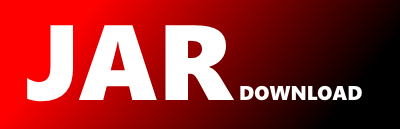
fr.ird.observe.toolkit.maven.plugin.tck.GenerateTckArchive Maven / Gradle / Ivy
package fr.ird.observe.toolkit.maven.plugin.tck;
/*-
* #%L
* ObServe Toolkit :: Maven plugin
* %%
* Copyright (C) 2017 - 2022 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.dto.db.configuration.topia.ObserveDataSourceConfigurationTopiaH2;
import fr.ird.observe.entities.ToolkitTopiaApplicationContextSupport;
import fr.ird.observe.spi.split.PersistenceScriptHelper;
import fr.ird.observe.test.DatabaseName;
import fr.ird.observe.toolkit.maven.plugin.PersistenceRunner;
import io.ultreia.java4all.util.Zips;
import org.nuiton.topia.persistence.script.SqlScriptConsumer;
import org.nuiton.topia.persistence.script.TopiaSqlScript;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* Created on 22/11/2021.
*
* @author Tony Chemit - [email protected]
* @since 5.0.55
*/
public class GenerateTckArchive extends PersistenceRunner {
private Path archiveFile;
private Path targetArchiveFile;
public void setArchiveFile(Path archiveFile) {
this.archiveFile = archiveFile;
}
public void setTargetArchiveFile(Path targetArchiveFile) {
this.targetArchiveFile = targetArchiveFile;
}
@Override
public void init() {
super.init();
Objects.requireNonNull(archiveFile);
Objects.requireNonNull(targetArchiveFile);
Objects.requireNonNull(previousModelVersion);
}
@Override
public void run() {
Path exploded = explodeArchive(archiveFile);
Path sources = getTemporaryPath().resolve("sources");
createForVersion(previousModelVersion, exploded, sources, DatabaseName.referential);
Path archive = getTemporaryPath().resolve("archive");
try {
generateTckScripts(exploded, sources, archive);
} catch (IOException e) {
throw new IllegalStateException(String.format("Can't generate tck scripts to: %s", archive), e);
}
try {
generateTckArchive(archive, targetArchiveFile);
} catch (IOException e) {
throw new IllegalStateException(String.format("Can't generate tck archive to: %s", targetArchiveFile), e);
}
}
private void generateTckScripts(Path exploded, Path sources, Path archive) throws IOException {
ObserveDataSourceConfigurationTopiaH2 configuration = createConfiguration("tck");
Path exportPath = archive.resolve(modelVersion.getVersion());
Path backupFile = getTemporaryPath().resolve("tck-backup.sql");
try (ToolkitTopiaApplicationContextSupport> applicationContext = createTopiaApplicationContext(configuration)) {
log.info(String.format("Load referential database for version %s", previousModelVersion));
applicationContext.executeSqlStatements(TopiaSqlScript.of(sources.resolve(previousModelVersion.getVersion()).resolve(DatabaseName.referential.name() + ".sql.gz")));
log.info(String.format("Migrate from version %s to %s", previousModelVersion, modelVersion));
applicationContext.migrate();
log.info(String.format("Load data script for version %s", modelVersion));
Path dataPath = exploded.resolve(modelVersion.getVersion()).resolve(DatabaseName.data.name() + ".sql");
applicationContext.executeSqlStatements(SqlScriptConsumer.builder(dataPath).batchSize(50).build());
log.info(String.format("Do backup to %s", backupFile));
applicationContext.backupSane(backupFile, false);
}
log.info(String.format("Generate tck scripts to %s", exportPath));
PersistenceScriptHelper.generateTckScripts(true, backupFile, exportPath);
}
private void generateTckArchive(Path archive, Path targetFile) throws IOException {
List files = Files.find(archive, 2, (path, basicFileAttributes) ->
Files.isRegularFile(path) && path.toFile().getName().endsWith(".sql")).collect(Collectors.toList());
log.info(String.format("Generate tck archive with %d script(s) to %s", files.size(), targetFile));
Files.deleteIfExists(targetFile);
if (Files.notExists(targetFile.getParent())) {
Files.createDirectories(targetFile.getParent());
}
Zips.compressFiles(targetFile.toFile(), archive.toFile(), files.stream().map(Path::toFile).collect(Collectors.toList()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy