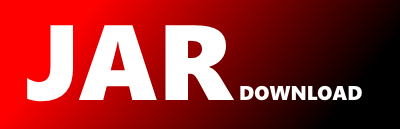
fr.ird.observe.toolkit.maven.plugin.fixtures.FixturesModel Maven / Gradle / Ivy
package fr.ird.observe.toolkit.maven.plugin.fixtures;
/*-
* #%L
* ObServe Toolkit :: Maven plugin
* %%
* Copyright (C) 2017 - 2022 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.dto.data.DataDto;
import fr.ird.observe.dto.data.EditableDto;
import fr.ird.observe.dto.referential.ReferentialDto;
import fr.ird.observe.spi.module.BusinessDataPackage;
import fr.ird.observe.spi.module.BusinessModule;
import fr.ird.observe.spi.module.BusinessProject;
import fr.ird.observe.spi.module.BusinessProjectVisitor;
import fr.ird.observe.spi.module.BusinessReferentialPackage;
import fr.ird.observe.spi.module.BusinessSubModule;
import fr.ird.observe.test.ToolkitFixtures;
import fr.ird.observe.toolkit.maven.plugin.server.html.model.Requests;
import io.ultreia.java4all.util.SortedProperties;
import io.ultreia.java4all.util.Version;
import org.apache.maven.plugin.logging.Log;
import java.io.BufferedReader;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.TreeMap;
/**
* FIXME Generate this model in navigation meta model, so after just a dummy generation and a concrete model to see.
* Created on 23/11/2021.
*
* @author Tony Chemit - [email protected]
* @since 5.0.56
*/
public class FixturesModel {
private final Path variablesPath;
private final SortedProperties variables;
private final Map dataPaths;
private final Map referentialPaths;
private final Map initPaths;
private final Map getByModule;
private final Map getByPackage;
private final Map> types;
private final Path fixturesPath;
private final Version modelVersion;
private final Version applicationVersion;
static class Builder {
private final Log log;
private final Path variablesPath;
private final Version modelVersion;
private final Version applicationVersion;
private final SortedProperties variables;
private final Map initPaths;
private final Map getByModule;
private final Map getByPackage;
private final Map dataPaths;
private final Map referentialPaths;
private final Map> types;
private final Path fixturesPath;
public Builder(Log log, Path fixturesPath, Version modelVersion, Version applicationVersion) {
this.log = log;
this.fixturesPath = fixturesPath;
this.variablesPath = fixturesPath.resolve("variables.properties");
this.modelVersion = modelVersion;
this.applicationVersion = applicationVersion;
this.variables = ToolkitFixtures.loadVariables(variablesPath);
this.getByModule = new LinkedHashMap<>();
this.getByPackage = new LinkedHashMap<>();
this.dataPaths = new TreeMap<>();
this.referentialPaths = new TreeMap<>();
this.initPaths = new TreeMap<>();
this.types = new TreeMap<>();
}
public FixturesModel build(BusinessProject businessProject) throws IOException {
if (Files.exists(variablesPath)) {
try (BufferedReader writer = Files.newBufferedReader(variablesPath)) {
variables.load(writer);
}
}
businessProject.accept(new BusinessProjectVisitor() {
@Override
public void enterSubModuleDataType(BusinessModule module, BusinessSubModule subModule, BusinessDataPackage dataPackage, Class extends DataDto> dtoType) {
Class> mainDtoType = businessProject.getMapping().getMainDtoType(dtoType);
if (mainDtoType != dtoType && !(EditableDto.class.isAssignableFrom(dtoType))) {
return;
}
String simpleName = dtoType.getSimpleName().replace("Dto", "");
String id = "data." + module.getName() + "." + subModule.getName() + "." + simpleName + ".id";
Path path = BusinessProject.getDtoVariablePath(Path.of(""), module, subModule, dtoType);
String property = variables.getProperty(id);
if (property == null || property.isEmpty()) {
log.warn("Could not find id for " + id);
return;
}
dataPaths.put(id, path);
types.put(id, dtoType);
}
@Override
public void enterProject(BusinessProject project) {
Path initPath = Path.of("").resolve("init");
initPaths.put(Requests.Ping.name(), initPath);
initPaths.put(Requests.Open.name(), initPath);
initPaths.put(Requests.Close.name(), initPath);
initPaths.put(Requests.Information.name(), initPath);
// add GetAll referential for project
getByModule.put(null, Path.of("").resolve("referential"));
}
@Override
public void enterModule(BusinessModule module) {
// add GetAll referential for module
Path path = BusinessProject.getDtoModulePath(Path.of(""), module, false);
getByModule.put(module.getName(), path);
}
@Override
public void enterSubModuleReferentialPackage(BusinessModule module, BusinessSubModule subModule, BusinessReferentialPackage referentialPackage) {
// add GetAll for subModule
Path path = BusinessProject.getDtoPackagePath(Path.of(""), module, subModule, false);
getByPackage.put(module.getName() + "_" + subModule.getName(), path);
}
@Override
public void enterSubModuleReferentialType(BusinessModule module, BusinessSubModule subModule, BusinessReferentialPackage referentialPackage, Class extends ReferentialDto> dtoType) {
String simpleName = dtoType.getSimpleName().replace("Dto", "");
String id = "referential." + module.getName() + "." + subModule.getName() + "." + simpleName + ".id";
Path path = BusinessProject.getDtoVariablePath(Path.of(""), module, subModule, dtoType);
String property = variables.getProperty(id);
if (property == null || property.isBlank()) {
log.warn("Could not find id for " + id);
return;
}
referentialPaths.put(id, path);
types.put(id, dtoType);
}
});
return new FixturesModel(variablesPath,
variables,
dataPaths,
referentialPaths,
initPaths,
getByModule,
getByPackage,
types,
fixturesPath,
modelVersion,
applicationVersion);
}
}
public static Builder create(Log log, Path fixturesPath, Version modelVersion, Version applicationVersion) {
return new Builder(log, fixturesPath, modelVersion, applicationVersion);
}
public FixturesModel(Path variablesPath,
SortedProperties variables,
Map dataPaths,
Map referentialPaths,
Map initPaths,
Map getByModule,
Map getByPackage,
Map> types,
Path fixturesPath,
Version modelVersion,
Version applicationVersion) {
this.variablesPath = variablesPath;
this.variables = variables;
this.dataPaths = dataPaths;
this.referentialPaths = referentialPaths;
this.initPaths = initPaths;
this.getByModule = getByModule;
this.getByPackage = getByPackage;
this.types = types;
this.fixturesPath = fixturesPath;
this.applicationVersion = applicationVersion;
this.modelVersion = modelVersion;
}
public Version getModelVersion() {
return modelVersion;
}
public Version getApplicationVersion() {
return applicationVersion;
}
public Path getVariablesPath() {
return variablesPath;
}
public SortedProperties getVariables() {
return variables;
}
public Map getDataPaths() {
return dataPaths;
}
public Map getReferentialPaths() {
return referentialPaths;
}
public Map> getTypes() {
return types;
}
public Path getFixturesPath() {
return fixturesPath;
}
public Map getGetByModule() {
return getByModule;
}
public Map getGetByPackage() {
return getByPackage;
}
public Map getInitPaths() {
return initPaths;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy