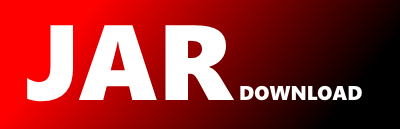
fr.ird.observe.toolkit.maven.plugin.persistence.GenerateMigrationScriptRunner Maven / Gradle / Ivy
package fr.ird.observe.toolkit.maven.plugin.persistence;
/*-
* #%L
* ObServe Toolkit :: Maven plugin
* %%
* Copyright (C) 2017 - 2022 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.dto.db.configuration.topia.ObserveDataSourceConfigurationTopiaH2;
import fr.ird.observe.entities.ToolkitTopiaApplicationContextSupport;
import fr.ird.observe.spi.migration.ByMajorMigrationVersionResource;
import fr.ird.observe.spi.split.PersistenceScriptHelper;
import fr.ird.observe.spi.split.ScriptSplitter;
import fr.ird.observe.toolkit.maven.plugin.PersistenceRunner;
import org.nuiton.topia.persistence.script.TopiaSqlScript;
import java.io.IOException;
import java.nio.file.Path;
import java.util.Objects;
import java.util.function.BiFunction;
/**
* Created on 24/11/2021.
*
* @author Tony Chemit - [email protected]
* @since 5.0.56
*/
public class GenerateMigrationScriptRunner extends PersistenceRunner {
private MigrationScriptTask task;
public void setTask(MigrationScriptTask task) {
this.task = task;
}
@Override
public void init() {
super.init();
Objects.requireNonNull(task);
}
@Override
public void run() {
Path scriptPath = sourceDirectory.resolve("db").resolve("migration").resolve(ByMajorMigrationVersionResource.getMajor(modelVersion)).resolve(modelVersion.getVersion());
ObserveDataSourceConfigurationTopiaH2 configuration = createConfiguration();
try {
try (ToolkitTopiaApplicationContextSupport> topiaApplicationContext = createTopiaApplicationContext(configuration)) {
run(true, scriptPath, topiaApplicationContext, (t, p) -> {
try {
return t.getSqlService().generateH2Schema(p, true);
} catch (IOException e) {
throw new IllegalStateException("Can't generate schema file at " + p, e);
}
});
run(false, scriptPath, topiaApplicationContext, (t, p) -> {
try {
return t.getSqlService().generatePgSchema(p, true);
} catch (IOException e) {
throw new IllegalStateException("Can't generate schema file at " + p, e);
}
});
}
} catch (Exception e) {
throw new IllegalStateException(e);
}
}
protected void run(boolean h2, Path scriptPath, ToolkitTopiaApplicationContextSupport> topiaApplicationContext, BiFunction, Path, TopiaSqlScript> scriptGenerator) throws IOException {
String scriptSuffix = (h2 ? "H2" : "PG") + ".sql";
TopiaSqlScript script = scriptGenerator.apply(topiaApplicationContext, getTemporaryPath().resolve(String.format("empty-schema-%s", scriptSuffix)));
ScriptSplitter.SplitSimpleResult context = ScriptSplitter.create(h2, script).splitSimple();
PersistenceScriptHelper.write(scriptPath, context, String.format("empty-%%s-%s", scriptSuffix), "", true);
for (MigrationScriptTask.ExtractSchemaTask task : task.getSchemaTasks()) {
String prefix = task.getPrefix();
String schemaName = task.getSchemaName();
String baseName = String.format("%s_%%s-%s-%s", prefix, schemaName, scriptSuffix);
ScriptSplitter.SplitSimpleResult filterOnSchema = ScriptSplitter.filterSchema(context, schemaName);
PersistenceScriptHelper.write(scriptPath, filterOnSchema, baseName, "-schema", false);
}
for (MigrationScriptTask.ExtractTableTask task : task.getTableTasks()) {
String prefix = task.getPrefix();
String schemaName = task.getSchemaName();
for (String name : task.getTableName()) {
String tableName = name.toLowerCase();
String baseName = String.format("%s_%%s-%s_%s-%s", prefix, schemaName, tableName, scriptSuffix);
ScriptSplitter.SplitSimpleResult filterOnSchema = ScriptSplitter.filterTable(context, schemaName, tableName);
PersistenceScriptHelper.write(scriptPath, filterOnSchema, baseName, "-table", false);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy