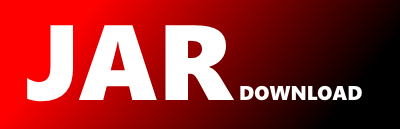
org.nuiton.topia.service.script.TopiaSqlScriptGeneratorRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topia-extension-script Show documentation
Show all versions of topia-extension-script Show documentation
ObServe ToPIA Script service module
package org.nuiton.topia.service.script;
/*-
* #%L
* ObServe Toolkit :: ToPIA Script service
* %%
* Copyright (C) 2017 - 2018 IRD, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import org.hibernate.dialect.Dialect;
import org.hibernate.dialect.H2Dialect;
import org.hibernate.dialect.PostgreSQL95Dialect;
import org.nuiton.topia.persistence.metadata.TopiaMetadataModel;
import org.nuiton.topia.service.script.request.CreateSchemaRequest;
import org.nuiton.topia.service.script.request.DeleteTablesRequest;
import org.nuiton.topia.service.script.request.ReplicateTablesRequest;
import org.nuiton.topia.service.script.request.SqlRequest;
import org.nuiton.topia.service.script.request.TopiaSqlTableSelectArgument;
import org.nuiton.topia.service.script.table.TopiaSqlTables;
import java.nio.file.Path;
import java.util.LinkedList;
import java.util.List;
/**
* Created by tchemit on 13/05/2018.
*
* @author Tony Chemit - [email protected]
*/
public class TopiaSqlScriptGeneratorRequest {
private final Path path;
private final List requests;
private boolean gzip;
private int readFetchSize;
private Class extends Dialect> dialect;
public TopiaSqlScriptGeneratorRequest(Path path) {
this.path = path;
this.requests = new LinkedList<>();
}
public int getReadFetchSize() {
return readFetchSize;
}
public Path getPath() {
return path;
}
public boolean isGzip() {
return gzip;
}
public Class extends Dialect> getDialect() {
return dialect;
}
public List getRequests() {
return requests;
}
public TopiaSqlScriptGeneratorRequest gzip(boolean gzip) {
this.gzip = gzip;
return this;
}
public TopiaSqlScriptGeneratorRequest readFetchSize(int readFetchSize) {
this.readFetchSize = readFetchSize;
return this;
}
public TopiaSqlScriptGeneratorRequest forH2() {
return dialect(H2Dialect.class);
}
public TopiaSqlScriptGeneratorRequest forPostgresql() {
return dialect(PostgreSQL95Dialect.class);
}
public TopiaSqlScriptGeneratorRequest dialect(Class extends Dialect> dialect) {
this.dialect = dialect;
return this;
}
public TopiaSqlScriptGeneratorRequest addCreateSchemaRequest(boolean addSchema, boolean addTables) {
return addRequest(new CreateSchemaRequest(dialect, addSchema, addTables));
}
public TopiaSqlScriptGeneratorRequest addReplicateTablesRequest(TopiaSqlTables tables, TopiaSqlTableSelectArgument selectArgument, TopiaMetadataModel metadataModel) {
return addRequest(new ReplicateTablesRequest(tables, selectArgument, metadataModel));
}
public TopiaSqlScriptGeneratorRequest addDeleteTablesRequest(TopiaSqlTables tables, TopiaSqlTableSelectArgument selectArgument) {
return addRequest(new DeleteTablesRequest(tables, selectArgument));
}
public TopiaSqlScriptGeneratorRequest addRequest(SqlRequest request) {
requests.add(request);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy