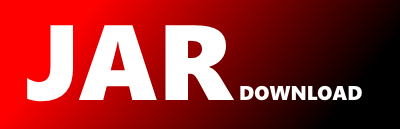
spoon.examples.delegate.template.DelegateTemplate Maven / Gradle / Ivy
The newest version!
package spoon.examples.delegate.template;
import java.util.ArrayList;
import java.util.List;
import java.util.TreeSet;
import spoon.reflect.Factory;
import spoon.reflect.declaration.CtClass;
import spoon.reflect.declaration.CtMethod;
import spoon.reflect.declaration.CtParameter;
import spoon.reflect.reference.CtTypeReference;
import spoon.template.Local;
import spoon.template.Parameter;
import spoon.template.Substitution;
import spoon.template.Template;
public class DelegateTemplate implements Template {
@Parameter
CtTypeReference> _DelegateType_;
@Parameter
CtTypeReference> _ReturnType_;
@Parameter
String _delegate_;
@Parameter
String _voidDelegate_;
@Parameter
List> _args_;
@Local
public DelegateTemplate(CtTypeReference> delegateType) {
this._DelegateType_ = delegateType;
}
@Local
public CtMethod> getDelegateMethod(CtClass> target, CtMethod> method) {
Factory f = target.getFactory();
boolean voidMethod = method.getType().equals(
f.Type().createReference(void.class));
if (voidMethod) {
_voidDelegate_ = method.getSimpleName();
} else {
this._ReturnType_ = method.getType();
_delegate_ = method.getSimpleName();
}
_args_ = new ArrayList>(method.getParameters());
CtMethod> delegateMethod;
if (voidMethod) {
delegateMethod = Substitution.substitute(target, this, f.Template()
.get(DelegateTemplate.class).getMethod(
"_voidDelegate_",
f.Type().createReference(List.class)));
} else {
delegateMethod = Substitution.substitute(target, this, f.Template()
.get(DelegateTemplate.class).getMethod(
"_delegate_", f.Type().createReference(List.class)));
}
delegateMethod
.setThrownTypes(new TreeSet>(
method.getThrownTypes()));
return delegateMethod;
}
_DelegateType_ delegate;
public DelegateTemplate(_DelegateType_ delegate) {
this.delegate = delegate;
}
@Local
public _ReturnType_ _delegate_(List> _args_) {
return delegate._delegate_(_args_);
}
@Local
public void _voidDelegate_(List> _args_) {
delegate._voidDelegate_(_args_);
}
}
interface _ReturnType_ {
}
interface _DelegateType_ {
_ReturnType_ _delegate_(List> _args_);
void _voidDelegate_(List> _args_);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy