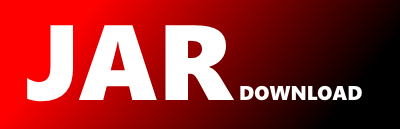
fi.otavanopisto.kuntaapi.server.rest.model.ServiceChannelCommon Maven / Gradle / Ivy
package fi.otavanopisto.kuntaapi.server.rest.model;
import fi.otavanopisto.kuntaapi.server.rest.model.LocalizedValue;
import fi.otavanopisto.kuntaapi.server.rest.model.ServiceChannelServiceHour;
import fi.otavanopisto.kuntaapi.server.rest.model.ServiceChannelSupport;
import fi.otavanopisto.kuntaapi.server.rest.model.ServiceChannelWebPage;
import java.util.ArrayList;
import java.util.List;
import io.swagger.annotations.*;
import java.util.Objects;
public class ServiceChannelCommon {
private String id = null;
private List names = new ArrayList();
private List descriptions = new ArrayList();
private List shortDescriptions = new ArrayList();
private List webPages = new ArrayList();
private List serviceHours = new ArrayList();
private String serviceHoursAdditinalInformation = null;
private List supportContacts = new ArrayList();
/**
**/
public ServiceChannelCommon id(String id) {
this.id = id;
return this;
}
@ApiModelProperty(example = "null", value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
/**
**/
public ServiceChannelCommon names(List names) {
this.names = names;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getNames() {
return names;
}
public void setNames(List names) {
this.names = names;
}
/**
**/
public ServiceChannelCommon descriptions(List descriptions) {
this.descriptions = descriptions;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getDescriptions() {
return descriptions;
}
public void setDescriptions(List descriptions) {
this.descriptions = descriptions;
}
/**
**/
public ServiceChannelCommon shortDescriptions(List shortDescriptions) {
this.shortDescriptions = shortDescriptions;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getShortDescriptions() {
return shortDescriptions;
}
public void setShortDescriptions(List shortDescriptions) {
this.shortDescriptions = shortDescriptions;
}
/**
**/
public ServiceChannelCommon webPages(List webPages) {
this.webPages = webPages;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getWebPages() {
return webPages;
}
public void setWebPages(List webPages) {
this.webPages = webPages;
}
/**
**/
public ServiceChannelCommon serviceHours(List serviceHours) {
this.serviceHours = serviceHours;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getServiceHours() {
return serviceHours;
}
public void setServiceHours(List serviceHours) {
this.serviceHours = serviceHours;
}
/**
**/
public ServiceChannelCommon serviceHoursAdditinalInformation(String serviceHoursAdditinalInformation) {
this.serviceHoursAdditinalInformation = serviceHoursAdditinalInformation;
return this;
}
@ApiModelProperty(example = "null", value = "")
public String getServiceHoursAdditinalInformation() {
return serviceHoursAdditinalInformation;
}
public void setServiceHoursAdditinalInformation(String serviceHoursAdditinalInformation) {
this.serviceHoursAdditinalInformation = serviceHoursAdditinalInformation;
}
/**
**/
public ServiceChannelCommon supportContacts(List supportContacts) {
this.supportContacts = supportContacts;
return this;
}
@ApiModelProperty(example = "null", value = "")
public List getSupportContacts() {
return supportContacts;
}
public void setSupportContacts(List supportContacts) {
this.supportContacts = supportContacts;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ServiceChannelCommon serviceChannelCommon = (ServiceChannelCommon) o;
return Objects.equals(id, serviceChannelCommon.id) &&
Objects.equals(names, serviceChannelCommon.names) &&
Objects.equals(descriptions, serviceChannelCommon.descriptions) &&
Objects.equals(shortDescriptions, serviceChannelCommon.shortDescriptions) &&
Objects.equals(webPages, serviceChannelCommon.webPages) &&
Objects.equals(serviceHours, serviceChannelCommon.serviceHours) &&
Objects.equals(serviceHoursAdditinalInformation, serviceChannelCommon.serviceHoursAdditinalInformation) &&
Objects.equals(supportContacts, serviceChannelCommon.supportContacts);
}
@Override
public int hashCode() {
return Objects.hash(id, names, descriptions, shortDescriptions, webPages, serviceHours, serviceHoursAdditinalInformation, supportContacts);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ServiceChannelCommon {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" names: ").append(toIndentedString(names)).append("\n");
sb.append(" descriptions: ").append(toIndentedString(descriptions)).append("\n");
sb.append(" shortDescriptions: ").append(toIndentedString(shortDescriptions)).append("\n");
sb.append(" webPages: ").append(toIndentedString(webPages)).append("\n");
sb.append(" serviceHours: ").append(toIndentedString(serviceHours)).append("\n");
sb.append(" serviceHoursAdditinalInformation: ").append(toIndentedString(serviceHoursAdditinalInformation)).append("\n");
sb.append(" supportContacts: ").append(toIndentedString(supportContacts)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy