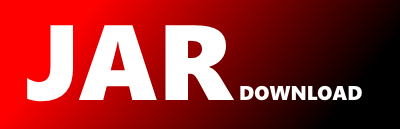
fr.ird.observe.toolkit.maven.plugin.ToolboxMojoSupport Maven / Gradle / Ivy
package fr.ird.observe.toolkit.maven.plugin;
/*-
* #%L
* ObServe Toolkit :: Maven plugin
* %%
* Copyright (C) 2017 - 2022 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.maven.artifact.Artifact;
import org.apache.maven.model.Resource;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* Created on 31/08/16.
*
* @author Tony Chemit - [email protected]
*/
public abstract class ToolboxMojoSupport extends AbstractMojo {
@Parameter(defaultValue = "${project}", required = true, readonly = true)
private MavenProject project;
protected abstract void doAction() throws Exception;
public abstract boolean isVerbose();
public abstract void setVerbose(boolean verbose);
protected abstract boolean isSkip();
@Override
public final void execute() throws MojoExecutionException, MojoFailureException {
if (getLog().isDebugEnabled()) {
// always be verbose in debug mode
setVerbose(true);
}
if (isSkip()) {
return;
}
try {
doAction();
} catch (MojoFailureException | MojoExecutionException e) {
throw e;
} catch (Exception e) {
throw new MojoExecutionException(
"could not execute goal " + getClass().getSimpleName() + " for reason : " + e.getMessage(), e);
}
}
public MavenProject getProject() {
return project;
}
public void setProject(MavenProject project) {
this.project = project;
}
/**
* Init mojo classLoader.
*
* @param project the maven project
* @param src the source directory
* @param addSourcesToClassPath a flag to a maven sources to classLoader
* @param testPhase a flag to specify if we are in a test phase (changes the classLoader)
* @param addResourcesToClassPath flag to add maven's resources to classLoader
* @param addCompileClassPath flag to add maven's project compile classPath to classLoader
* @param addProjectClassPath flag to add maven project dependencies to classLoader
* @return the new classLoader
* @throws MalformedURLException if an url was not correct
*/
protected URLClassLoader initClassLoader(
MavenProject project,
File src,
boolean addSourcesToClassPath,
boolean testPhase,
boolean addResourcesToClassPath,
boolean addCompileClassPath,
boolean addProjectClassPath) throws MalformedURLException {
URLClassLoader loader;
if (project != null) {
URLClassLoader result;
try {
Set doneSet = new HashSet<>();
List lUrls = new ArrayList<>();
List sources = project.getCompileSourceRoots();
if (addSourcesToClassPath) {
for (String source : sources) {
addDirectoryToUrlsList(new File(source), lUrls, doneSet);
}
if (testPhase) {
for (Object source :
project.getTestCompileSourceRoots()) {
addDirectoryToUrlsList(new File(source.toString()), lUrls, doneSet);
}
}
}
if (addResourcesToClassPath) {
for (Resource source : project.getResources()) {
addDirectoryToUrlsList(new File(source.getDirectory()), lUrls, doneSet);
}
}
if (testPhase && addCompileClassPath) {
addDirectoryToUrlsList(new File(project.getBuild().getOutputDirectory()), lUrls, doneSet);
}
if (src != null) {
addDirectoryToUrlsList(src, lUrls, doneSet);
}
if (addProjectClassPath) {
getLog().info("use project compile scope class-path");
// add also all dependencies of the project in compile
// scope
Set> artifacts = project.getArtifacts();
for (Object o : artifacts) {
Artifact a = (Artifact) o;
addDirectoryToUrlsList(a.getFile(), lUrls, doneSet);
}
}
result = new URLClassLoader(lUrls.toArray(new URL[0]), getClass().getClassLoader());
} catch (IOException e) {
throw new RuntimeException(
"Can't create ClassLoader for reason " +
e.getMessage(), e);
}
loader = result;
} else {
List lUrls = new ArrayList<>();
if (addSourcesToClassPath) {
lUrls.add(src.toURI().toURL());
}
loader = new URLClassLoader(lUrls.toArray(new URL[0]), getClass().getClassLoader());
}
if (isVerbose()) {
for (URL entry : loader.getURLs()) {
getLog().info("classpath : " + entry);
}
}
return loader;
}
/**
* Add the given {@code directory} in {@code urls} if not already included.
*
* Note: We use a extra list to store file string representation,
* since we do NOT want any url resolution and the
* {@link URL#equals(Object)} is doing some...
*
* @param directory the directory to insert in {@code urls}
* @param urls list of urls
* @param done list of string representation of urls
* @throws MalformedURLException if pb while converting file to url
* @since 1.1.0
*/
protected void addDirectoryToUrlsList(
File directory,
List urls,
Set done) throws MalformedURLException {
// do the comparison on a String to avoid url to be resolved (in URL.equals method)
addUrlToUrlsList(directory.toURI().toURL(), urls, done);
}
/**
* Add the given {@code url} in {@code urls} if not already included.
*
* Note: We use a extra list to store file string representation,
* since we do NOT want any url resolution and the
* {@link URL#equals(Object)} is doing some..
*
* @param url the url to insert in {@code urls}
* @param urls list of urls
* @param done list of string representation of urls
* @since 1.1.0
*/
protected void addUrlToUrlsList(URL url, List urls, Set done) {
// do the comparison on a String to avoid url to be resolved (in URL.equals method)
String u = url.toString();
if (!done.contains(u)) {
done.add(u);
urls.add(url);
}
}
/**
* Add a given directory in maven project's compile source roots (if not already present).
*
* @param srcDir the location to include in compile source roots
*/
protected void addCompileSourceRoots(File srcDir) {
if (!getProject().getCompileSourceRoots().contains(srcDir.getPath())) {
if (isVerbose()) {
getLog().info("adding source roots : " + srcDir.getPath());
}
getProject().addCompileSourceRoot(srcDir.getPath());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy