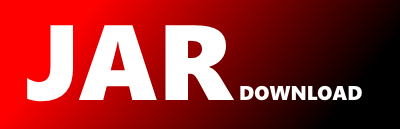
fr.adbonnin.cz2128.json.repository.MapRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cz2128 Show documentation
Show all versions of cz2128 Show documentation
Repository library based on FasterXML / Jackson.
The newest version!
package fr.adbonnin.cz2128.json.repository;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.ObjectReader;
import fr.adbonnin.cz2128.collect.IteratorUtils;
import fr.adbonnin.cz2128.json.JsonException;
import fr.adbonnin.cz2128.json.JsonProvider;
import fr.adbonnin.cz2128.json.iterator.FieldObjectIterator;
import fr.adbonnin.cz2128.json.iterator.FieldValueObjectIterator;
import java.io.IOException;
import java.util.*;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import static java.util.Objects.requireNonNull;
public abstract class MapRepository extends BaseRepository {
private final ObjectReader reader;
protected abstract long saveAll(JsonParser parser, JsonGenerator generator, Map elements) throws IOException;
protected abstract long deleteAll(JsonParser parser, JsonGenerator generator, Predicate super Map.Entry> predicate) throws IOException;
public MapRepository(JsonProvider provider, ObjectReader reader) {
super(provider);
this.reader = requireNonNull(reader);
}
public ObjectReader getReader() {
return reader;
}
@Override
public long count() {
return withFieldIterator(IteratorUtils::count);
}
public long count(Predicate super T> predicate) {
return withEntryIterator(field -> true, predicate, IteratorUtils::count);
}
public long countFields(Predicate predicate) {
return withEntryIterator(predicate, value -> true, IteratorUtils::count);
}
public long countEntries(Predicate super Map.Entry> predicate) {
return withEntryIterator(iterator -> {
final Iterator> filtered = IteratorUtils.filter(iterator, predicate);
return IteratorUtils.count(filtered);
});
}
public Optional> findFirst(Predicate super T> predicate) {
return withEntryIterator(field -> true, predicate, IteratorUtils::first);
}
public Optional> findFirstField(Predicate predicate) {
return withEntryIterator(predicate, value -> true, IteratorUtils::first);
}
public Optional> findFirstEntry(Predicate super Map.Entry> predicate) {
return withEntryIterator(iterator -> IteratorUtils.find(iterator, predicate));
}
public Map findAll() {
return findAll(entry -> true);
}
public Map findAll(Predicate super Map.Entry> predicate) {
return withEntryIterator(iterator -> {
final Iterator extends Map.Entry> filtered = IteratorUtils.filter(iterator, predicate);
return IteratorUtils.newLinkedHashMap(filtered);
});
}
@Override
public R withIterator(Function, ? extends R> function) {
return withEntryIterator(iterator -> {
final Iterator valueIterator = IteratorUtils.valueIterator(iterator);
return function.apply(valueIterator);
});
}
public R withFieldIterator(Function, R> function) {
return withParser(parser -> {
final FieldObjectIterator fieldIterator = new FieldObjectIterator(parser);
return function.apply(fieldIterator);
});
}
public R withEntryIterator(Function>, R> function) {
return withEntryIterator(field -> true, value -> true, function);
}
private R withEntryIterator(Predicate fieldPredicate, Predicate super T> valuePredicate, Function>, R> function) {
return withParser(parser -> {
final FieldValueObjectIterator fieldValueIterator = new FieldValueObjectIterator<>(parser, reader, fieldPredicate, valuePredicate);
return function.apply(fieldValueIterator);
});
}
public R withEntryStream(Function>, R> function) {
return withEntryIterator(iterator -> {
final Spliterator> spliterator = Spliterators.spliteratorUnknownSize(iterator, 0);
final Stream> stream = StreamSupport.stream(spliterator, false);
return function.apply(stream);
});
}
public boolean save(String key, T element) {
return saveAll(Collections.singletonMap(key, element)) != 0;
}
public long saveAll(Map elements) {
return withGenerator((parser, generator) -> {
try {
return saveAll(parser, generator, elements);
}
catch (IOException e) {
throw new JsonException(e);
}
});
}
public boolean delete(String key) {
return deleteAll(e -> key.equals(e.getKey())) > 0;
}
public long deleteAll() {
return deleteAll(value -> true);
}
public long deleteAll(Collection elements) {
return deleteAll(e -> elements.contains(e.getKey()));
}
public long deleteAll(Predicate super Map.Entry> predicate) {
return withGenerator((parser, generator) -> {
try {
return deleteAll(parser, generator, predicate);
}
catch (IOException e) {
throw new JsonException(e);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy