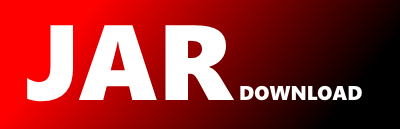
spoon.examples.distcalc.processing.NodeProcessor Maven / Gradle / Ivy
The newest version!
package spoon.examples.distcalc.processing;
import java.util.ArrayList;
import spoon.examples.distcalc.annotation.Node;
import spoon.examples.distcalc.template.ServicesImplTemplate;
import spoon.examples.distcalc.template._NodeImpl_;
import spoon.examples.distcalc.template._ReturnType_;
import spoon.processing.AbstractAnnotationProcessor;
import spoon.processing.Environment;
import spoon.processing.Severity;
import spoon.reflect.Factory;
import spoon.reflect.code.CtBlock;
import spoon.reflect.code.CtIf;
import spoon.reflect.code.CtInvocation;
import spoon.reflect.code.CtStatement;
import spoon.reflect.declaration.CtAnonymousExecutable;
import spoon.reflect.declaration.CtClass;
import spoon.reflect.declaration.CtInterface;
import spoon.reflect.declaration.CtMethod;
import spoon.reflect.declaration.CtPackage;
import spoon.reflect.declaration.CtParameter;
import spoon.reflect.declaration.ModifierKind;
import spoon.reflect.reference.CtTypeReference;
import spoon.template.Substitution;
import spoon.template.TemplateParameter;
public class NodeProcessor extends
AbstractAnnotationProcessor> {
public CtTypeReference> getNodeInterfaceReference(CtPackage p,
String funName) {
return getFactory().Type().createReference(
p.getQualifiedName() + ".Node_" + funName);
}
public static CtInterface> getNodeInterface(Factory f, _NodeImpl_ t,
CtPackage p, String funName) {
CtInterface> i = null;
i = f.Interface().get(p.getQualifiedName() + ".Node_" + funName);
if (i == null) {
i = f.Interface().create(p, "Node_" + funName);
i.getModifiers().add(ModifierKind.PUBLIC);
Substitution.insertAll(i, t);
}
return i;
}
public void process(Node node, CtMethod> method) {
Factory f = method.getFactory();
Environment env = f.getEnvironment();
CtPackage p = method.getParent(CtPackage.class);
CtClass
© 2015 - 2025 Weber Informatics LLC | Privacy Policy