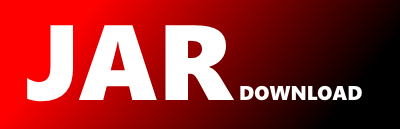
spoon.reflect.code.CtAbstractSwitch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spoon-core Show documentation
Show all versions of spoon-core Show documentation
cbc30506a032395e3b97b908b3d1f6240ce59db6
/*
* SPDX-License-Identifier: (MIT OR CECILL-C)
*
* Copyright (C) 2006-2023 INRIA and contributors
*
* Spoon is available either under the terms of the MIT License (see LICENSE-MIT.txt) or the Cecill-C License (see LICENSE-CECILL-C.txt). You as the user are entitled to choose the terms under which to adopt Spoon.
*/
package spoon.reflect.code;
import spoon.reflect.annotations.PropertyGetter;
import spoon.reflect.annotations.PropertySetter;
import spoon.reflect.declaration.CtElement;
import java.util.List;
import static spoon.reflect.path.CtRole.CASE;
import static spoon.reflect.path.CtRole.EXPRESSION;
/**
* This code element defines an abstract switch
* (either switch statement or switch expression).
*
* @param
* the type of the selector expression
*/
public interface CtAbstractSwitch extends CtElement {
/**
* Gets the selector. The type of the Expression must be char
,
* byte
, short
, int
,
* Character
, Byte
, Short
,
* Integer
, or an enum
type
*/
@PropertyGetter(role = EXPRESSION)
CtExpression getSelector();
/**
* Sets the selector. The type of the Expression must be char
,
* byte
, short
, int
,
* Character
, Byte
, Short
,
* Integer
, or an enum
type
*/
@PropertySetter(role = EXPRESSION)
> T setSelector(CtExpression selector);
/**
* Gets the list of cases defined for this switch.
*/
@PropertyGetter(role = CASE)
List> getCases();
/**
* Sets the list of cases defined for this switch.
*/
@PropertySetter(role = CASE)
> T setCases(List> cases);
/**
* Adds a case;
*/
@PropertySetter(role = CASE)
> T addCase(CtCase super S> c);
/**
* Adds a case at the specified position.
*
* @param type of the switch - {@link CtSwitch} or {@link CtSwitchExpression}
* @param position index at which the case needs to be inserted
* @param c case which has to be inserted in the switch block
* @return switch block in which the case is inserted
*/
@PropertySetter(role = CASE)
> T addCaseAt(int position, CtCase super S> c);
/**
* Removes a case;
*/
@PropertySetter(role = CASE)
boolean removeCase(CtCase super S> c);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy