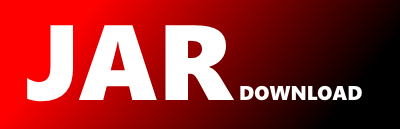
com.founder.core.domain.IeInterfaceBS303 Maven / Gradle / Ivy
package com.founder.core.domain;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.Date;
public class IeInterfaceBS303 implements Serializable {
private String id;
private Date opera_date;
private String code_type;
private String visit_id;
private String apply_unit_id;
private String send_sys_id;
private String order_exec_id;
private String extend_sub_id;
private String msg_create_date;
private String msg_id;
private String msg_type;
private String domain_id;
private String patient_id;
private String p_bar_code;
private String name;
private String sex;
private String birthday;
private String age;
private String apply_unit;
private String apply_unit_name;
private String hospitalID;
private String hospitalName;
private Integer times;
private String ghSerialNo;
private String visit_type_code;
private String visit_type_name;
private Integer item_no;
private String yz_no;
private String order_no;
private String order_type;
private String order_type_name;
private String comment;
private String freq_code;
private String freq_code_name;
private String yz_oldward_name;
private String yz_oldward_code;
private String yz_olddept_code;
private String yz_olddept_name;
private String enter_date;
private String doctor_code;
private String doctor_name;
private String confrim_date;
private String confrim_opera_code;
private String confirm_opera;
private String exec_sn;
private String exec_name;
private String flag_fee_code;
private String flag_fee_value;
private String is_em;
private String is_em_r;
private String isYG;
private String isYGResult;
private String flag_yyt_code;
private BigDecimal charge_amount;
private String pack_serial;
private String yz_code;
private String yz_name;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Date getOpera_date() {
return opera_date;
}
public void setOpera_date(Date opera_date) {
this.opera_date = opera_date;
}
public String getCode_type() {
return code_type;
}
public void setCode_type(String code_type) {
this.code_type = code_type;
}
public String getVisit_id() {
return visit_id;
}
public void setVisit_id(String visit_id) {
this.visit_id = visit_id;
}
public String getApply_unit_id() {
return apply_unit_id;
}
public void setApply_unit_id(String apply_unit_id) {
this.apply_unit_id = apply_unit_id;
}
public String getSend_sys_id() {
return send_sys_id;
}
public void setSend_sys_id(String send_sys_id) {
this.send_sys_id = send_sys_id;
}
public String getOrder_exec_id() {
return order_exec_id;
}
public void setOrder_exec_id(String order_exec_id) {
this.order_exec_id = order_exec_id;
}
public String getExtend_sub_id() {
return extend_sub_id;
}
public void setExtend_sub_id(String extend_sub_id) {
this.extend_sub_id = extend_sub_id;
}
public String getMsg_create_date() {
return msg_create_date;
}
public void setMsg_create_date(String msg_create_date) {
this.msg_create_date = msg_create_date;
}
public String getMsg_id() {
return msg_id;
}
public void setMsg_id(String msg_id) {
this.msg_id = msg_id;
}
public String getMsg_type() {
return msg_type;
}
public void setMsg_type(String msg_type) {
this.msg_type = msg_type;
}
public String getDomain_id() {
return domain_id;
}
public void setDomain_id(String domain_id) {
this.domain_id = domain_id;
}
public String getPatient_id() {
return patient_id;
}
public void setPatient_id(String patient_id) {
this.patient_id = patient_id;
}
public String getP_bar_code() {
return p_bar_code;
}
public void setP_bar_code(String p_bar_code) {
this.p_bar_code = p_bar_code;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
public String getApply_unit() {
return apply_unit;
}
public void setApply_unit(String apply_unit) {
this.apply_unit = apply_unit;
}
public String getApply_unit_name() {
return apply_unit_name;
}
public void setApply_unit_name(String apply_unit_name) {
this.apply_unit_name = apply_unit_name;
}
public String getHospitalID() {
return hospitalID;
}
public void setHospitalID(String hospitalID) {
this.hospitalID = hospitalID;
}
public String getHospitalName() {
return hospitalName;
}
public void setHospitalName(String hospitalName) {
this.hospitalName = hospitalName;
}
public Integer getTimes() {
return times;
}
public void setTimes(Integer times) {
this.times = times;
}
public String getGhSerialNo() {
return ghSerialNo;
}
public void setGhSerialNo(String ghSerialNo) {
this.ghSerialNo = ghSerialNo;
}
public String getVisit_type_code() {
return visit_type_code;
}
public void setVisit_type_code(String visit_type_code) {
this.visit_type_code = visit_type_code;
}
public String getVisit_type_name() {
return visit_type_name;
}
public void setVisit_type_name(String visit_type_name) {
this.visit_type_name = visit_type_name;
}
public Integer getItem_no() {
return item_no;
}
public void setItem_no(Integer item_no) {
this.item_no = item_no;
}
public String getYz_no() {
return yz_no;
}
public void setYz_no(String yz_no) {
this.yz_no = yz_no;
}
public String getOrder_no() {
return order_no;
}
public void setOrder_no(String order_no) {
this.order_no = order_no;
}
public String getOrder_type() {
return order_type;
}
public void setOrder_type(String order_type) {
this.order_type = order_type;
}
public String getOrder_type_name() {
return order_type_name;
}
public void setOrder_type_name(String order_type_name) {
this.order_type_name = order_type_name;
}
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public String getFreq_code() {
return freq_code;
}
public void setFreq_code(String freq_code) {
this.freq_code = freq_code;
}
public String getFreq_code_name() {
return freq_code_name;
}
public void setFreq_code_name(String freq_code_name) {
this.freq_code_name = freq_code_name;
}
public String getYz_oldward_name() {
return yz_oldward_name;
}
public void setYz_oldward_name(String yz_oldward_name) {
this.yz_oldward_name = yz_oldward_name;
}
public String getYz_oldward_code() {
return yz_oldward_code;
}
public void setYz_oldward_code(String yz_oldward_code) {
this.yz_oldward_code = yz_oldward_code;
}
public String getYz_olddept_code() {
return yz_olddept_code;
}
public void setYz_olddept_code(String yz_olddept_code) {
this.yz_olddept_code = yz_olddept_code;
}
public String getYz_olddept_name() {
return yz_olddept_name;
}
public void setYz_olddept_name(String yz_olddept_name) {
this.yz_olddept_name = yz_olddept_name;
}
public String getEnter_date() {
return enter_date;
}
public void setEnter_date(String enter_date) {
this.enter_date = enter_date;
}
public String getDoctor_code() {
return doctor_code;
}
public void setDoctor_code(String doctor_code) {
this.doctor_code = doctor_code;
}
public String getDoctor_name() {
return doctor_name;
}
public void setDoctor_name(String doctor_name) {
this.doctor_name = doctor_name;
}
public String getConfrim_date() {
return confrim_date;
}
public void setConfrim_date(String confrim_date) {
this.confrim_date = confrim_date;
}
public String getConfrim_opera_code() {
return confrim_opera_code;
}
public void setConfrim_opera_code(String confrim_opera_code) {
this.confrim_opera_code = confrim_opera_code;
}
public String getConfirm_opera() {
return confirm_opera;
}
public void setConfirm_opera(String confirm_opera) {
this.confirm_opera = confirm_opera;
}
public String getExec_sn() {
return exec_sn;
}
public void setExec_sn(String exec_sn) {
this.exec_sn = exec_sn;
}
public String getExec_name() {
return exec_name;
}
public void setExec_name(String exec_name) {
this.exec_name = exec_name;
}
public String getFlag_fee_code() {
return flag_fee_code;
}
public void setFlag_fee_code(String flag_fee_code) {
this.flag_fee_code = flag_fee_code;
}
public String getFlag_fee_value() {
return flag_fee_value;
}
public void setFlag_fee_value(String flag_fee_value) {
this.flag_fee_value = flag_fee_value;
}
public String getIs_em() {
return is_em;
}
public void setIs_em(String is_em) {
this.is_em = is_em;
}
public String getIs_em_r() {
return is_em_r;
}
public void setIs_em_r(String is_em_r) {
this.is_em_r = is_em_r;
}
public String getIsYG() {
return isYG;
}
public void setIsYG(String isYG) {
this.isYG = isYG;
}
public String getIsYGResult() {
return isYGResult;
}
public void setIsYGResult(String isYGResult) {
this.isYGResult = isYGResult;
}
public String getFlag_yyt_code() {
return flag_yyt_code;
}
public void setFlag_yyt_code(String flag_yyt_code) {
this.flag_yyt_code = flag_yyt_code;
}
public BigDecimal getCharge_amount() {
return charge_amount;
}
public void setCharge_amount(BigDecimal charge_amount) {
this.charge_amount = charge_amount;
}
public String getPack_serial() {
return pack_serial;
}
public void setPack_serial(String pack_serial) {
this.pack_serial = pack_serial;
}
public String getYz_code() {
return yz_code;
}
public void setYz_code(String yz_code) {
this.yz_code = yz_code;
}
public String getYz_name() {
return yz_name;
}
public void setYz_name(String yz_name) {
this.yz_name = yz_name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy