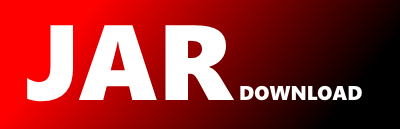
fr.avianey.androidsvgdrawable.maven.SvgDrawableMavenPlugin Maven / Gradle / Ivy
/*
* Copyright 2013, 2014, 2015 Antoine Vianey
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package fr.avianey.androidsvgdrawable.maven;
import fr.avianey.androidsvgdrawable.*;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import java.io.File;
import java.util.Set;
/**
* Goal which generates drawable from Scalable Vector Graphics (SVG) files.
*
* @author antoine vianey
*/
@Mojo(name = "gen")
public class SvgDrawableMavenPlugin extends AbstractMojo implements SvgDrawablePlugin.Parameters {
/**
* Directory of the svg resources to generate drawable from.
*/
@Parameter(required = true)
private Set from;
/**
* Location of the Android "./src/main/res/drawable(-.*)" directories :
*
* - drawable
* - drawable-hdpi
* - drawable-ldpi
* - drawable-mdpi
* - drawable-xhdpi
* - drawable-xxhdpi
* - drawable-xxxhdpi
*
*/
@Parameter(defaultValue = "${project.basedir}/src/main/res")
private File to;
/**
* Create a drawable-density directory when no directory exists for the
* given qualifiers.
* If set to false, the plugin will generate the drawable in the best
* matching directory :
*
* - match all of the qualifiers
* - no other matching directory with less qualifiers
*
*/
@Parameter(defaultValue = "true")
private boolean createMissingDirectories;
/**
* Enumeration of desired target densities.
* If no density specified, PNG are only generated to existing directories.
* If at least one density is specified, PNG are only generated in matching
* directories.
*/
@Parameter
private Density.Value[] targetedDensities;
/**
* Path to the 9-patch drawable configuration file.
*/
@Parameter
private File ninePatchConfig;
/**
* Path to the .svgmask directory.
* The {@link SvgDrawableMavenPlugin#from} directory will be use if not specified.
*/
@Parameter
private Set svgMaskFiles;
/**
* Path to a directory referencing additional svg resources to be taken in
* account for masking.
* The {@link SvgDrawableMavenPlugin#from} directory will be use if not specified.
*/
@Parameter
private Set svgMaskResourceFiles;
/**
* Path to the directory where masked svg files are generated.
* "target/generated-svg"
*/
@Parameter(readonly = true, defaultValue = "${project.build.directory}/generated-svg")
private File svgMaskedSvgOutputDirectory;
/**
* If set to true a mask combination will be ignored when a
* .svgmask use the same .svg resources in
* at least two different <image> tags.
*/
@Parameter(defaultValue = "true")
private boolean useSameSvgOnlyOnceInMask;
/**
* Overwrite existing generated resources.
* It's recommended to use {@link OverwriteMode#always} for tests and
* production releases.
*
* @see OverwriteMode
*/
@Parameter(defaultValue = "always", alias = "overwrite")
private OverwriteMode overwriteMode;
/**
*
* USE WITH CAUTION
* You'll more likely take time to set desired width and height properly
*
*
* When <SVG> attributes "x", "y", "width" and "height" are not
* present defines which element are taken in account to compute the Area Of
* Interest of the image. The plugin will output a WARNING log if no width
* or height are specified within the <svg> element.
*
* - all
* - This includes primitive paint, filtering, clipping and masking.
* - sensitive
* - This includes the stroked area but does not include the effects of clipping, masking or filtering.
* - geometry
* - This excludes any clipping, masking, filtering or stroking.
* - primitive
* - This is the painted region of fill and stroke but does not account for clipping, masking or filtering.
*
*
* @see BoundsType
*/
@Parameter(defaultValue = "sensitive")
private BoundsType svgBoundsType;
/**
* The output folder for the generated images.
*
* - drawable
* - mipmap
*
*
* @see OutputType
*/
@Parameter(defaultValue = "drawable")
private OutputType outputType;
/**
* The format for the generated images.
*
* - PNG
* - JPG
*
*
* @see OutputFormat
*/
@Parameter(defaultValue = "PNG")
private OutputFormat outputFormat;
/**
* The quality for the JPG output format.
*/
@Parameter(defaultValue = "85")
private int jpgQuality;
/**
* The background color to use when {@link OutputFormat#JPG} is specified.
* Default value is 0xFFFFFFFF (white)
*/
@Parameter(defaultValue = "-1")
private int jpgBackgroundColor;
public void execute() {
final SvgDrawablePlugin plugin = new SvgDrawablePlugin(this, new MavenLogger(getLog()));
plugin.execute();
}
@Override
public Iterable getFiles() {
return from;
}
@Override
public File getTo() {
return to;
}
@Override
public boolean isCreateMissingDirectories() {
return createMissingDirectories;
}
@Override
public OverwriteMode getOverwriteMode() {
return overwriteMode;
}
@Override
public Density.Value[] getTargetedDensities() {
return targetedDensities;
}
@Override
public File getNinePatchConfig() {
return ninePatchConfig;
}
@Override
public Iterable getSvgMaskFiles() {
return svgMaskFiles;
}
@Override
public Iterable getSvgMaskResourceFiles() {
return svgMaskResourceFiles;
}
@Override
public File getSvgMaskedSvgOutputDirectory() {
return svgMaskedSvgOutputDirectory;
}
@Override
public boolean isUseSameSvgOnlyOnceInMask() {
return useSameSvgOnlyOnceInMask;
}
@Override
public OutputType getOutputType() {
return outputType;
}
@Override
public OutputFormat getOutputFormat() {
return outputFormat;
}
@Override
public int getJpgQuality() {
return jpgQuality;
}
@Override
public int getJpgBackgroundColor() {
return jpgBackgroundColor;
}
@Override
public BoundsType getSvgBoundsType() {
return svgBoundsType;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy