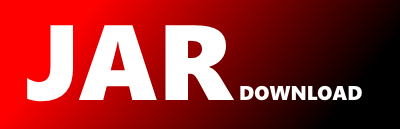
com.founder.core.domain.IeInterfaceBS002 Maven / Gradle / Ivy
package com.founder.core.domain;
import java.io.Serializable;
import java.util.Date;
public class IeInterfaceBS002 implements Serializable {
private String id;
private Date opera_date;
private String code_type;
private String visit_id;
private String apply_unit_id;
private String send_sys_id;
private String order_exec_id;
private String extend_sub_id;
private String msg_id;
private String msg_create_date;
private String acceptAckCode;
private String msg_type;
private String domain_id;
private String patient_id;
private String p_bar_code;
private String ward_code_name;
private String ward_code;
private String bed_no;
private String social_no;
private String name;
private String home_tel;
private String sex;
private String birthday;
private String age;
private String home_street;
private String marry_code;
private String nation_code;
private String occupation_type;
private String occupation_type_name;
private String country_code;
private String country_code_name;
private String relation_tel;
private String relation_name;
private String apply_unit;
private String apply_unit_name;
private String hospitalID;
private String hospitalName;
private String enter_date;
private String apply_doctor;
private String apply_doctor_name;
private String confirm_date;
private String confirm_opera;
private String confirm_opera_name;
private Integer times;
private String ghSerialNo;
private String visit_type_code;
private String visit_type_name;
private String diag_type_code;
private String diag_type_name;
private String diag_input_date;
private String diag_code;
private String diag_str;
private Integer apply_serial;
private String exam_content;
private String exam_request_date;
private String samp_bar_code;
private String samp_content;
private String exam_exec_date;
private String exec_unit;
private String exec_unit_name;
private Integer exam_serial;
private String exam_sub_type;
private String exam_sub_type_name;
private String exam_freq_code;
private String exam_freq_name;
private String exam_type;
private String exam_type_name;
private String exam_region;
private String exam_region_name;
private String exam_method;
private String exam_method_name;
private String exam_method_type;
private String exam_method_type_name;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Date getOpera_date() {
return opera_date;
}
public void setOpera_date(Date opera_date) {
this.opera_date = opera_date;
}
public String getCode_type() {
return code_type;
}
public void setCode_type(String code_type) {
this.code_type = code_type;
}
public String getVisit_id() {
return visit_id;
}
public void setVisit_id(String visit_id) {
this.visit_id = visit_id;
}
public String getApply_unit_id() {
return apply_unit_id;
}
public void setApply_unit_id(String apply_unit_id) {
this.apply_unit_id = apply_unit_id;
}
public String getSend_sys_id() {
return send_sys_id;
}
public void setSend_sys_id(String send_sys_id) {
this.send_sys_id = send_sys_id;
}
public String getOrder_exec_id() {
return order_exec_id;
}
public void setOrder_exec_id(String order_exec_id) {
this.order_exec_id = order_exec_id;
}
public String getExtend_sub_id() {
return extend_sub_id;
}
public void setExtend_sub_id(String extend_sub_id) {
this.extend_sub_id = extend_sub_id;
}
public String getMsg_id() {
return msg_id;
}
public void setMsg_id(String msg_id) {
this.msg_id = msg_id;
}
public String getMsg_create_date() {
return msg_create_date;
}
public void setMsg_create_date(String msg_create_date) {
this.msg_create_date = msg_create_date;
}
public String getAcceptAckCode() {
return acceptAckCode;
}
public void setAcceptAckCode(String acceptAckCode) {
this.acceptAckCode = acceptAckCode;
}
public String getMsg_type() {
return msg_type;
}
public void setMsg_type(String msg_type) {
this.msg_type = msg_type;
}
public String getDomain_id() {
return domain_id;
}
public void setDomain_id(String domain_id) {
this.domain_id = domain_id;
}
public String getPatient_id() {
return patient_id;
}
public void setPatient_id(String patient_id) {
this.patient_id = patient_id;
}
public String getP_bar_code() {
return p_bar_code;
}
public void setP_bar_code(String p_bar_code) {
this.p_bar_code = p_bar_code;
}
public String getWard_code_name() {
return ward_code_name;
}
public void setWard_code_name(String ward_code_name) {
this.ward_code_name = ward_code_name;
}
public String getWard_code() {
return ward_code;
}
public void setWard_code(String ward_code) {
this.ward_code = ward_code;
}
public String getBed_no() {
return bed_no;
}
public void setBed_no(String bed_no) {
this.bed_no = bed_no;
}
public String getSocial_no() {
return social_no;
}
public void setSocial_no(String social_no) {
this.social_no = social_no;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getHome_tel() {
return home_tel;
}
public void setHome_tel(String home_tel) {
this.home_tel = home_tel;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
public String getHome_street() {
return home_street;
}
public void setHome_street(String home_street) {
this.home_street = home_street;
}
public String getMarry_code() {
return marry_code;
}
public void setMarry_code(String marry_code) {
this.marry_code = marry_code;
}
public String getNation_code() {
return nation_code;
}
public void setNation_code(String nation_code) {
this.nation_code = nation_code;
}
public String getOccupation_type() {
return occupation_type;
}
public void setOccupation_type(String occupation_type) {
this.occupation_type = occupation_type;
}
public String getOccupation_type_name() {
return occupation_type_name;
}
public void setOccupation_type_name(String occupation_type_name) {
this.occupation_type_name = occupation_type_name;
}
public String getCountry_code() {
return country_code;
}
public void setCountry_code(String country_code) {
this.country_code = country_code;
}
public String getCountry_code_name() {
return country_code_name;
}
public void setCountry_code_name(String country_code_name) {
this.country_code_name = country_code_name;
}
public String getRelation_tel() {
return relation_tel;
}
public void setRelation_tel(String relation_tel) {
this.relation_tel = relation_tel;
}
public String getRelation_name() {
return relation_name;
}
public void setRelation_name(String relation_name) {
this.relation_name = relation_name;
}
public String getApply_unit() {
return apply_unit;
}
public void setApply_unit(String apply_unit) {
this.apply_unit = apply_unit;
}
public String getApply_unit_name() {
return apply_unit_name;
}
public void setApply_unit_name(String apply_unit_name) {
this.apply_unit_name = apply_unit_name;
}
public String getHospitalID() {
return hospitalID;
}
public void setHospitalID(String hospitalID) {
this.hospitalID = hospitalID;
}
public String getHospitalName() {
return hospitalName;
}
public void setHospitalName(String hospitalName) {
this.hospitalName = hospitalName;
}
public String getEnter_date() {
return enter_date;
}
public void setEnter_date(String enter_date) {
this.enter_date = enter_date;
}
public String getApply_doctor() {
return apply_doctor;
}
public void setApply_doctor(String apply_doctor) {
this.apply_doctor = apply_doctor;
}
public String getApply_doctor_name() {
return apply_doctor_name;
}
public void setApply_doctor_name(String apply_doctor_name) {
this.apply_doctor_name = apply_doctor_name;
}
public String getConfirm_date() {
return confirm_date;
}
public void setConfirm_date(String confirm_date) {
this.confirm_date = confirm_date;
}
public String getConfirm_opera() {
return confirm_opera;
}
public void setConfirm_opera(String confirm_opera) {
this.confirm_opera = confirm_opera;
}
public String getConfirm_opera_name() {
return confirm_opera_name;
}
public void setConfirm_opera_name(String confirm_opera_name) {
this.confirm_opera_name = confirm_opera_name;
}
public Integer getTimes() {
return times;
}
public void setTimes(Integer times) {
this.times = times;
}
public String getGhSerialNo() {
return ghSerialNo;
}
public void setGhSerialNo(String ghSerialNo) {
this.ghSerialNo = ghSerialNo;
}
public String getVisit_type_code() {
return visit_type_code;
}
public void setVisit_type_code(String visit_type_code) {
this.visit_type_code = visit_type_code;
}
public String getVisit_type_name() {
return visit_type_name;
}
public void setVisit_type_name(String visit_type_name) {
this.visit_type_name = visit_type_name;
}
public String getDiag_type_code() {
return diag_type_code;
}
public void setDiag_type_code(String diag_type_code) {
this.diag_type_code = diag_type_code;
}
public String getDiag_type_name() {
return diag_type_name;
}
public void setDiag_type_name(String diag_type_name) {
this.diag_type_name = diag_type_name;
}
public String getDiag_input_date() {
return diag_input_date;
}
public void setDiag_input_date(String diag_input_date) {
this.diag_input_date = diag_input_date;
}
public String getDiag_code() {
return diag_code;
}
public void setDiag_code(String diag_code) {
this.diag_code = diag_code;
}
public String getDiag_str() {
return diag_str;
}
public void setDiag_str(String diag_str) {
this.diag_str = diag_str;
}
public Integer getApply_serial() {
return apply_serial;
}
public void setApply_serial(Integer apply_serial) {
this.apply_serial = apply_serial;
}
public String getExam_content() {
return exam_content;
}
public void setExam_content(String exam_content) {
this.exam_content = exam_content;
}
public String getExam_request_date() {
return exam_request_date;
}
public void setExam_request_date(String exam_request_date) {
this.exam_request_date = exam_request_date;
}
public String getSamp_bar_code() {
return samp_bar_code;
}
public void setSamp_bar_code(String samp_bar_code) {
this.samp_bar_code = samp_bar_code;
}
public String getSamp_content() {
return samp_content;
}
public void setSamp_content(String samp_content) {
this.samp_content = samp_content;
}
public String getExam_exec_date() {
return exam_exec_date;
}
public void setExam_exec_date(String exam_exec_date) {
this.exam_exec_date = exam_exec_date;
}
public String getExec_unit() {
return exec_unit;
}
public void setExec_unit(String exec_unit) {
this.exec_unit = exec_unit;
}
public String getExec_unit_name() {
return exec_unit_name;
}
public void setExec_unit_name(String exec_unit_name) {
this.exec_unit_name = exec_unit_name;
}
public Integer getExam_serial() {
return exam_serial;
}
public void setExam_serial(Integer exam_serial) {
this.exam_serial = exam_serial;
}
public String getExam_sub_type() {
return exam_sub_type;
}
public void setExam_sub_type(String exam_sub_type) {
this.exam_sub_type = exam_sub_type;
}
public String getExam_sub_type_name() {
return exam_sub_type_name;
}
public void setExam_sub_type_name(String exam_sub_type_name) {
this.exam_sub_type_name = exam_sub_type_name;
}
public String getExam_freq_code() {
return exam_freq_code;
}
public void setExam_freq_code(String exam_freq_code) {
this.exam_freq_code = exam_freq_code;
}
public String getExam_freq_name() {
return exam_freq_name;
}
public void setExam_freq_name(String exam_freq_name) {
this.exam_freq_name = exam_freq_name;
}
public String getExam_type() {
return exam_type;
}
public void setExam_type(String exam_type) {
this.exam_type = exam_type;
}
public String getExam_type_name() {
return exam_type_name;
}
public void setExam_type_name(String exam_type_name) {
this.exam_type_name = exam_type_name;
}
public String getExam_region() {
return exam_region;
}
public void setExam_region(String exam_region) {
this.exam_region = exam_region;
}
public String getExam_region_name() {
return exam_region_name;
}
public void setExam_region_name(String exam_region_name) {
this.exam_region_name = exam_region_name;
}
public String getExam_method() {
return exam_method;
}
public void setExam_method(String exam_method) {
this.exam_method = exam_method;
}
public String getExam_method_name() {
return exam_method_name;
}
public void setExam_method_name(String exam_method_name) {
this.exam_method_name = exam_method_name;
}
public String getExam_method_type() {
return exam_method_type;
}
public void setExam_method_type(String exam_method_type) {
this.exam_method_type = exam_method_type;
}
public String getExam_method_type_name() {
return exam_method_type_name;
}
public void setExam_method_type_name(String exam_method_type_name) {
this.exam_method_type_name = exam_method_type_name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy