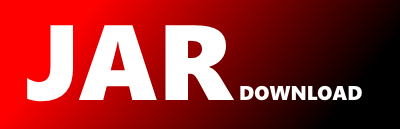
wallettemplate.utils.easing.ElasticInterpolator Maven / Gradle / Ivy
The newest version!
/*
* The MIT License (MIT)
*
* Copyright (c) 2013, Christian Schudt
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package wallettemplate.utils.easing;
import javafx.beans.property.DoubleProperty;
import javafx.beans.property.SimpleDoubleProperty;
/**
* This interpolator simulates an elastic behavior.
*
* The following curve illustrates the interpolation.
*
*
*
* The math in this class is taken from
* http://www.robertpenner.com/easing/.
*
* @author Christian Schudt
*/
public class ElasticInterpolator extends EasingInterpolator {
/**
* The amplitude.
*/
private DoubleProperty amplitude = new SimpleDoubleProperty(this, "amplitude", 1);
/**
* The number of oscillations.
*/
private DoubleProperty oscillations = new SimpleDoubleProperty(this, "oscillations", 3);
/**
* Default constructor. Initializes the interpolator with ease out mode.
*/
public ElasticInterpolator() {
this(EasingMode.EASE_OUT);
}
/**
* Constructs the interpolator with a specific easing mode.
*
* @param easingMode The easing mode.
*/
public ElasticInterpolator(EasingMode easingMode) {
super(easingMode);
}
/**
* Sets the easing mode.
*
* @param easingMode The easing mode.
* @see #easingModeProperty()
*/
public ElasticInterpolator(EasingMode easingMode, double amplitude, double oscillations) {
super(easingMode);
this.amplitude.set(amplitude);
this.oscillations.set(oscillations);
}
/**
* The oscillations property. Defines number of oscillations.
*
* @return The property.
* @see #getOscillations()
* @see #setOscillations(double)
*/
public DoubleProperty oscillationsProperty() {
return oscillations;
}
/**
* The amplitude. The minimum value is 1. If this value is < 1 it will be set to 1 during animation.
*
* @return The property.
* @see #getAmplitude()
* @see #setAmplitude(double)
*/
public DoubleProperty amplitudeProperty() {
return amplitude;
}
/**
* Gets the amplitude.
*
* @return The amplitude.
* @see #amplitudeProperty()
*/
public double getAmplitude() {
return amplitude.get();
}
/**
* Sets the amplitude.
*
* @param amplitude The amplitude.
* @see #amplitudeProperty()
*/
public void setAmplitude(final double amplitude) {
this.amplitude.set(amplitude);
}
/**
* Gets the number of oscillations.
*
* @return The oscillations.
* @see #oscillationsProperty()
*/
public double getOscillations() {
return oscillations.get();
}
/**
* Sets the number of oscillations.
*
* @param oscillations The oscillations.
* @see #oscillationsProperty()
*/
public void setOscillations(final double oscillations) {
this.oscillations.set(oscillations);
}
@Override
protected double baseCurve(double v) {
if (v == 0) {
return 0;
}
if (v == 1) {
return 1;
}
double p = 1.0 / oscillations.get();
double a = amplitude.get();
double s;
if (a < Math.abs(1)) {
a = 1;
s = p / 4;
} else {
s = p / (2 * Math.PI) * Math.asin(1 / a);
}
return -(a * Math.pow(2, 10 * (v -= 1)) * Math.sin((v - s) * (2 * Math.PI) / p));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy