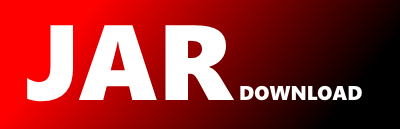
org.aion.avm.RuntimeMethodFeeSchedule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javaee-rt Show documentation
Show all versions of javaee-rt Show documentation
An Execution Environment for Java SCOREs
package org.aion.avm;
public class RuntimeMethodFeeSchedule {
public static final int RT_METHOD_FEE_LEVEL_1 = 100;
public static final int RT_METHOD_FEE_LEVEL_2 = 300;
public static final int RT_METHOD_FEE_LEVEL_3 = 600;
public static final int RT_METHOD_FEE_LEVEL_4 = 1500;
public static final int RT_METHOD_FEE_LEVEL_5 = 3000;
public static final int RT_METHOD_FEE_LEVEL_6 = 5000;
public static final int RT_METHOD_FEE_FACTOR_LEVEL_1 = 1;
public static final int RT_METHOD_FEE_FACTOR_LEVEL_2 = 5;
public static final int BigInteger_avm_constructor = RT_METHOD_FEE_LEVEL_3; // totalCost - 687; // byte code cost - 459; invoked methods cost - 228;
public static final int BigInteger_avm_constructor_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 693; // byte code cost - 465; invoked methods cost - 228;
public static final int BigInteger_avm_constructor_4 = RT_METHOD_FEE_LEVEL_3; // totalCost - 681; // byte code cost - 453; invoked methods cost - 228;
public static final int BigInteger_avm_valueOf = RT_METHOD_FEE_LEVEL_3; // totalCost - 472; // byte code cost - 372; invoked methods cost - 100;
public static final int BigInteger_avm_add = RT_METHOD_FEE_LEVEL_3; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_subtract = RT_METHOD_FEE_LEVEL_3; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_multiply = RT_METHOD_FEE_LEVEL_4; // totalCost - 1390; // byte code cost - 390; invoked methods cost - 1000;
public static final int BigInteger_avm_divide = RT_METHOD_FEE_LEVEL_4; // totalCost - 1390; // byte code cost - 390; invoked methods cost - 1000;
public static final int BigInteger_avm_remainder = RT_METHOD_FEE_LEVEL_4; // totalCost - 1390; // byte code cost - 390; invoked methods cost - 1000;
public static final int BigInteger_avm_sqrt = RT_METHOD_FEE_LEVEL_5; // totalCost - 1878; // byte code cost - 378; invoked methods cost - 1500;
public static final int BigInteger_avm_gcd = RT_METHOD_FEE_LEVEL_4; // totalCost - 2390; // byte code cost - 390; invoked methods cost - 2000;
public static final int BigInteger_avm_abs = RT_METHOD_FEE_LEVEL_3; // totalCost - 478; // byte code cost - 378; invoked methods cost - 100;
public static final int BigInteger_avm_negate = RT_METHOD_FEE_LEVEL_3; // totalCost - 478; // byte code cost - 378; invoked methods cost - 100;
public static final int BigInteger_avm_signum = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int BigInteger_avm_mod = RT_METHOD_FEE_LEVEL_4; // totalCost - 1390; // byte code cost - 390; invoked methods cost - 1000;
public static final int BigInteger_avm_modPow = RT_METHOD_FEE_LEVEL_4; // totalCost - 1902; // byte code cost - 402; invoked methods cost - 1500;
public static final int BigInteger_avm_modInverse = RT_METHOD_FEE_LEVEL_4; // totalCost - 1890; // byte code cost - 390; invoked methods cost - 1500;
public static final int BigInteger_avm_shiftLeft = RT_METHOD_FEE_LEVEL_2; // totalCost - 484; // byte code cost - 384; invoked methods cost - 100;
public static final int BigInteger_avm_shiftRight = RT_METHOD_FEE_LEVEL_2; // totalCost - 484; // byte code cost - 384; invoked methods cost - 100;
public static final int BigInteger_avm_and = RT_METHOD_FEE_LEVEL_2; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_or = RT_METHOD_FEE_LEVEL_2; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_xor = RT_METHOD_FEE_LEVEL_2; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_not = RT_METHOD_FEE_LEVEL_2; // totalCost - 478; // byte code cost - 378; invoked methods cost - 100;
public static final int BigInteger_avm_andNot = RT_METHOD_FEE_LEVEL_3; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_testBit = RT_METHOD_FEE_LEVEL_2; // totalCost - 228; // byte code cost - 128; invoked methods cost - 100;
public static final int BigInteger_avm_setBit = RT_METHOD_FEE_LEVEL_3; // totalCost - 684; // byte code cost - 384; invoked methods cost - 300;
public static final int BigInteger_avm_clearBit = RT_METHOD_FEE_LEVEL_3; // totalCost - 684; // byte code cost - 384; invoked methods cost - 300;
public static final int BigInteger_avm_flipBit = RT_METHOD_FEE_LEVEL_3; // totalCost - 684; // byte code cost - 384; invoked methods cost - 300;
public static final int BigInteger_avm_getLowestSetBit = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 122; invoked methods cost - 300;
public static final int BigInteger_avm_bitLength = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 122; invoked methods cost - 300;
public static final int BigInteger_avm_bitCount = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 122; invoked methods cost - 300;
public static final int BigInteger_avm_compareTo = RT_METHOD_FEE_LEVEL_2; // totalCost - 234; // byte code cost - 134; invoked methods cost - 100;
public static final int BigInteger_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 462; //maximum cost of multiple blocks; // byte code cost - 362; invoked methods cost - 100;
public static final int BigInteger_avm_min = RT_METHOD_FEE_LEVEL_2; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_max = RT_METHOD_FEE_LEVEL_2; // totalCost - 490; // byte code cost - 390; invoked methods cost - 100;
public static final int BigInteger_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int BigInteger_avm_toString = RT_METHOD_FEE_LEVEL_4; // totalCost - 588; // byte code cost - 288; invoked methods cost - 300;
public static final int BigInteger_avm_toString_1 = RT_METHOD_FEE_LEVEL_4; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int BigInteger_avm_toByteArray = RT_METHOD_FEE_LEVEL_2; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int BigInteger_avm_intValue = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int BigInteger_avm_longValue = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int BigInteger_avm_floatValue = RT_METHOD_FEE_LEVEL_2; // totalCost - 322; // byte code cost - 122; invoked methods cost - 200;
public static final int BigInteger_avm_doubleValue = RT_METHOD_FEE_LEVEL_2; // totalCost - 322; // byte code cost - 122; invoked methods cost - 200;
public static final int BigInteger_avm_longValueExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 122; invoked methods cost - 300;
public static final int BigInteger_avm_intValueExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 122; invoked methods cost - 300;
public static final int BigInteger_avm_shortValueExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 122; invoked methods cost - 300;
public static final int BigInteger_avm_byteValueExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 122; invoked methods cost - 300;
public static final int Number_avm_byteValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 118;
public static final int Number_avm_shortValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 118;
public static final int Integer_avm_toString = RT_METHOD_FEE_LEVEL_3; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int Integer_avm_toUnsignedString = RT_METHOD_FEE_LEVEL_3; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int Integer_avm_toHexString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Integer_avm_toOctalString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Integer_avm_toBinaryString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Integer_avm_toString_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Integer_avm_toUnsignedString_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Integer_avm_parseInt = RT_METHOD_FEE_LEVEL_3; // totalCost - 610; // byte code cost - 182; invoked methods cost - 428;
public static final int Integer_avm_parseInt_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 604; // byte code cost - 176; invoked methods cost - 428;
public static final int Integer_avm_parseInt_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 682; // byte code cost - 254; invoked methods cost - 428;
public static final int Integer_avm_parseUnsignedInt = RT_METHOD_FEE_LEVEL_3; // totalCost - 610; // byte code cost - 182; invoked methods cost - 428;
public static final int Integer_avm_parseUnsignedInt_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 604; // byte code cost - 176; invoked methods cost - 428;
public static final int Integer_avm_parseUnsignedInt_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 682; // byte code cost - 254; invoked methods cost - 428;
public static final int Integer_avm_valueOf = RT_METHOD_FEE_LEVEL_3; // totalCost - 182;
public static final int Integer_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 182;
public static final int Integer_avm_valueOf_2 = RT_METHOD_FEE_LEVEL_1; // totalCost - 288;
public static final int Integer_avm_byteValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Integer_avm_shortValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Integer_avm_intValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Integer_avm_longValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Integer_avm_floatValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Integer_avm_doubleValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Integer_avm_toString_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Integer_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Integer_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 56;
public static final int Integer_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 333; //maximum cost of multiple blocks;
public static final int Integer_avm_decode = RT_METHOD_FEE_LEVEL_4; // totalCost - 996; // byte code cost - 468; invoked methods cost - 528;
public static final int Integer_avm_compareTo = RT_METHOD_FEE_LEVEL_1; // totalCost - 134;
public static final int Integer_avm_compare = RT_METHOD_FEE_LEVEL_1; // totalCost - 262; //maximum cost of multiple blocks;
public static final int Integer_avm_compareUnsigned = RT_METHOD_FEE_LEVEL_1; // totalCost - 140;
public static final int Integer_avm_toUnsignedLong = RT_METHOD_FEE_LEVEL_1; // totalCost - 67;
public static final int Integer_avm_divideUnsigned = RT_METHOD_FEE_LEVEL_1; // totalCost - 189;
public static final int Integer_avm_remainderUnsigned = RT_METHOD_FEE_LEVEL_1; // totalCost - 189;
public static final int Integer_avm_highestOneBit = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Integer_avm_lowestOneBit = RT_METHOD_FEE_LEVEL_1; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Integer_avm_numberOfLeadingZeros = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Integer_avm_numberOfTrailingZeros = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Integer_avm_bitCount = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Integer_avm_reverse = RT_METHOD_FEE_LEVEL_2; // totalCost - 218; // byte code cost - 118; invoked methods cost - 100;
public static final int Integer_avm_signum = RT_METHOD_FEE_LEVEL_1; // totalCost - 86;
public static final int Integer_avm_reverseBytes = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Integer_avm_sum = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Integer_avm_max = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Integer_avm_min = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int ByteArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 762; // byte code cost - 362; invoked methods cost - 400;
public static final int ByteArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int Double_avm_toHexString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Double_avm_toString = RT_METHOD_FEE_LEVEL_4; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Double_avm_valueOf = RT_METHOD_FEE_LEVEL_4; // totalCost - 492;
public static final int Double_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 432;
public static final int Double_avm_parseDouble = RT_METHOD_FEE_LEVEL_4; // totalCost - 604; // byte code cost - 176; invoked methods cost - 428;
public static final int Double_avm_isNaN = RT_METHOD_FEE_LEVEL_1; // totalCost - 161; //maximum cost of multiple blocks;
public static final int Double_avm_isInfinite = RT_METHOD_FEE_LEVEL_1; // totalCost - 218; //maximum cost of multiple blocks;
public static final int Double_avm_isFinite = RT_METHOD_FEE_LEVEL_1; // totalCost - 221; //maximum cost of multiple blocks;
public static final int Double_avm_isNaN_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Double_avm_isInfinite_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Double_avm_toString_1 = RT_METHOD_FEE_LEVEL_4; // totalCost - 122;
public static final int Double_avm_byteValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 66;
public static final int Double_avm_shortValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 66;
public static final int Double_avm_intValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Double_avm_longValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Double_avm_floatValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Double_avm_doubleValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Double_avm_hashCode = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Double_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Double_avm_doubleToLongBits = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Double_avm_longBitsToDouble = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Double_avm_compareTo = RT_METHOD_FEE_LEVEL_3; // totalCost - 134;
public static final int Double_avm_compare = RT_METHOD_FEE_LEVEL_3; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int Double_avm_sum = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Double_avm_max = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Double_avm_min = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Double_avm_equals = RT_METHOD_FEE_LEVEL_3;
public static final int FloatArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 562; // byte code cost - 362; invoked methods cost - 200;
public static final int FloatArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int DoubleArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 562; // byte code cost - 362; invoked methods cost - 200;
public static final int DoubleArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int Address_avm_constructor = RT_METHOD_FEE_LEVEL_2; // totalCost - 687; //maximum cost of multiple blocks; // byte code cost - 457; invoked methods cost - 230;
public static final int Address_avm_unwrap = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Address_avm_hashCode = RT_METHOD_FEE_LEVEL_2; // totalCost - 447; //maximum cost of multiple blocks; // byte code cost - 319; invoked methods cost - 128;
public static final int Address_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 1606; //maximum cost of multiple blocks; // byte code cost - 1090; invoked methods cost - 516;
public static final int Address_avm_toString = RT_METHOD_FEE_LEVEL_3; // totalCost - 1116; // byte code cost - 182; invoked methods cost - 934;
public static final int Address_avm_fromString = RT_METHOD_FEE_LEVEL_3;
public static final int Float_avm_toString = RT_METHOD_FEE_LEVEL_4; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Float_avm_toHexString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Float_avm_valueOf = RT_METHOD_FEE_LEVEL_4; // totalCost - 420;
public static final int Float_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 360;
public static final int Float_avm_parseFloat = RT_METHOD_FEE_LEVEL_4; // totalCost - 604; // byte code cost - 176; invoked methods cost - 428;
public static final int Float_avm_isNaN = RT_METHOD_FEE_LEVEL_1; // totalCost - 161; //maximum cost of multiple blocks;
public static final int Float_avm_isInfinite = RT_METHOD_FEE_LEVEL_1; // totalCost - 218; //maximum cost of multiple blocks;
public static final int Float_avm_isFinite = RT_METHOD_FEE_LEVEL_1; // totalCost - 221; //maximum cost of multiple blocks;
public static final int Float_avm_isNaN_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Float_avm_isInfinite_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Float_avm_toString_1 = RT_METHOD_FEE_LEVEL_4; // totalCost - 122;
public static final int Float_avm_byteValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 66;
public static final int Float_avm_shortValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 66;
public static final int Float_avm_intValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Float_avm_longValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Float_avm_floatValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Float_avm_doubleValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Float_avm_hashCode = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Float_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int Float_avm_equals = RT_METHOD_FEE_LEVEL_3; // totalCost - 343; //maximum cost of multiple blocks;
public static final int Float_avm_floatToIntBits = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Float_avm_intBitsToFloat = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Float_avm_compareTo = RT_METHOD_FEE_LEVEL_3; // totalCost - 134;
public static final int Float_avm_compare = RT_METHOD_FEE_LEVEL_3; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int Float_avm_sum = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Float_avm_max = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Float_avm_min = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int BigDecimal_avm_equals = RT_METHOD_FEE_LEVEL_3;
public static final int BigDecimal_avm_constructor_4 = RT_METHOD_FEE_LEVEL_3; // totalCost - 651; // byte code cost - 423; invoked methods cost - 228;
public static final int BigDecimal_avm_constructor_5 = RT_METHOD_FEE_LEVEL_3; // totalCost - 779; // byte code cost - 489; invoked methods cost - 290;
public static final int BigDecimal_avm_constructor_6 = RT_METHOD_FEE_LEVEL_3; // totalCost - 463; // byte code cost - 363; invoked methods cost - 100;
public static final int BigDecimal_avm_constructor_7 = RT_METHOD_FEE_LEVEL_3; // totalCost - 591; // byte code cost - 429; invoked methods cost - 162;
public static final int BigDecimal_avm_constructor_12 = RT_METHOD_FEE_LEVEL_3; // totalCost - 463; // byte code cost - 363; invoked methods cost - 100;
public static final int BigDecimal_avm_constructor_13 = RT_METHOD_FEE_LEVEL_3; // totalCost - 591; // byte code cost - 429; invoked methods cost - 162;
public static final int BigDecimal_avm_constructor_14 = RT_METHOD_FEE_LEVEL_3; // totalCost - 463; // byte code cost - 363; invoked methods cost - 100;
public static final int BigDecimal_avm_constructor_15 = RT_METHOD_FEE_LEVEL_3; // totalCost - 591; // byte code cost - 429; invoked methods cost - 162;
public static final int BigDecimal_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 448; // byte code cost - 348; invoked methods cost - 100;
public static final int BigDecimal_avm_valueOf_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 448; // byte code cost - 348; invoked methods cost - 100;
public static final int BigDecimal_avm_compareTo = RT_METHOD_FEE_LEVEL_3; // totalCost - 334; // byte code cost - 134; invoked methods cost - 200;
public static final int BigDecimal_avm_hashCode = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int BigDecimal_avm_toString = RT_METHOD_FEE_LEVEL_3; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int BigDecimal_avm_toPlainString = RT_METHOD_FEE_LEVEL_3; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int BigDecimal_avm_toBigInteger = RT_METHOD_FEE_LEVEL_4; // totalCost - 1378; // byte code cost - 378; invoked methods cost - 1000;
public static final int BigDecimal_avm_toBigIntegerExact = RT_METHOD_FEE_LEVEL_4; // totalCost - 1378; // byte code cost - 378; invoked methods cost - 1000;
public static final int BigDecimal_avm_longValue = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int BigDecimal_avm_longValueExact = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int BigDecimal_avm_intValue = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int BigDecimal_avm_intValueExact = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int BigDecimal_avm_shortValueExact = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int BigDecimal_avm_byteValueExact = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int BigDecimal_avm_floatValue = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int BigDecimal_avm_doubleValue = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int ObjectArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 562; // byte code cost - 362; invoked methods cost - 200;
public static final int ObjectArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int ObjectArray_avm_constructor_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 300; //maximum cost of multiple blocks;
public static final int IntArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 562; // byte code cost - 362; invoked methods cost - 200;
public static final int IntArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int String_avm_constructor = RT_METHOD_FEE_LEVEL_2; // totalCost - 397; // byte code cost - 297; invoked methods cost - 100;
public static final int String_avm_constructor_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 579; // byte code cost - 351; invoked methods cost - 228;
public static final int String_avm_constructor_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 591; // byte code cost - 363; invoked methods cost - 228;
public static final int String_avm_constructor_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 591; // byte code cost - 363; invoked methods cost - 228;
public static final int String_avm_constructor_7 = RT_METHOD_FEE_LEVEL_2; // totalCost - 579; // byte code cost - 351; invoked methods cost - 228;
public static final int String_avm_constructor_8 = RT_METHOD_FEE_LEVEL_2; // totalCost - 513; // byte code cost - 351; invoked methods cost - 162;
public static final int String_avm_constructor_9 = RT_METHOD_FEE_LEVEL_2; // totalCost - 513; // byte code cost - 351; invoked methods cost - 162;
public static final int String_avm_length = RT_METHOD_FEE_LEVEL_1; // totalCost - 288; // byte code cost - 188; invoked methods cost - 100;
public static final int String_avm_isEmpty = RT_METHOD_FEE_LEVEL_1; // totalCost - 288; // byte code cost - 188; invoked methods cost - 100;
public static final int String_avm_charAt = RT_METHOD_FEE_LEVEL_2; // totalCost - 294; // byte code cost - 194; invoked methods cost - 100;
public static final int String_avm_getChars = RT_METHOD_FEE_LEVEL_2; // totalCost - 500; // byte code cost - 272; invoked methods cost - 228;
public static final int String_avm_getBytes_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 448; // byte code cost - 348; invoked methods cost - 100;
public static final int String_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 483; //maximum cost of multiple blocks; // byte code cost - 383; invoked methods cost - 100;
public static final int String_avm_contentEquals = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 254; invoked methods cost - 162;
public static final int String_avm_contentEquals_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 542; // byte code cost - 314; invoked methods cost - 228;
public static final int String_avm_equalsIgnoreCase = RT_METHOD_FEE_LEVEL_3; // totalCost - 300; // byte code cost - 200; invoked methods cost - 100;
public static final int String_avm_compareTo = RT_METHOD_FEE_LEVEL_2; // totalCost - 482; // byte code cost - 254; invoked methods cost - 228;
public static final int String_avm_compareToIgnoreCase = RT_METHOD_FEE_LEVEL_3; // totalCost - 300; // byte code cost - 200; invoked methods cost - 100;
public static final int String_avm_regionMatches = RT_METHOD_FEE_LEVEL_2; // totalCost - 318; // byte code cost - 218; invoked methods cost - 100;
public static final int String_avm_regionMatches_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 324; // byte code cost - 224; invoked methods cost - 100;
public static final int String_avm_startsWith = RT_METHOD_FEE_LEVEL_2; // totalCost - 306; // byte code cost - 206; invoked methods cost - 100;
public static final int String_avm_startsWith_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 300; // byte code cost - 200; invoked methods cost - 100;
public static final int String_avm_endsWith = RT_METHOD_FEE_LEVEL_2; // totalCost - 300; // byte code cost - 200; invoked methods cost - 100;
public static final int String_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 288; // byte code cost - 188; invoked methods cost - 100;
public static final int String_avm_indexOf = RT_METHOD_FEE_LEVEL_2; // totalCost - 294; // byte code cost - 194; invoked methods cost - 100;
public static final int String_avm_indexOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 300; // byte code cost - 200; invoked methods cost - 100;
public static final int String_avm_lastIndexOf = RT_METHOD_FEE_LEVEL_2; // totalCost - 294; // byte code cost - 194; invoked methods cost - 100;
public static final int String_avm_lastIndexOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 300; // byte code cost - 200; invoked methods cost - 100;
public static final int String_avm_indexOf_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 366; // byte code cost - 266; invoked methods cost - 100;
public static final int String_avm_lastIndexOf_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 366; // byte code cost - 266; invoked methods cost - 100;
public static final int String_avm_lastIndexOf_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 372; // byte code cost - 272; invoked methods cost - 100;
public static final int String_avm_substring = RT_METHOD_FEE_LEVEL_2; // totalCost - 454; // byte code cost - 354; invoked methods cost - 100;
public static final int String_avm_substring_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 460; // byte code cost - 360; invoked methods cost - 100;
public static final int String_avm_subSequence = RT_METHOD_FEE_LEVEL_2; // totalCost - 194;
public static final int String_avm_concat = RT_METHOD_FEE_LEVEL_2; // totalCost - 526; // byte code cost - 426; invoked methods cost - 100;
public static final int String_avm_replace = RT_METHOD_FEE_LEVEL_3; // totalCost - 460; // byte code cost - 360; invoked methods cost - 100;
public static final int String_avm_contains = RT_METHOD_FEE_LEVEL_2; // totalCost - 412; //maximum cost of multiple blocks;
public static final int String_avm_replace_1 = RT_METHOD_FEE_LEVEL_4; // totalCost - 1092; // byte code cost - 736; invoked methods cost - 356;
public static final int String_avm_toLowerCase = RT_METHOD_FEE_LEVEL_3; // totalCost - 448; // byte code cost - 348; invoked methods cost - 100;
public static final int String_avm_toUpperCase = RT_METHOD_FEE_LEVEL_3; // totalCost - 448; // byte code cost - 348; invoked methods cost - 100;
public static final int String_avm_trim = RT_METHOD_FEE_LEVEL_2; // totalCost - 448; // byte code cost - 348; invoked methods cost - 100;
public static final int String_avm_toString = RT_METHOD_FEE_LEVEL_1; // totalCost - 56;
public static final int String_avm_toCharArray = RT_METHOD_FEE_LEVEL_2; // totalCost - 448; // byte code cost - 348; invoked methods cost - 100;
public static final int String_avm_valueOf = RT_METHOD_FEE_LEVEL_3; // totalCost - 442; // byte code cost - 342; invoked methods cost - 100;
public static final int String_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 630; // byte code cost - 402; invoked methods cost - 228;
public static final int String_avm_valueOf_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 642; // byte code cost - 414; invoked methods cost - 228;
public static final int String_avm_copyValueOf = RT_METHOD_FEE_LEVEL_2; // totalCost - 642; // byte code cost - 414; invoked methods cost - 228;
public static final int String_avm_copyValueOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 630; // byte code cost - 402; invoked methods cost - 228;
public static final int String_avm_valueOf_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 376; // byte code cost - 276; invoked methods cost - 100;
public static final int String_avm_valueOf_4 = RT_METHOD_FEE_LEVEL_2; // totalCost - 376; // byte code cost - 276; invoked methods cost - 100;
public static final int String_avm_valueOf_5 = RT_METHOD_FEE_LEVEL_2; // totalCost - 376; // byte code cost - 276; invoked methods cost - 100;
public static final int String_avm_valueOf_6 = RT_METHOD_FEE_LEVEL_2; // totalCost - 376; // byte code cost - 276; invoked methods cost - 100;
public static final int String_avm_valueOf_7 = RT_METHOD_FEE_LEVEL_4; // totalCost - 376; // byte code cost - 276; invoked methods cost - 100;
public static final int String_avm_valueOf_8 = RT_METHOD_FEE_LEVEL_4; // totalCost - 376; // byte code cost - 276; invoked methods cost - 100;
public static final int String_avm_constructor_10 = RT_METHOD_FEE_LEVEL_2; // totalCost - 331; // byte code cost - 131; invoked methods cost - 200;
public static final int BlockchainRuntime_avm_getTransactionHash = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getTransactionIndex = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getTransactionTimestamp = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getTransactionNonce = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getAddress = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getCaller = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getOrigin = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getOwner = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getBlockTimestamp = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getBlockHeight = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_getBalance = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int BlockchainRuntime_avm_revert = RT_METHOD_FEE_LEVEL_1; // totalCost - 116;
public static final int BlockchainRuntime_avm_require = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_println = RT_METHOD_FEE_LEVEL_1; // totalCost - 696; // byte code cost - 122; invoked methods cost - 574;
public static final int BlockchainRuntime_avm_hash_base = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_hash_per_bytes = 50;
public static final int BlockchainRuntime_avm_verifySignature = RT_METHOD_FEE_LEVEL_6;
public static final int BlockchainRuntime_avm_verifySignature_per_bytes = 50;
public static final int BlockchainRuntime_avm_recoverKey = RT_METHOD_FEE_LEVEL_6;
public static final int BlockchainRuntime_avm_recoverKey_per_bytes = 50;
public static final int BlockchainRuntime_avm_getAddressFromKey = RT_METHOD_FEE_LEVEL_5;
public static final int BlockchainRuntime_avm_getFeeSharingProportion = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_setFeeSharingProportion = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_newDictDB = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_newArrayDB = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_newVarDB = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_newByteArrayObjectReader = RT_METHOD_FEE_LEVEL_1;
public static final int BlockchainRuntime_avm_newByteArrayObjectWriter = RT_METHOD_FEE_LEVEL_1;
public static final int DictDB_avm_at = RT_METHOD_FEE_LEVEL_1;
public static final int ShortArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 462; // byte code cost - 362; invoked methods cost - 100;
public static final int ShortArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int Object_avm_getClass = RT_METHOD_FEE_LEVEL_2; // totalCost - 344; // byte code cost - 244; invoked methods cost - 100;
public static final int Object_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 128;
public static final int Object_avm_equals = RT_METHOD_FEE_LEVEL_1; // totalCost - 159; //maximum cost of multiple blocks;
public static final int Object_avm_clone = RT_METHOD_FEE_LEVEL_2; // totalCost - 263; // byte code cost - 163; invoked methods cost - 100;
public static final int Object_avm_toString = RT_METHOD_FEE_LEVEL_3; // totalCost - 56; // this was increased due to multiple operations being done for getting the class name
public static final int MathContext_avm_constructor = RT_METHOD_FEE_LEVEL_3; // totalCost - 487; // byte code cost - 387; invoked methods cost - 100;
public static final int MathContext_avm_constructor_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 615; // byte code cost - 453; invoked methods cost - 162;
public static final int MathContext_avm_constructor_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 675; // byte code cost - 447; invoked methods cost - 228;
public static final int MathContext_avm_getPrecision = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int MathContext_avm_getRoundingMode = RT_METHOD_FEE_LEVEL_4; // totalCost - 602; // byte code cost - 402; invoked methods cost - 200;
public static final int MathContext_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 351; //maximum cost of multiple blocks; // byte code cost - 251; invoked methods cost - 100;
public static final int MathContext_avm_hashCode = RT_METHOD_FEE_LEVEL_4; // totalCost - 973; // byte code cost - 557; invoked methods cost - 416;
public static final int MathContext_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 382; // byte code cost - 282; invoked methods cost - 100;
public static final int RoundingMode_avm_values = RT_METHOD_FEE_LEVEL_3; // totalCost - 418; // byte code cost - 118; invoked methods cost - 300;
public static final int RoundingMode_avm_valueOf = RT_METHOD_FEE_LEVEL_4; // totalCost - 284;
public static final int RoundingMode_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 656; //maximum cost of multiple blocks; // byte code cost - 396; invoked methods cost - 260;
public static final int Boolean_avm_parseBoolean = RT_METHOD_FEE_LEVEL_3; // totalCost - 548; //maximum cost of multiple blocks; // byte code cost - 320; invoked methods cost - 228;
public static final int Boolean_avm_booleanValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Boolean_avm_valueOf = RT_METHOD_FEE_LEVEL_1; // totalCost - 152; //maximum cost of multiple blocks;
public static final int Boolean_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 212; //maximum cost of multiple blocks;
public static final int Boolean_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 472; //maximum cost of multiple blocks;
public static final int Boolean_avm_toString_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 478; //maximum cost of multiple blocks;
public static final int Boolean_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Boolean_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 152; //maximum cost of multiple blocks;
public static final int Boolean_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 333; //maximum cost of multiple blocks;
public static final int Boolean_avm_compareTo = RT_METHOD_FEE_LEVEL_1; // totalCost - 134;
public static final int Boolean_avm_compare = RT_METHOD_FEE_LEVEL_1; // totalCost - 255; //maximum cost of multiple blocks;
public static final int Boolean_avm_logicalAnd = RT_METHOD_FEE_LEVEL_1; // totalCost - 200; //maximum cost of multiple blocks;
public static final int Boolean_avm_logicalOr = RT_METHOD_FEE_LEVEL_1; // totalCost - 200; //maximum cost of multiple blocks;
public static final int Boolean_avm_logicalXor = RT_METHOD_FEE_LEVEL_1; // totalCost - 165; // byte code cost - 65; invoked methods cost - 100;
public static final int Character_avm_valueOf = RT_METHOD_FEE_LEVEL_1; // totalCost - 534;
public static final int Character_avm_charValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 128;
public static final int Character_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 188;
public static final int Character_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 56;
public static final int Character_avm_equals = RT_METHOD_FEE_LEVEL_2;
public static final int Character_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 452;
public static final int Character_avm_toString_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 376; // byte code cost - 276; invoked methods cost - 100;
public static final int Character_avm_isLowerCase = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_isUpperCase = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_isDigit = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_isLetter = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_isLetterOrDigit = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_toLowerCase = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_toUpperCase = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_digit = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int Character_avm_getNumericValue = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_isSpaceChar = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_isWhitespace = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Character_avm_forDigit = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int Character_avm_compareTo = RT_METHOD_FEE_LEVEL_1; // totalCost - 134;
public static final int Character_avm_compare = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Class_avm_getName = RT_METHOD_FEE_LEVEL_2; // totalCost - 382; // byte code cost - 282; invoked methods cost - 100;
public static final int Class_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 56;
public static final int Class_avm_cast = RT_METHOD_FEE_LEVEL_2; // totalCost - 230; // byte code cost - 130; invoked methods cost - 100;
public static final int Class_avm_getSuperclass = RT_METHOD_FEE_LEVEL_3; // totalCost - 429; //maximum cost of multiple blocks; // byte code cost - 329; invoked methods cost - 100;
public static final int Class_avm_desiredAssertionStatus = RT_METHOD_FEE_LEVEL_1; // totalCost - 1997; // byte code cost - 56; invoked methods cost - 1941;
public static final int StringBuilder_avm_constructor = RT_METHOD_FEE_LEVEL_2; // totalCost - 391; // byte code cost - 291; invoked methods cost - 100;
public static final int StringBuilder_avm_constructor_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 579; // byte code cost - 351; invoked methods cost - 228;
public static final int StringBuilder_avm_constructor_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 639; // byte code cost - 411; invoked methods cost - 228;
public static final int StringBuilder_avm_constructor_3 = RT_METHOD_FEE_LEVEL_3;
public static final int StringBuilder_avm_append = RT_METHOD_FEE_LEVEL_2;
public static final int StringBuilder_avm_append_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 424; // byte code cost - 196; invoked methods cost - 228;
public static final int StringBuilder_avm_append_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 358; // byte code cost - 196; invoked methods cost - 162;
public static final int StringBuilder_avm_append_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 424; // byte code cost - 196; invoked methods cost - 228;
public static final int StringBuilder_avm_append_4 = RT_METHOD_FEE_LEVEL_2; // totalCost - 436; // byte code cost - 208; invoked methods cost - 228;
public static final int StringBuilder_avm_append_5 = RT_METHOD_FEE_LEVEL_2; // totalCost - 296; // byte code cost - 196; invoked methods cost - 100;
public static final int StringBuilder_avm_append_6 = RT_METHOD_FEE_LEVEL_2; // totalCost - 496; // byte code cost - 268; invoked methods cost - 228;
public static final int StringBuilder_avm_append_7 = RT_METHOD_FEE_LEVEL_2; // totalCost - 236; // byte code cost - 136; invoked methods cost - 100;
public static final int StringBuilder_avm_append_8 = RT_METHOD_FEE_LEVEL_2; // totalCost - 236; // byte code cost - 136; invoked methods cost - 100;
public static final int StringBuilder_avm_append_9 = RT_METHOD_FEE_LEVEL_2; // totalCost - 236; // byte code cost - 136; invoked methods cost - 100;
public static final int StringBuilder_avm_append_10 = RT_METHOD_FEE_LEVEL_2; // totalCost - 236; // byte code cost - 136; invoked methods cost - 100;
public static final int StringBuilder_avm_append_11 = RT_METHOD_FEE_LEVEL_2; // totalCost - 236; // byte code cost - 136; invoked methods cost - 100;
public static final int StringBuilder_avm_append_12 = RT_METHOD_FEE_LEVEL_2; // totalCost - 236; // byte code cost - 136; invoked methods cost - 100;
public static final int StringBuilder_avm_delete = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuilder_avm_deleteCharAt = RT_METHOD_FEE_LEVEL_2; // totalCost - 236; // byte code cost - 136; invoked methods cost - 100;
public static final int StringBuilder_avm_replace = RT_METHOD_FEE_LEVEL_2; // totalCost - 443; // byte code cost - 215; invoked methods cost - 228;
public static final int StringBuilder_avm_insert = RT_METHOD_FEE_LEVEL_2; // totalCost - 442; // byte code cost - 214; invoked methods cost - 228;
public static final int StringBuilder_avm_insert_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 430; // byte code cost - 202; invoked methods cost - 228;
public static final int StringBuilder_avm_insert_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 430; // byte code cost - 202; invoked methods cost - 228;
public static final int StringBuilder_avm_insert_4 = RT_METHOD_FEE_LEVEL_2; // totalCost - 490; // byte code cost - 262; invoked methods cost - 228;
public static final int StringBuilder_avm_insert_5 = RT_METHOD_FEE_LEVEL_2; // totalCost - 562; // byte code cost - 334; invoked methods cost - 228;
public static final int StringBuilder_avm_insert_6 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuilder_avm_insert_7 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuilder_avm_insert_8 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuilder_avm_insert_9 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuilder_avm_insert_10 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuilder_avm_insert_11 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuilder_avm_indexOf = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 188; invoked methods cost - 228;
public static final int StringBuilder_avm_indexOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 194; invoked methods cost - 228;
public static final int StringBuilder_avm_lastIndexOf = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 188; invoked methods cost - 228;
public static final int StringBuilder_avm_lastIndexOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 194; invoked methods cost - 228;
public static final int StringBuilder_avm_reverse = RT_METHOD_FEE_LEVEL_2; // totalCost - 230; // byte code cost - 130; invoked methods cost - 100;
public static final int StringBuilder_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 216;
public static final int StringBuilder_avm_charAt = RT_METHOD_FEE_LEVEL_2; // totalCost - 228; // byte code cost - 128; invoked methods cost - 100;
public static final int StringBuilder_avm_subSequence = RT_METHOD_FEE_LEVEL_2; // totalCost - 188;
public static final int StringBuilder_avm_length = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int StringBuilder_avm_setLength = RT_METHOD_FEE_LEVEL_2;
public static final int System_avm_arraycopy = RT_METHOD_FEE_LEVEL_1; // totalCost - 819; //maximum cost of multiple blocks; // byte code cost - 619; invoked methods cost - 200;
public static final int StringBuffer_avm_constructor = RT_METHOD_FEE_LEVEL_2; // totalCost - 391; // byte code cost - 291; invoked methods cost - 100;
public static final int StringBuffer_avm_constructor_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 265;
public static final int StringBuffer_avm_constructor_3 = RT_METHOD_FEE_LEVEL_3; // totalCost - 265;
public static final int StringBuffer_avm_length = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StringBuffer_avm_trimToSize = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StringBuffer_avm_setLength = RT_METHOD_FEE_LEVEL_2; // totalCost - 228; // byte code cost - 128; invoked methods cost - 100;
public static final int StringBuffer_avm_charAt = RT_METHOD_FEE_LEVEL_2; // totalCost - 228; // byte code cost - 128; invoked methods cost - 100;
public static final int StringBuffer_avm_getChars = RT_METHOD_FEE_LEVEL_2; // totalCost - 246; // byte code cost - 146; invoked methods cost - 100;
public static final int StringBuffer_avm_setCharAt = RT_METHOD_FEE_LEVEL_2; // totalCost - 234; // byte code cost - 134; invoked methods cost - 100;
public static final int StringBuffer_avm_append = RT_METHOD_FEE_LEVEL_2;
public static final int StringBuffer_avm_append_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_append_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 249; // byte code cost - 149; invoked methods cost - 100;
public static final int StringBuffer_avm_append_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 303; // byte code cost - 203; invoked methods cost - 100;
public static final int StringBuffer_avm_append_4 = RT_METHOD_FEE_LEVEL_2; // totalCost - 503; // byte code cost - 275; invoked methods cost - 228;
public static final int StringBuffer_avm_append_5 = RT_METHOD_FEE_LEVEL_2; // totalCost - 431; // byte code cost - 203; invoked methods cost - 228;
public static final int StringBuffer_avm_append_6 = RT_METHOD_FEE_LEVEL_2; // totalCost - 443; // byte code cost - 215; invoked methods cost - 228;
public static final int StringBuffer_avm_append_7 = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_append_8 = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_append_9 = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_append_10 = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_append_11 = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_append_12 = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_delete = RT_METHOD_FEE_LEVEL_2; // totalCost - 249; // byte code cost - 149; invoked methods cost - 100;
public static final int StringBuffer_avm_deleteCharAt = RT_METHOD_FEE_LEVEL_2; // totalCost - 243; // byte code cost - 143; invoked methods cost - 100;
public static final int StringBuffer_avm_replace = RT_METHOD_FEE_LEVEL_2; // totalCost - 443; // byte code cost - 215; invoked methods cost - 228;
public static final int StringBuffer_avm_substring = RT_METHOD_FEE_LEVEL_2; // totalCost - 388; // byte code cost - 288; invoked methods cost - 100;
public static final int StringBuffer_avm_subSequence = RT_METHOD_FEE_LEVEL_2; // totalCost - 554; // byte code cost - 354; invoked methods cost - 200;
public static final int StringBuffer_avm_substring_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 394; // byte code cost - 294; invoked methods cost - 100;
public static final int StringBuffer_avm_insert = RT_METHOD_FEE_LEVEL_2; // totalCost - 442; // byte code cost - 214; invoked methods cost - 228;
public static final int StringBuffer_avm_insert_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 430; // byte code cost - 202; invoked methods cost - 228;
public static final int StringBuffer_avm_insert_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 430; // byte code cost - 202; invoked methods cost - 228;
public static final int StringBuffer_avm_insert_4 = RT_METHOD_FEE_LEVEL_2; // totalCost - 302; // byte code cost - 202; invoked methods cost - 100;
public static final int StringBuffer_avm_insert_5 = RT_METHOD_FEE_LEVEL_2; // totalCost - 314; // byte code cost - 214; invoked methods cost - 100;
public static final int StringBuffer_avm_insert_6 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuffer_avm_insert_7 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuffer_avm_insert_8 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuffer_avm_insert_9 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuffer_avm_insert_10 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuffer_avm_insert_11 = RT_METHOD_FEE_LEVEL_2; // totalCost - 242; // byte code cost - 142; invoked methods cost - 100;
public static final int StringBuffer_avm_indexOf = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 188; invoked methods cost - 228;
public static final int StringBuffer_avm_indexOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 194; invoked methods cost - 228;
public static final int StringBuffer_avm_lastIndexOf = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 188; invoked methods cost - 228;
public static final int StringBuffer_avm_lastIndexOf_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 422; // byte code cost - 194; invoked methods cost - 228;
public static final int StringBuffer_avm_reverse = RT_METHOD_FEE_LEVEL_2; // totalCost - 230; // byte code cost - 130; invoked methods cost - 100;
public static final int StringBuffer_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 216;
public static final int Arrays_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 508; //maximum cost of multiple blocks; // byte code cost - 280; invoked methods cost - 228;
public static final int Arrays_avm_equals = RT_METHOD_FEE_LEVEL_1; // totalCost - 861; //maximum cost of multiple blocks; // byte code cost - 505; invoked methods cost - 356;
public static final int Arrays_avm_copyOfRange = RT_METHOD_FEE_LEVEL_1; // totalCost - 576; // byte code cost - 348; invoked methods cost - 228;
public static final int Arrays_avm_fill = RT_METHOD_FEE_LEVEL_1; // totalCost - 422; // byte code cost - 194; invoked methods cost - 228;
public static final int StrictMath_avm_sin = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_cos = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_tan = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_asin = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_acos = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_atan = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_toRadians = RT_METHOD_FEE_LEVEL_1; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_toDegrees = RT_METHOD_FEE_LEVEL_1; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_exp = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_log = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_log10 = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_sqrt = RT_METHOD_FEE_LEVEL_4; // totalCost - 1616; // byte code cost - 116; invoked methods cost - 1500;
public static final int StrictMath_avm_cbrt = RT_METHOD_FEE_LEVEL_4; // totalCost - 1616; // byte code cost - 116; invoked methods cost - 1500;
public static final int StrictMath_avm_IEEEremainder = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_ceil = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_floor = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_rint = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_atan2 = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_pow = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_round = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_round_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_addExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_addExact_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_subtractExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_subtractExact_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_multiplyExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_multiplyExact_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_multiplyExact_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_toIntExact = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_multiplyFull = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_multiplyHigh = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_floorDiv = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_floorDiv_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_floorDiv_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_floorMod = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_floorMod_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_floorMod_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_abs = RT_METHOD_FEE_LEVEL_1; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_abs_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_abs_2 = RT_METHOD_FEE_LEVEL_1; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_abs_3 = RT_METHOD_FEE_LEVEL_1; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int StrictMath_avm_max = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_max_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_max_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_max_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_min = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_min_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_min_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_min_3 = RT_METHOD_FEE_LEVEL_2; // totalCost - 222; // byte code cost - 122; invoked methods cost - 100;
public static final int StrictMath_avm_fma = RT_METHOD_FEE_LEVEL_2; // totalCost - 128;
public static final int StrictMath_avm_fma_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 128;
public static final int StrictMath_avm_ulp = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_ulp_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_signum = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_signum_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_sinh = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_cosh = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_tanh = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_hypot = RT_METHOD_FEE_LEVEL_4; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int StrictMath_avm_expm1 = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_log1p = RT_METHOD_FEE_LEVEL_4; // totalCost - 1116; // byte code cost - 116; invoked methods cost - 1000;
public static final int StrictMath_avm_copySign = RT_METHOD_FEE_LEVEL_2; // totalCost - 278; //maximum cost of multiple blocks;
public static final int StrictMath_avm_copySign_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 278; //maximum cost of multiple blocks;
public static final int StrictMath_avm_getExponent = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_getExponent_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_nextAfter = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int StrictMath_avm_nextAfter_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int StrictMath_avm_nextUp = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_nextUp_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_nextDown = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_nextDown_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 116;
public static final int StrictMath_avm_scalb = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int StrictMath_avm_scalb_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Enum_avm_name = RT_METHOD_FEE_LEVEL_1; // totalCost - 128;
public static final int Enum_avm_ordinal = RT_METHOD_FEE_LEVEL_1; // totalCost - 128;
public static final int Enum_avm_constructor = RT_METHOD_FEE_LEVEL_2; // totalCost - 146;
public static final int Enum_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 128;
public static final int Enum_avm_equals = RT_METHOD_FEE_LEVEL_1; // totalCost - 225; //maximum cost of multiple blocks;
public static final int Enum_avm_clone = RT_METHOD_FEE_LEVEL_2; // totalCost - 263; // byte code cost - 163; invoked methods cost - 100;
public static final int Enum_avm_valueOf = RT_METHOD_FEE_LEVEL_4; // totalCost - 3516; //maximum cost of multiple blocks; // byte code cost - 753; invoked methods cost - 2763;
public static final int Byte_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Byte_avm_valueOf = RT_METHOD_FEE_LEVEL_1; // totalCost - 270;
public static final int Byte_avm_parseByte = RT_METHOD_FEE_LEVEL_3; // totalCost - 410; // byte code cost - 182; invoked methods cost - 228;
public static final int Byte_avm_parseByte_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 122;
public static final int Byte_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 182;
public static final int Byte_avm_valueOf_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 122;
public static final int Byte_avm_decode = RT_METHOD_FEE_LEVEL_3; // totalCost - 778; // byte code cost - 450; invoked methods cost - 328;
public static final int Byte_avm_byteValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Byte_avm_shortValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Byte_avm_intValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Byte_avm_longValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Byte_avm_floatValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Byte_avm_doubleValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Byte_avm_toString_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Byte_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Byte_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 56;
public static final int Byte_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 333; //maximum cost of multiple blocks;
public static final int Byte_avm_compareTo = RT_METHOD_FEE_LEVEL_1; // totalCost - 134;
public static final int Byte_avm_compare = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Byte_avm_compareUnsigned = RT_METHOD_FEE_LEVEL_1; // totalCost - 185;
public static final int Byte_avm_toUnsignedInt = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Byte_avm_toUnsignedLong = RT_METHOD_FEE_LEVEL_1; // totalCost - 67;
public static final int CharArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 562; // byte code cost - 362; invoked methods cost - 200;
public static final int CharArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int LongArray_avm_clone = RT_METHOD_FEE_LEVEL_3; // totalCost - 562; // byte code cost - 362; invoked methods cost - 200;
public static final int LongArray_avm_constructor = RT_METHOD_FEE_LEVEL_1; // totalCost - 176;
public static final int Long_avm_toString = RT_METHOD_FEE_LEVEL_4; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int Long_avm_toUnsignedString = RT_METHOD_FEE_LEVEL_3; // totalCost - 582; // byte code cost - 282; invoked methods cost - 300;
public static final int Long_avm_toHexString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Long_avm_toOctalString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Long_avm_toBinaryString = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Long_avm_toString_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Long_avm_toUnsignedString_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 576; // byte code cost - 276; invoked methods cost - 300;
public static final int Long_avm_parseLong = RT_METHOD_FEE_LEVEL_3; // totalCost - 610; // byte code cost - 182; invoked methods cost - 428;
public static final int Long_avm_parseLong_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 610; // byte code cost - 182; invoked methods cost - 428;
public static final int Long_avm_parseLong_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 682; // byte code cost - 254; invoked methods cost - 428;
public static final int Long_avm_parseUnsignedLong = RT_METHOD_FEE_LEVEL_3; // totalCost - 610; // byte code cost - 182; invoked methods cost - 428;
public static final int Long_avm_parseUnsignedLong_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 610; // byte code cost - 182; invoked methods cost - 428;
public static final int Long_avm_parseUnsignedLong_2 = RT_METHOD_FEE_LEVEL_3; // totalCost - 682; // byte code cost - 254; invoked methods cost - 428;
public static final int Long_avm_valueOf = RT_METHOD_FEE_LEVEL_3; // totalCost - 182;
public static final int Long_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 182;
public static final int Long_avm_valueOf_2 = RT_METHOD_FEE_LEVEL_1; // totalCost - 312;
public static final int Long_avm_decode = RT_METHOD_FEE_LEVEL_4; // totalCost - 1020; // byte code cost - 492; invoked methods cost - 528;
public static final int Long_avm_byteValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 66;
public static final int Long_avm_shortValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 66;
public static final int Long_avm_intValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Long_avm_longValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Long_avm_floatValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Long_avm_doubleValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Long_avm_toString_2 = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Long_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Long_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 76;
public static final int Long_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 335; //maximum cost of multiple blocks;
public static final int Long_avm_compareTo = RT_METHOD_FEE_LEVEL_1; // totalCost - 134;
public static final int Long_avm_compare = RT_METHOD_FEE_LEVEL_1; // totalCost - 266; //maximum cost of multiple blocks;
public static final int Long_avm_compareUnsigned = RT_METHOD_FEE_LEVEL_1; // totalCost - 140;
public static final int Long_avm_divideUnsigned = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int Long_avm_remainderUnsigned = RT_METHOD_FEE_LEVEL_2; // totalCost - 1122; // byte code cost - 122; invoked methods cost - 1000;
public static final int Long_avm_highestOneBit = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Long_avm_lowestOneBit = RT_METHOD_FEE_LEVEL_2; // totalCost - 216; // byte code cost - 116; invoked methods cost - 100;
public static final int Long_avm_numberOfLeadingZeros = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 116; invoked methods cost - 300;
public static final int Long_avm_numberOfTrailingZeros = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 116; invoked methods cost - 300;
public static final int Long_avm_bitCount = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 116; invoked methods cost - 300;
public static final int Long_avm_rotateLeft = RT_METHOD_FEE_LEVEL_1; // totalCost - 86;
public static final int Long_avm_rotateRight = RT_METHOD_FEE_LEVEL_1; // totalCost - 86;
public static final int Long_avm_reverse = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 116; invoked methods cost - 300;
public static final int Long_avm_signum = RT_METHOD_FEE_LEVEL_1; // totalCost - 88;
public static final int Long_avm_reverseBytes = RT_METHOD_FEE_LEVEL_2; // totalCost - 416; // byte code cost - 116; invoked methods cost - 300;
public static final int Long_avm_sum = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Long_avm_max = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Long_avm_min = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Short_avm_toString = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Short_avm_parseShort = RT_METHOD_FEE_LEVEL_3; // totalCost - 610; // byte code cost - 182; invoked methods cost - 428;
public static final int Short_avm_parseShort_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 122;
public static final int Short_avm_valueOf = RT_METHOD_FEE_LEVEL_3; // totalCost - 182;
public static final int Short_avm_valueOf_1 = RT_METHOD_FEE_LEVEL_3; // totalCost - 122;
public static final int Short_avm_valueOf_2 = RT_METHOD_FEE_LEVEL_1; // totalCost - 276;
public static final int Short_avm_decode = RT_METHOD_FEE_LEVEL_4; // totalCost - 984; // byte code cost - 456; invoked methods cost - 528;
public static final int Short_avm_byteValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Short_avm_shortValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Short_avm_intValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 62;
public static final int Short_avm_longValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Short_avm_floatValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Short_avm_doubleValue = RT_METHOD_FEE_LEVEL_1; // totalCost - 64;
public static final int Short_avm_toString_1 = RT_METHOD_FEE_LEVEL_2; // totalCost - 122;
public static final int Short_avm_hashCode = RT_METHOD_FEE_LEVEL_1; // totalCost - 122;
public static final int Short_avm_hashCode_1 = RT_METHOD_FEE_LEVEL_1; // totalCost - 56;
public static final int Short_avm_equals = RT_METHOD_FEE_LEVEL_2; // totalCost - 333; //maximum cost of multiple blocks;
public static final int Short_avm_compareTo = RT_METHOD_FEE_LEVEL_1; // totalCost - 134;
public static final int Short_avm_compare = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Short_avm_compareUnsigned = RT_METHOD_FEE_LEVEL_1; // totalCost - 185;
public static final int Short_avm_reverseBytes = RT_METHOD_FEE_LEVEL_1; // totalCost - 416; // byte code cost - 116; invoked methods cost - 300;
public static final int Short_avm_toUnsignedInt = RT_METHOD_FEE_LEVEL_1; // totalCost - 65;
public static final int Short_avm_toUnsignedLong = RT_METHOD_FEE_LEVEL_1; // totalCost - 67;
public static final int TimeUnit_avm_convert = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_toDays = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_toHours = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_toMinutes = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_toSeconds = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_toMillis = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_toMicros = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_toNanos = RT_METHOD_FEE_LEVEL_1;
public static final int TimeUnit_avm_values = RT_METHOD_FEE_LEVEL_3;
public static final int TimeUnit_avm_valueOf = RT_METHOD_FEE_LEVEL_4;
public static final int Throwable_Hierarchy_Base_Fee = RT_METHOD_FEE_LEVEL_1;
public static final int Throwable_avm_toString = RT_METHOD_FEE_LEVEL_2;
public static final int UnmodifiableArrayCollection_size = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayCollection_contains = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayCollection_toArray = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayCollection_containsAll = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayCollection_iterator = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayCollection_Iter_hasNext = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayCollection_Iter_next = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_constructor = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_equals = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_hashCode = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_get = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_indexOf = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_lastIndexOf = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_listIterator = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_subList = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_emptyList = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_ListIter_hasPrevious = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_ListIter_previous = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_ListIter_nextIndex = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayList_ListIter_previousIndex = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_constructor = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_size = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_containsKey = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_containsValue = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_get = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_keySet = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_values = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_entrySet = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_equals = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_hashCode = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArrayMap_emptyMap = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArraySet_constructor = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArraySet_equals = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableArraySet_hashCode = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableMapEntry_constructor = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableMapEntry_getKey = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableMapEntry_getValue = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableMapEntry_equals = RT_METHOD_FEE_LEVEL_1;
public static final int UnmodifiableMapEntry_hashCode = RT_METHOD_FEE_LEVEL_1;
public static final int ObjectWriter_writePricePerByte = 2;
public static final int ObjectWriter_customMethodBase = RT_METHOD_FEE_LEVEL_1;
public static final int ObjectWriter_beginBase = RT_METHOD_FEE_LEVEL_1;
public static final int ObjectWriter_endBase = RT_METHOD_FEE_LEVEL_1;
public static final int ObjectReader_readPricePerByte = 2;
public static final int ObjectReader_skipPricePerByte = 1;
public static final int ObjectReader_customMethodBase = RT_METHOD_FEE_LEVEL_1;
public static final int ObjectReader_beginBase = RT_METHOD_FEE_LEVEL_1;
public static final int ObjectReader_endBase = RT_METHOD_FEE_LEVEL_1;
public static final int ObjectReader_hasNext = RT_METHOD_FEE_LEVEL_1;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy