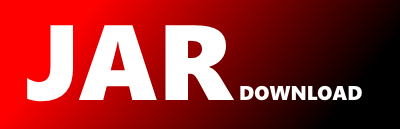
fr.ird.observe.toolkit.maven.plugin.service.ServiceGenerateLocalRunner Maven / Gradle / Ivy
package fr.ird.observe.toolkit.maven.plugin.service;
/*-
* #%L
* ObServe Toolkit :: Maven plugin
* %%
* Copyright (C) 2017 - 2022 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.security.Permission;
import fr.ird.observe.services.service.ObserveService;
import fr.ird.observe.toolkit.maven.plugin.MojoRunnable;
import io.ultreia.java4all.http.maven.plugin.HttpMojoSupport;
import io.ultreia.java4all.http.maven.plugin.model.ImportManager;
import io.ultreia.java4all.http.maven.plugin.model.MethodDescription;
import io.ultreia.java4all.util.TimeLog;
import org.nuiton.eugene.GeneratorUtil;
import org.nuiton.eugene.java.extension.ImportsManager;
import javax.annotation.processing.Generated;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Date;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Created on 21/01/2022.
*
* @author Tony Chemit - [email protected]
* @since 5.0.64
*/
public class ServiceGenerateLocalRunner extends MojoRunnable {
public static final String TEMPLATE = "\n" +
"package %1$s;\n\n" +
"\n" +
"%2$s\n" +
"@Generated(value = \"%3$s\", date = \"%4$s\")\n" +
"public class %5$s extends %5$sSupport {\n\n" +
"%6$s\n" +
"}";
public static final String CALL_BODY_NO_PERSISTENCE = "" +
" long t0 = TimeLog.getTime();\n" +
" try {\n" +
" %1$s" +
" } catch (Exception e) {\n" +
" recordError(e, \"%2$s\");\n" +
" throw e;\n" +
" } finally {\n" +
" logInvokeMethod(t0, \"%2$s\");\n" +
" }";
public static final String CALL_BODY_NO_TRANSACTION = "" +
" long t0 = TimeLog.getTime();\n" +
" initPersistence(\"%2$s\");\n" +
" try {\n" +
" %1$s" +
" } catch (Exception e) {\n" +
" recordError(e, \"%2$s\");\n" +
" throw e;\n" +
" } finally {\n" +
" logInvokeMethod(t0, \"%2$s\");\n" +
" }";
public static final String CALL_BODY_WITH_TRANSACTION = "" +
" long t0 = TimeLog.getTime();\n" +
" boolean newTransaction = %3$s(\"%2$s\", Permission.%4$s);\n" +
" try {\n" +
" %1$s" +
" } catch (Exception e) {\n" +
" recordError(e, \"%2$s\");\n" +
" throw e;\n" +
" } finally {\n" +
" logInvokeMethod(t0, \"%2$s\");\n" +
" closeTransaction(newTransaction, TimeLog.getTime(), \"%2$s\");\n" +
" }";
public static final String METHOD = " @Override\n" +
" public %1$s %2$s(%3$s) %4$s{\n" +
"%5$s\n" +
" }\n\n";
private Path sourceDirectory;
private Path targetDirectory;
private boolean withErrors = false;
public void setTargetDirectory(Path targetDirectory) {
this.targetDirectory = targetDirectory;
}
public void setSourceDirectory(Path sourceDirectory) {
this.sourceDirectory = sourceDirectory;
}
@Override
public void init() {
super.init();
Objects.requireNonNull(targetDirectory);
Objects.requireNonNull(sourceDirectory);
}
@Override
public void run() {
getLog().info(String.format("Will generate at %s", targetDirectory));
List> allServices = HttpMojoSupport.getAllServices(ObserveService.class);
for (Class> service : allServices) {
try {
processInput(service);
} catch (IOException e) {
throw new IllegalStateException("Some errors occurs, fix them to continue.", e);
}
}
if (withErrors) {
throw new IllegalStateException("Some errors occurs, fix them to continue.");
}
}
public void processInput(Class> serviceType) throws IOException {
String servicePackageName = serviceType.getPackage().getName();
String serviceSimpleName = serviceType.getSimpleName();
String serviceLocalPackageName = servicePackageName.replace(".services.", ".services.local.");
String superClassName = serviceSimpleName + "LocalSupport";
Map genericMapping = HttpMojoSupport.genericMapping(getLog(), ObserveService.class, serviceType);
String superClassFullyQualifiedName = serviceLocalPackageName + "." + superClassName;
String generatedClassName = serviceSimpleName + "Local";
String generatedFullyQualifiedClassName = serviceLocalPackageName + "." + generatedClassName;
Path superClassPackage = sourceDirectory.resolve(serviceLocalPackageName.replaceAll("\\.", "/"));
Path targetPackage = targetDirectory.resolve(serviceLocalPackageName.replaceAll("\\.", "/"));
Path superClassTargetFile = superClassPackage.resolve(superClassName + ".java");
if (Files.notExists(superClassTargetFile)) {
getLog().error(String.format("could not find class: %s", superClassFullyQualifiedName));
withErrors = true;
return;
}
Path targetFile = targetPackage.resolve(generatedClassName + ".java");
ImportManager importManager = new ImportManager();
Set methods = new LinkedHashSet<>();
MethodDescription.createMethodDescriptions(isVerbose() ? getLog() : null,
ObserveService.class,
serviceType,
methods,
method -> new ServiceLocalMethodDescriptionImpl(importManager, serviceType, method, genericMapping));
List methodsContent = new LinkedList<>();
ImportsManager realImportManager = new ImportsManager();
realImportManager.addImport(TimeLog.class);
realImportManager.addImport(Generated.class);
realImportManager.addExcludedPattern(".+\\." + generatedFullyQualifiedClassName);
getLog().info(String.format("will generate %d method(s) for %s", methods.size(), generatedFullyQualifiedClassName));
for (ServiceLocalMethodDescriptionImpl methodDescription : methods) {
String methodContent = addMethod(realImportManager, generatedFullyQualifiedClassName, methodDescription);
methodsContent.add(methodContent);
}
importManager.toDescription().forEach(t -> realImportManager.addImport(t.getName()));
List imports = realImportManager.getImports(serviceLocalPackageName).stream().map(s -> "import " + s + ";\n").collect(Collectors.toList());
String content = String.format(TEMPLATE,
serviceLocalPackageName,
String.join("", imports),
getClass().getName(),
new Date(),
generatedClassName,
String.join("", methodsContent));
store(targetFile, content);
}
private String addMethod(ImportsManager importManager, String className, ServiceLocalMethodDescriptionImpl methodDescription) {
Permission methodeCredentials = methodDescription.getMethodeCredentials();
boolean write = methodDescription.isWrite();
boolean noTransaction = methodDescription.isNoTransaction();
String methodName = methodDescription.getName();
String realReturnType = importAndSimplify(importManager, methodDescription.getReturnType());
StringBuilder exceptionsBuilder = new StringBuilder();
for (Class> exception : methodDescription.getExceptions()) {
exceptionsBuilder.append(", ").append(importAndSimplify(importManager, exception.getName()));
}
String exceptions = exceptionsBuilder.length() == 0 ? "" : "throws " + exceptionsBuilder.substring(2);
StringBuilder parametersBuilder = new StringBuilder();
StringBuilder parametersDeclarationBuilder = new StringBuilder();
int index = 0;
for (String parameterName : methodDescription.getParameterNames()) {
String parameterType = methodDescription.getParameterTypes().get(index++);
if (!parameterType.endsWith("[]")) {
parameterType = importAndSimplify(importManager, parameterType);
}
parametersBuilder.append(", ").append(parameterName);
parametersDeclarationBuilder.append(", ").append(parameterType).append(" ").append(parameterName);
}
String parameters = index == 0 ? "" : parametersBuilder.substring(2);
String parametersDeclaration = index == 0 ? "" : parametersDeclarationBuilder.substring(2);
String returnInvocation = methodDescription.getReturnInvocation();
@SuppressWarnings("SpellCheckingInspection") String superCall = String.format("%ssuper.%s(%s);\n", returnInvocation, methodName, parameters);
String bodyContent;
if (noTransaction) {
getLog().debug(String.format("No transaction required on %s for method: %s", className, methodName));
// no transaction in this call
bodyContent = String.format(CALL_BODY_NO_PERSISTENCE, superCall, methodName);
} else {
boolean noCredential = methodeCredentials == null;
if (noCredential) {
getLog().warn(String.format("No credential on %s for method: %s", className, methodName));
}
if (noCredential) {
// init persistence, but do not open transaction
bodyContent = String.format(CALL_BODY_NO_TRANSACTION, superCall, methodName);
} else {
// init persistence and create a new transaction if required
String initTransactionMethodName = write ? "initWriteTransaction" : "initReadTransaction";
importManager.importAndSimplify(Permission.class.getName());
bodyContent = String.format(CALL_BODY_WITH_TRANSACTION, superCall, methodName, initTransactionMethodName, methodeCredentials);
}
}
return String.format(METHOD, realReturnType, methodName, parametersDeclaration, exceptions, bodyContent);
}
public String importAndSimplify(ImportsManager manager, String imports) {
imports = Objects.requireNonNull(imports).trim();
// remove ... operator
if (imports.endsWith("...")) {
imports = imports.substring(0, imports.length() - 3);
return importAndSimplify(manager, imports) + "...";
}
Set typesList = GeneratorUtil.getTypesList(imports);
if (typesList.size() > 1) {
// Can't simplify in this case (need to rebuild the hole thing, not now...)
typesList.forEach(manager::addImport);
return imports;
}
String oneType = typesList.iterator().next();
return manager.importAndSimplify(oneType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy