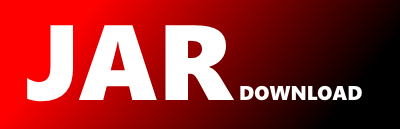
org.aion.avm.EnergyCalculator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javaee-rt Show documentation
Show all versions of javaee-rt Show documentation
An Execution Environment for Java SCOREs
package org.aion.avm;
/**
* This class performs the linear fee calculation for JCL classes.
* If the product of values overflows, Integer.MAX_VALUE is returned.
*/
public class EnergyCalculator {
/**
* @param base base cost
* @param linearValue linear cost
* @return base + linearValue * RT_METHOD_FEE_FACTOR_LEVEL_2
*/
public static int multiplyLinearValueByMethodFeeLevel2AndAddBase(int base, int linearValue) {
return addAndCheckForOverflow(base, multiplyAndCheckForOverflow(linearValue, RuntimeMethodFeeSchedule.RT_METHOD_FEE_FACTOR_LEVEL_2));
}
/**
* @param base base cost
* @param linearValue linear cost
* @return base + linearValue * RT_METHOD_FEE_FACTOR_LEVEL_1
*/
public static int multiplyLinearValueByMethodFeeLevel1AndAddBase(int base, int linearValue) {
return addAndCheckForOverflow(base, multiplyAndCheckForOverflow(linearValue, RuntimeMethodFeeSchedule.RT_METHOD_FEE_FACTOR_LEVEL_1));
}
public static int multiply(int value1, int value2) {
return multiplyAndCheckForOverflow(value1, value2);
}
private static int addAndCheckForOverflow(int value1, int value2) {
long result = (long) value1 + (long) value2;
if (result > Integer.MAX_VALUE) {
return Integer.MAX_VALUE;
} else {
return (int) result;
}
}
private static int multiplyAndCheckForOverflow(int value1, int value2) {
long result = (long) value1 * (long) value2;
if (result > Integer.MAX_VALUE) {
return Integer.MAX_VALUE;
} else {
return (int) result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy