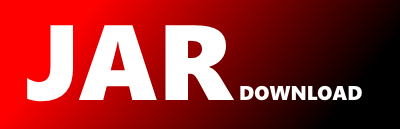
fluximpl.RabbitMQActionImpl Maven / Gradle / Ivy
The newest version!
package fluximpl;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
import com.rabbitmq.client.MessageProperties;
import flux.RabbitMQAction;
import flux.EngineException;
import flux.FlowContext;
import flux.logging.Logger;
import java.io.IOException;
import java.util.*;
/**
* Implementation for RabbitMQAction. Used to publish messages to RabbitMQ.
*
* @author [email protected]
*/
public class RabbitMQActionImpl extends TriggerImpl implements RabbitMQAction {
private static final String ACTION_VARIABLE = "RABBITMQ_ACTION_VARIABLE";
private static final String EXCLUSIVE_TYPE = "EXCLUSIVE";
public RabbitMQActionImpl() {
super(new FlowChartImpl(), "RabbitMQ Action");
}
public RabbitMQActionImpl(FlowChartImpl fc, String name) {
super(fc, name);
}
public Set getHiddenVariableNames() {
// The returned raw type is a Set of String.
@SuppressWarnings("unchecked")
Set set = super.getHiddenVariableNames();
set.add(ACTION_VARIABLE);
return set;
}
public String getHost() {
return getVariable().host;
}
@Override
public void setHost(String host) {
RabbitMQActionVariable var = getVariable();
var.host = host;
putVariable(var);
}
public int getPort() {
return getVariable().port;
}
@Override
public void setPort(int port) {
RabbitMQActionVariable var = getVariable();
var.port = port;
putVariable(var);
}
public String getVirtualHost() {
return getVariable().virtualHost;
}
@Override
public void setVirtualHost(String virtualHost) {
RabbitMQActionVariable var = getVariable();
var.virtualHost = virtualHost;
putVariable(var);
}
public String getUsername() {
return getVariable().username;
}
@Override
public void setUsername(String username) {
RabbitMQActionVariable var = getVariable();
var.username = username;
putVariable(var);
}
public String getPassword() {
Password password = getVariable().password;
if (password != null) {
return password.getEncryptedPassword();
}
return null;
}
@Override
public void setPassword(String password) {
RabbitMQActionVariable var = getVariable();
if (password != null) {
var.password = Password.makePassword(password);
}
putVariable(var);
}
public String getExchangeName() {
return getVariable().exchangeName;
}
@Override
public void setExchangeName(String exchangeName) {
RabbitMQActionVariable var = getVariable();
var.exchangeName = exchangeName;
putVariable(var);
}
public String getRoutingKey() {
return getVariable().routingKey;
}
@Override
public void setRoutingKey(String routingKey) {
RabbitMQActionVariable var = getVariable();
var.routingKey = routingKey;
putVariable(var);
}
public String getMessage() {
return getVariable().message;
}
@Override
public void setMessage(String message) {
RabbitMQActionVariable var = getVariable();
var.message = message;
putVariable(var);
}
public String getQueueName() {
return getVariable().queueName;
}
@Override
public void setQueueName(String queueName) {
RabbitMQActionVariable var = getVariable();
var.queueName = queueName;
putVariable(var);
}
public String getQueueType() {
return getVariable().queueType;
}
@Override
public void setQueueType(String queueType) {
RabbitMQActionVariable var = getVariable();
var.queueType = queueType;
putVariable(var);
}
private boolean isExclusive() {
return getQueueType().equalsIgnoreCase(EXCLUSIVE_TYPE);
}
@Override
public void setExchangeType(String exchangeType) {
RabbitMQActionVariable var = getVariable();
var.exchangeType = exchangeType;
putVariable(var);
}
public String getExchangeType() {
return getVariable().exchangeType;
}
@Override
public Object execute(FlowContext flowContext) throws Exception {
Logger log = flowContext.getLogger();
ConnectionFactory factory = new ConnectionFactory();
factory.setHost(getHost());
factory.setPort(getPort());
if (!StringUtil.isNullOrEmpty(getVirtualHost())) {
factory.setVirtualHost(getVirtualHost());
}
factory.setUsername(getUsername());
factory.setPassword(getPassword());
Connection conn = null;
Channel channel = null;
try {
conn = factory.newConnection();
// Use channel to send and receive messages
channel = conn.createChannel();
if (isExclusive()) {
channel.exchangeDeclare(getExchangeName(), getExchangeType(), true);
String queueName = channel.queueDeclare().getQueue();
channel.queueBind(queueName, getExchangeName(), getRoutingKey());
} else {
channel.exchangeDeclare(getExchangeName(), getExchangeType(), true);
channel.queueDeclare(getQueueName(), true, false, false, null);
channel.queueBind(getQueueName(), getExchangeName(), getRoutingKey());
}
log.info("Publishing message " + getMessage());
byte[] messageBodyBytes = getMessage().getBytes();
channel.basicPublish(getExchangeName(), getRoutingKey(),
MessageProperties.PERSISTENT_TEXT_PLAIN,
messageBodyBytes);
log.info("Message published successfully.");
} catch (IOException exception) {
log.severe("Exception during publish. Reason : " + exception.getMessage());
} finally {
if (channel != null) {
channel.close();
}
if (conn != null) {
conn.close();
}
}
return null;
}
@Override
public void verify() throws EngineException {
if (StringUtil.isNullOrEmpty(getExchangeName())) {
throw new EngineException("Expected \"Exchange name\" to be non-null or non-empty, but it was null or empty.");
}
if (StringUtil.isNullOrEmpty(getMessage())) {
throw new EngineException("Expected \"Message\" to be non-null or non-empty, but it was null or empty.");
}
if (StringUtil.isNullOrEmpty(getExchangeType())) {
throw new EngineException("Expected \"Exchange type\" to be non-null or non-empty, but it was null or empty.");
}
if (!ExchangeType.contains(getExchangeType())) {
throw new EngineException("Invalid \"Exchange type\". Valid values are DIRECT, FANOUT, TOPIC.");
}
verifyCommonProperties();
if (StringUtil.isNullOrEmpty(getQueueType())) {
throw new EngineException("Expected \"Queue type\" to be non-null or non-empty, but it was null or empty.");
}
if (!QueueType.contains(getQueueType())) {
throw new EngineException("Invalid \"Queue type\". Valid values are EXCLUSIVE, SHARED.");
}
if (StringUtil.isNullOrEmpty(getRoutingKey())) {
throw new EngineException("Expected \"Routing key\" to be non-null or non-empty, but it was null or empty. It can be same as the queue name.");
}
}
protected void verifyCommonProperties() throws EngineException {
if (StringUtil.isNullOrEmpty(getHost())) {
throw new EngineException("Expected \"Hostname\" to be non-null or non-empty, but it was null or empty.");
}
if (StringUtil.isNullOrEmpty(getUsername())) {
throw new EngineException("Expected \"Username\" to be non-null or non-empty, but it was null or empty.");
}
if (StringUtil.isNullOrEmpty(getPassword())) {
throw new EngineException("Expected \"Password\" to be non-null or non-empty, but it was null or empty.");
}
if (getPort() < 0 || getPort() > 65535) {
throw new EngineException("Invalid \" port\". Valid values are between 1 and 65535.");
}
if (StringUtil.isNullOrEmpty(getQueueName())) {
throw new EngineException("Expected \"Queue name\" to be non-null or non-empty, but it was null or empty.");
}
}
enum ExchangeType {
DIRECT, FANOUT, TOPIC, HEADERS;
private static final Map stringToEnum = new HashMap();
private static final Set values = new HashSet();
static {
for (ExchangeType type : values()) {
stringToEnum.put(type.toString(), type);
values.add(type.toString());
}
}
public static ExchangeType fromString(String type) {
return stringToEnum.get(type.toUpperCase());
}
public static boolean contains(String type) {
return values.contains(type.toUpperCase());
}
}
enum QueueType {
EXCLUSIVE, SHARED;
private static final Map stringToEnum = new HashMap();
private static final Set values = new HashSet();
static {
for (QueueType type : values()) {
stringToEnum.put(type.toString(), type);
values.add(type.toString());
}
}
public static QueueType fromString(String type) {
return stringToEnum.get(type.toUpperCase());
}
public static boolean contains(String type) {
return values.contains(type.toUpperCase());
}
}
private RabbitMQActionVariable getVariable() {
if (!getVariableManager().contains(ACTION_VARIABLE)) {
getVariableManager().put(ACTION_VARIABLE, new RabbitMQActionVariable());
}
return (RabbitMQActionVariable) getVariableManager().get(ACTION_VARIABLE);
}
private void putVariable(RabbitMQVariable variable) {
getVariableManager().put(ACTION_VARIABLE, variable);
}
@Override
public Date getNextPollingDate() {
//This was added for reusing the implementation between Action and Trigger.
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy