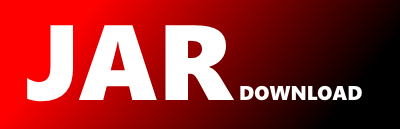
fr.ird.observe.spi.persistence.CommentableDataEntityDefinition Maven / Gradle / Ivy
package fr.ird.observe.spi.persistence;
/*-
* #%L
* ObServe Toolkit :: Common Persistence
* %%
* Copyright (C) 2008 - 2017 IRD, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
import fr.ird.observe.binder.data.DataEntityDtoBinderSupport;
import fr.ird.observe.binder.data.DataEntityReferenceBinderSupport;
import fr.ird.observe.dto.data.CommentableDto;
import fr.ird.observe.dto.data.DataDto;
import fr.ird.observe.dto.data.DataListDto;
import fr.ird.observe.dto.form.Form;
import fr.ird.observe.dto.reference.DataDtoReference;
import fr.ird.observe.dto.referential.ReferentialLocale;
import fr.ird.observe.entities.CommentableEntity;
import fr.ird.observe.entities.ObserveDataEntity;
import org.apache.commons.collections4.CollectionUtils;
import org.nuiton.topia.persistence.TopiaDao;
import org.nuiton.topia.persistence.TopiaEntities;
import org.nuiton.topia.persistence.TopiaPersistenceContext;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
import java.util.Objects;
import java.util.function.Function;
/**
* Created by tchemit on 03/09/17.
*
* @author Tony Chemit - [email protected]
*/
@SuppressWarnings("unchecked")
public abstract class CommentableDataEntityDefinition, E extends CommentableEntity, T extends TopiaDao> extends DataEntityDefinition {
public CommentableDataEntityDefinition(DataEntityDtoBinderSupport entityBinder, DataEntityReferenceBinderSupport entityReferenceBinder, Function daoFunction) {
super(entityBinder, entityReferenceBinder, daoFunction);
}
public Form> dataEntityToDataListForm(ReferentialLocale referentialLocale, E entity, Collection entityChildren, String propertyName) {
D dto = toDto(referentialLocale, entity);
List children;
if (CollectionUtils.isEmpty(entityChildren)) {
children = Collections.emptyList();
} else {
children = new LinkedList<>();
DataEntityDefinition> childrenContext = PersistenceModuleHelper.fromDataEntity(entityChildren);
DataEntityDtoBinderSupport childrenBinder = childrenContext.toEntityBinder();
for (EC entityChild : entityChildren) {
children.add(childrenBinder.toDto(referentialLocale, entityChild));
}
}
DataListDto, C> result = new DataListDto<>(propertyName, dto, children);
return Form.newFormDto((Class) DataListDto.class, result);
}
public Form> dataEntityToDataListForm(ReferentialLocale referentialLocale, E entity, String propertyName) {
D dto = toDto(referentialLocale, entity);
List children;
Collection entityChildren = entity.get(propertyName);
if (CollectionUtils.isEmpty(entityChildren)) {
children = Collections.emptyList();
} else {
children = new LinkedList<>();
DataEntityDefinition> childrenContext = PersistenceModuleHelper.fromDataEntity(entityChildren);
DataEntityDtoBinderSupport childrenBinder = childrenContext.toEntityBinder();
for (EC entityChild : entityChildren) {
children.add(childrenBinder.toDto(referentialLocale, entityChild));
}
}
DataListDto result = new DataListDto<>(propertyName, dto, children);
return Form.newFormDto((Class) DataListDto.class, result);
}
public void copyDataListDtoToEntity(ReferentialLocale referentialLocale, DataListDto dataListDo, E entity) {
copyToEntity(referentialLocale, dataListDo.getDto(), entity);
Collection children = entity.get(dataListDo.getPropertyName());
Objects.requireNonNull(children, String.format("Can't find collection %s on %s", dataListDo.getPropertyName(), entity));
ImmutableMap entitiesById = Maps.uniqueIndex(children, TopiaEntities.getTopiaIdFunction());
children.clear();
if (dataListDo.isNotEmpty()) {
List dtoChildren = dataListDo.getChildren();
DataEntityDefinition childEntityContext = PersistenceModuleHelper.fromDataDto(dtoChildren);
DataEntityDtoBinderSupport childBinder = childEntityContext.toEntityBinder();
for (C dtoChild : dtoChildren) {
EC entityChild;
if (dtoChild.isPersisted()) {
entityChild = entitiesById.get(dtoChild.getId());
childBinder.copyToEntity(referentialLocale, dtoChild, entityChild);
} else {
entityChild = childBinder.toEntity(referentialLocale, dtoChild);
}
children.add(entityChild);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy