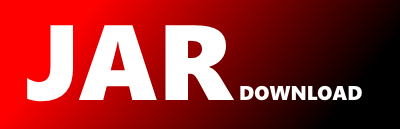
fr.ird.observe.toolkit.maven.plugin.navigation.tree.BeanTemplate Maven / Gradle / Ivy
package fr.ird.observe.toolkit.maven.plugin.navigation.tree;
/*-
* #%L
* ObServe Toolkit :: Maven plugin
* %%
* Copyright (C) 2017 - 2022 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.navigation.tree.NavigationNodeType;
import fr.ird.observe.spi.navigation.model.MetaModelNodeLinkMultiplicity;
import fr.ird.observe.spi.navigation.model.MetaModelNodeType;
import fr.ird.observe.spi.navigation.model.tree.TreeNodeLink;
import fr.ird.observe.spi.navigation.model.tree.TreeNodeModel;
import fr.ird.observe.spi.navigation.model.tree.TreeProjectModel;
import java.util.Date;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.TreeMap;
import java.util.stream.Collectors;
import static fr.ird.observe.toolkit.maven.plugin.navigation.tree.GenerateTreeModelSupport.BEAN;
/**
* Created on 03/01/2022.
*
* @author Tony Chemit - [email protected]
* @since 5.0.64
*/
public class BeanTemplate {
public static final String MAP_BUILDER = "ImmutableMap.<%1$s, %2$s>builder()\n" +
" %4$s%3$s\n" +
" %4$s.build()";
private final GenerateTreeModelSupport generator;
public BeanTemplate(GenerateTreeModelSupport generator) {
this.generator = generator;
}
public String generateContent(TreeProjectModel project, TreeNodeModel node) {
Map defaultStates = generateDefaultStates(project, node);
String map = generateMap(defaultStates, "ToolkitTreeNodeBeanState>", "Object", " ");
MetaModelNodeType nodeType = node.getNodeType();
return String.format(generator.getBeanTemplate()
, generator.getNodePackageName(node)
, new Date()
, node.getSimpleName() + generator.getSuffix() + BEAN
, nodeType == null ? "Root" : nodeType.name()
, map
, getClass().getName());
}
private Map generateDefaultStates(TreeProjectModel project, TreeNodeModel node) {
boolean notRoot = !node.isRoot();
boolean addIconPath = false;
boolean addPath = false;
boolean addReferentialType = false;
boolean addDataType = false;
boolean addFilterType = false;
boolean capabilitySelectReferenceContainer = false;
boolean capabilityEditReferenceContainer = false;
boolean multiple = false;
boolean capabilityLeaf = node.isLeaf();
boolean capabilitySelectReference = false;
boolean capabilityEditReference = false;
Map mappingState = new LinkedHashMap<>();
if (!capabilityLeaf) {
for (TreeNodeLink link : node.getChildren()) {
TreeNodeModel child = project.getNode(link.getTargetClassName()).orElseThrow();
String propertyName = link.getPropertyName();
mappingState.put("\"" + propertyName + "\"", generator.getNodeFullyQualifiedName(child.getClassName()) + ".class");
if (child.isEdit()) {
capabilitySelectReferenceContainer = true;
}
if (child.isOpen() || child.isRootOpen()) {
capabilityEditReferenceContainer = true;
}
}
}
String stateType;
String textState = null;
String dtoType = generator.getDtoFullyQualifiedName(node.getType());
Optional nodeLink;
if (notRoot) {
addIconPath = true;
boolean addText = false;
nodeLink = project.getNodeLink(node);
switch (Objects.requireNonNull(node.getNodeType())) {
case ReferentialPackage:
addPath = true;
break;
case ReferentialType:
addPath = true;
addReferentialType = true;
break;
case RootOpen:
addPath = true;
multiple = true;
addDataType = true;
capabilityEditReference = true;
capabilitySelectReference = true;
break;
case Open:
addDataType = true;
capabilityEditReference = true;
capabilitySelectReference = true;
break;
case RootOpenFilter: {
multiple = true;
addPath = true;
addFilterType = true;
}
break;
case OpenList: {
multiple = true;
addPath = true;
addDataType = true;
TreeNodeLink nodeLinkModel = node.getChildren().get(0);
textState = String.format("fr.ird.observe.spi.decoration.I18nDecoratorHelper.getPropertyI18nKey(%s.class, \"list.title\")", generator.getDtoFullyQualifiedName(nodeLinkModel.getTargetClassName()));
}
break;
case Edit:
addText = true;
addPath = true;
addDataType = true;
capabilitySelectReference = true;
break;
case Table:
case Simple:
addText = true;
addPath = true;
addDataType = true;
break;
}
if (addText) {
textState = String.format("fr.ird.observe.spi.decoration.I18nDecoratorHelper.getTypeKey(%s.class)", dtoType);
}
stateType = node.getNodeType().name();
multiple |= nodeLink.map(TreeNodeLink::getMultiplicity).map(m -> m.equals(MetaModelNodeLinkMultiplicity.MANY)).orElse(false);
} else {
stateType = NavigationNodeType.Root.name();
nodeLink = Optional.empty();
}
Map defaultStates = new TreeMap<>();
defaultStates.put("STATE_TYPE", NavigationNodeType.class.getSimpleName() + "." + stateType);
defaultStates.put("STATE_NODE_MAPPING", generateMap(mappingState, "String", "Class>", " "));
if (textState != null) {
defaultStates.put("STATE_TEXT", textState);
}
if (addPath) {
String statePath = node.getStatePath(project);
if (statePath != null) {
if (node.isRootOpen()) {
defaultStates.put("STATE_STANDALONE_PATH", String.format("\"%s\"", statePath));
} else {
defaultStates.put("STATE_PATH", String.format("\"%s\"", statePath));
}
}
}
if (addIconPath) {
defaultStates.put("STATE_ICON_PATH", String.format("\"navigation.%1$s\"", node.getIconPath()));
}
if (addDataType) {
defaultStates.put("STATE_DATA_TYPE", dtoType + ".class");
}
if (addReferentialType) {
defaultStates.put("STATE_REFERENTIAL_TYPE", dtoType + ".class");
}
if (addFilterType) {
defaultStates.put("STATE_FILTER_TYPE", dtoType + ".class");
defaultStates.put("STATE_FILTER_NAME", String.format("\"%s\"", nodeLink.orElseThrow().getPropertyName()));
defaultStates.put("STATE_CAPABILITY_FILTER", "true");
}
if (multiple) {
defaultStates.put("STATE_MULTIPLICITY", "true");
}
if (capabilitySelectReference) {
defaultStates.put("STATE_CAPABILITY_SELECT_REFERENCE", "true");
}
if (capabilityEditReference) {
defaultStates.put("STATE_CAPABILITY_EDIT_REFERENCE", "true");
}
if (capabilityLeaf) {
defaultStates.put("STATE_CAPABILITY_LEAF", "true");
} else {
defaultStates.put("STATE_CAPABILITY_CONTAINER", "true");
}
if (capabilitySelectReferenceContainer) {
defaultStates.put("STATE_CAPABILITY_SELECT_REFERENCE_CONTAINER", "true");
}
if (capabilityEditReferenceContainer) {
defaultStates.put("STATE_CAPABILITY_EDIT_REFERENCE_CONTAINER", "true");
}
return defaultStates;
}
private String generateMap(Map defaultStates, String keyType, String valueType, String prefix) {
String map;
if (defaultStates.isEmpty()) {
map = "ImmutableMap.of()";
} else {
List collect = defaultStates.entrySet().stream().map(e -> prefix + ".put(" + e.getKey() + ", " + e.getValue() + ")\n").collect(Collectors.toList());
map = String.format(MAP_BUILDER, keyType, valueType, String.join(" ", collect).substring(2).trim(), prefix);
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy