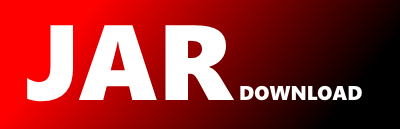
fr.ird.observe.toolkit.maven.plugin.server.responses.Template Maven / Gradle / Ivy
package fr.ird.observe.toolkit.maven.plugin.server.responses;
/*-
* #%L
* ObServe Toolkit :: Maven plugin
* %%
* Copyright (C) 2017 - 2022 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import fr.ird.observe.dto.ToolkitIdBean;
import fr.ird.observe.spi.json.java.util.DateAdapter;
import fr.ird.observe.toolkit.maven.plugin.server.TemplateHelper;
import org.apache.maven.plugin.logging.Log;
import org.nuiton.topia.persistence.filter.OrderEnum;
import org.nuiton.topia.persistence.filter.ToolkitRequestFilter;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Properties;
/**
* Created on 23/04/2021.
*
* @author Tony Chemit - [email protected]
* @since 5.0.18
*/
public class Template extends fr.ird.observe.toolkit.maven.plugin.server.Template {
private final Gson gson;
private final Gson gsonWithNoNull;
private final DateAdapter dataAdapter;
private List requests;
private Properties variables;
private Path docDirectory;
public static String unboxVariable(String realKey) {
if (realKey.startsWith("$")) {
return realKey.replace("${", "").replace("}", "");
} else {
return realKey;
}
}
public Template(Log log, Path targetDirectory) {
super(log, "", "", targetDirectory);
this.dataAdapter = new DateAdapter();
this.gson = new GsonBuilder().registerTypeAdapter(java.util.Date.class, dataAdapter)
.setPrettyPrinting().serializeNulls().disableHtmlEscaping().create();
this.gsonWithNoNull = new GsonBuilder().registerTypeAdapter(java.util.Date.class, dataAdapter)
.setPrettyPrinting().disableHtmlEscaping().create();
}
public void init() throws IOException {
docDirectory = targetDirectory.resolve("doc").resolve("api").resolve("public");
Path requestPath = docDirectory.resolve("requests.text");
requests = Files.readAllLines(requestPath);
log.info(String.format("Found %d request(s) in public API.", requests.size()));
variables = new Properties();
URL resource = getClass().getClassLoader().getResource("fixtures/variables.properties");
try (InputStream inputStream = Objects.requireNonNull(resource).openStream()) {
variables.load(inputStream);
}
variables.setProperty("_host", "http://localhost:8080/observeweb");
variables.setProperty("_config.login", "admin");
variables.setProperty("_config.password", "a");
variables.setProperty("_config.databaseName", "9-tck");
variables.setProperty("_config.modelVersion", "9.0");
variables.setProperty("_referentialLocale", "FR");
}
public void generate() throws IOException {
doPing(docDirectory, variables);
doOpen(docDirectory, variables);
try {
doInformation(docDirectory, variables);
variables.setProperty("_config.loadReferential", "true");
variables.setProperty("_config.recursive", "true");
variables.setProperty("_config.prettyPrint", "true");
variables.setProperty("_config.serializeNulls", "true");
generateReferential(docDirectory, variables);
generateData(docDirectory, variables);
} finally {
doClose(docDirectory, variables);
}
}
private void doPing(Path docDirectory, Properties variables) throws IOException {
RequestModel request = loadRequest(docDirectory.resolve("init/Ping/request.json"));
Map, ?> response = (Map, ?>) request.invoke(gson, variables);
request.storeResponse(gson, gsonWithNoNull, response);
}
private void doOpen(Path docDirectory, Properties variables) throws IOException {
String authenticationToken;
RequestModel request = loadRequest(docDirectory.resolve("init/Open/request.json"));
Map, ?> response = (Map, ?>) request.invoke(gson, variables);
authenticationToken = (String) response.get("authenticationToken");
request.storeResponse(gson, gsonWithNoNull, response);
variables.setProperty("_authenticationToken", authenticationToken);
variables.remove("_config.login");
variables.remove("_config.password");
variables.remove("_config.databaseName");
variables.remove("_config.modelVersion");
log.info(String.format("Connexion with success: %s", authenticationToken));
}
private void doInformation(Path docDirectory, Properties variables) throws IOException {
RequestModel request = loadRequest(docDirectory.resolve("init/Information/request.json"));
Map, ?> response = (Map, ?>) request.invoke(gson, variables);
request.storeResponse(gson, gsonWithNoNull, response);
}
private void doClose(Path docDirectory, Properties variables) throws IOException {
RequestModel request = loadRequest(docDirectory.resolve("init/Close/request.json"));
Map, ?> response = (Map, ?>) request.invoke(gson, variables);
request.storeResponse(gson, gsonWithNoNull, response);
}
private void generateReferential(Path docDirectory, Properties variables) throws IOException {
for (String request : requests) {
if (request.contains("referential/") && request.contains("/GetAll/")) {
runGetAllReferentialRequest(docDirectory, variables, request);
}
if (request.contains("referential/") && request.contains("/Get/")) {
runGetReferentialRequest(docDirectory, variables, request);
}
}
}
private void generateData(Path docDirectory, Properties variables) throws IOException {
for (String request : requests) {
if (request.contains("data/") && request.contains("/Get/")) {
RequestModel getOneRequest = loadRequest(docDirectory.resolve(request));
String id = variables.getProperty(unboxVariable(getOneRequest.getParameter("filter.id")));
runGetOneRequest(docDirectory, request, variables, getOneRequest, id);
}
}
}
protected void runGetAllReferentialRequest(Path docDirectory, Properties variables, String request) throws IOException {
log.info(String.format("Load %s", request));
RequestModel getAllRequest = loadRequest(docDirectory.resolve(request));
getAllRequest.getParameters().remove("filter.id");
getAllRequest.getParameters().remove("filter.properties");
getAllRequest.getParameters().remove("config.loadReferential");
getAllRequest.getParameters().remove("config.recursive");
getAllRequest.getParameters().remove("config.serializeNulls");
Map, ?> getAllResponse = (Map, ?>) getAllRequest.invoke(gson, variables);
log.info(String.format("Loaded %s.", request));
TemplateHelper.reduceResult((Map, ?>) getAllResponse.get("content"));
getAllRequest.storeResponse(gson, gsonWithNoNull, getAllResponse);
}
protected void runGetReferentialRequest(Path docDirectory, Properties variables, String request) throws IOException {
log.info(String.format("Load %s", request));
RequestModel getOneRequest = loadRequest(docDirectory.resolve(request));
String filterId = unboxVariable(getOneRequest.getParameter("filter.id"));
String requestId = variables.getProperty(filterId);
log.info(String.format("Use id: %s → %s", filterId, requestId));
runGetOneRequest(docDirectory, request, variables, getOneRequest, requestId);
log.info(String.format("Loaded %s", request));
if (requestId == null || requestId.isBlank()) {
return;
}
getOneRequest.getParameters().remove("filter.id");
getOneRequest.getParameters().remove("filter.properties");
Map oneRequestParameters = getOneRequest.getParameters();
oneRequestParameters.remove("filter.id");
ToolkitRequestFilter filter = ToolkitRequestFilter.onProperties(Map.of("status", "enabled"),
Map.of("code", OrderEnum.ASC));
String filterJson = gson.toJson(filter);
oneRequestParameters.put("filter", URLEncoder.encode(filterJson, StandardCharsets.UTF_8));
Map, ?> getEnabledResponse = (Map, ?>) getOneRequest.invoke(gson, variables);
Object getEnabledContent = getEnabledResponse.get("content");
int enabledCount = count(getEnabledContent);
log.info(String.format("Loaded enabled by json %s.", enabledCount));
oneRequestParameters.remove("filter");
oneRequestParameters.put("filter.properties.status", "enabled");
oneRequestParameters.put("filter.orders.code", "DESC");
getEnabledResponse = (Map, ?>) getOneRequest.invoke(gson, variables);
Object getEnabledContent2 = getEnabledResponse.get("content");
int enabledCount2 = count(getEnabledContent2);
log.info(String.format("Loaded enabled by parameters %s.", enabledCount2));
}
protected void runGetOneRequest(Path docDirectory, String request, Properties variables, RequestModel getOneRequest, String id) throws IOException {
if (id != null && !id.isBlank()) {
log.info(String.format("Load %s → %s", getOneRequest.getPath(), id));
Map, ?> getOneResponse = (Map, ?>) getOneRequest.invoke(gson, variables);
List> content1 = (List>) getOneResponse.get("content");
if (content1.isEmpty()) {
log.warn("Could not find this resource!!!! " + getOneRequest.getParameter("filter.id"));
return;
}
Map, ?> content = (Map, ?>) content1.get(0);
TemplateHelper.reduceResult(content);
List> list = (List>) getOneResponse.get("references");
TemplateHelper.reduceResult(list);
getOneRequest.storeResponse(gson, gsonWithNoNull, getOneResponse);
log.info(String.format("Loaded %s → %s.", getOneRequest.getPath(), id));
String createPath = request.replace("Get/", "Create/");
if (requests.contains(createPath)) {
String topiaId = (String) content.get("topiaId");
String topiaCreateDate = (String) content.get("topiaCreateDate");
String lastUpdateDate = (String) content.get("lastUpdateDate");
ToolkitIdBean result = new ToolkitIdBean(topiaId, dataAdapter.deserializeToDate(lastUpdateDate));
result.setTopiaCreateDate(dataAdapter.deserializeToDate(topiaCreateDate));
content.remove("topiaId");
content.remove("topiaCreateDate");
content.remove("lastUpdateDate");
RequestModel updateRequest = loadRequest(docDirectory.resolve(createPath.replace("/Create/", "/Update/")));
updateRequest.storeContent(gson, gsonWithNoNull, content, result, variables);
result.setTopiaCreateDate(result.getLastUpdateDate());
RequestModel createRequest = loadRequest(docDirectory.resolve(createPath));
createRequest.storeContent(gson, gsonWithNoNull, content, result, variables);
}
}
}
private int count(Object content) {
return content instanceof Collection ? ((Collection>) content).size() : 1;
}
protected RequestModel loadRequest(Path path) throws IOException {
try (BufferedReader reader = Files.newBufferedReader(path)) {
return gson.fromJson(reader, RequestModel.class).setPath(path);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy