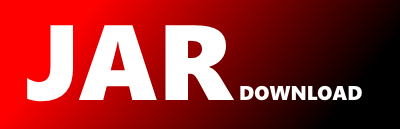
s.java.util.List Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javaee-rt Show documentation
Show all versions of javaee-rt Show documentation
An Execution Environment for Java SCOREs
package s.java.util;
import a.ObjectArray;
import pi.UnmodifiableArrayList;
import foundation.icon.ee.util.IObjects;
import i.IObject;
import i.IObjectArray;
import java.util.Objects;
public interface List extends Collection {
// Positional Access Operations
E avm_get(int index);
IObject avm_set(int index, E element);
void avm_add(int index, E element);
E avm_remove(int index);
int avm_indexOf(IObject o);
int avm_lastIndexOf(IObject o);
ListIterator avm_listIterator();
ListIterator avm_listIterator(int index);
// View
List avm_subList(int fromIndex, int toIndex);
static List avm_of() {
return UnmodifiableArrayList.emptyList();
}
static List avm_of(E o0) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0}));
}
static List avm_of(E o0, E o1) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1}));
}
static List avm_of(E o0, E o1, E o2) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2}));
}
static List avm_of(E o0, E o1, E o2, E o3) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2, o3}));
}
static List avm_of(E o0, E o1, E o2, E o3,
E o4) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2, o3, o4}));
}
static List avm_of(E o0, E o1, E o2, E o3,
E o4, E o5) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2, o3, o4, o5}));
}
static List avm_of(E o0, E o1, E o2, E o3,
E o4, E o5, E o6) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2, o3, o4, o5, o6}));
}
static List avm_of(E o0, E o1, E o2, E o3,
E o4, E o5, E o6, E o7) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2, o3, o4, o5, o6, o7}));
}
static List avm_of(E o0, E o1, E o2, E o3,
E o4, E o5, E o6, E o7,
E o8) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2, o3, o4, o5, o6, o7, o8}));
}
static List avm_of(E o0, E o1, E o2, E o3,
E o4, E o5, E o6, E o7,
E o8, E o9) {
return new UnmodifiableArrayList<>(IObjects.requireNonNullElements(
new IObject[]{o0, o1, o2, o3, o4, o5, o6, o7, o8, o9}));
}
static List avm_of(IObjectArray elements) {
var oa = ((ObjectArray) elements).getUnderlying();
var data = new IObject[oa.length];
for (int i = 0; i < oa.length; i++) {
data[i] = Objects.requireNonNull((IObject) oa[i]);
}
return new UnmodifiableArrayList<>(data);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy