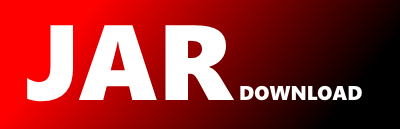
fr.ird.observe.services.ObserveServiceMainFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of toolkit-service Show documentation
Show all versions of toolkit-service Show documentation
ObServe Toolkit Service module
The newest version!
package fr.ird.observe.services;
/*
* #%L
* ObServe Toolkit :: Service
* %%
* Copyright (C) 2017 - 2021 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.dto.db.configuration.ObserveDataSourceConfiguration;
import fr.ird.observe.dto.db.configuration.ObserveDataSourceConnection;
import fr.ird.observe.services.service.ObserveService;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Objects;
import java.util.ServiceLoader;
import java.util.Set;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
/**
* Created on 16/08/15.
*
* @author Tony Chemit - [email protected]
*/
public class ObserveServiceMainFactory implements ObserveServiceFactory {
private static final Logger log = LogManager.getLogger(ObserveServiceMainFactory.class);
private static ExecutorService EXECUTOR_SERVICE;
private final Set delegateFactories;
public static ExecutorService getExecutorService() {
if (EXECUTOR_SERVICE == null) {
EXECUTOR_SERVICE = Executors.newFixedThreadPool(5);
log.info(String.format("Create executor service: %s", EXECUTOR_SERVICE));
}
return EXECUTOR_SERVICE;
}
public ObserveServiceMainFactory() {
log.info(String.format("ObServe Service factory initialization (%s).", this));
Set builder = new LinkedHashSet<>();
for (ObserveServiceFactory factory : ServiceLoader.load(ObserveServiceFactory.class)) {
log.info(String.format("Discover service factory: %s", factory));
factory.setMainServiceFactory(this);
builder.add(factory);
}
if (builder.isEmpty()) {
throw new IllegalStateException("No service factory found.");
}
log.info(String.format("Discover %d service factories.", builder.size()));
this.delegateFactories = Collections.unmodifiableSet(builder);
log.info(String.format("ObServe Service factory is initialized (%s).", this));
}
@Override
public ObserveServiceFactory getMainServiceFactory() {
return this;
}
@Override
public void setMainServiceFactory(ObserveServiceFactory mainServiceFactory) {
throw new IllegalStateException("You can't set main factory inside the main factory");
}
@Override
public boolean accept(ObserveDataSourceConfiguration dataSourceConfiguration, Class serviceType) {
getFactory(dataSourceConfiguration, serviceType);
return true;
}
@Override
public boolean accept(ObserveDataSourceConnection dataSourceConnection, Class serviceType) {
getFactory(dataSourceConnection, serviceType);
return true;
}
@Override
public S newService(ObserveServiceInitializer observeServiceInitializer, Class serviceType) {
Objects.requireNonNull(observeServiceInitializer, "observeServiceInitializerContext can't be null.");
Objects.requireNonNull(serviceType, "serviceType can't be null.");
ObserveServiceFactory factory;
if (observeServiceInitializer.withConnection()) {
factory = getFactory(observeServiceInitializer.getConnection(), serviceType);
} else if (observeServiceInitializer.withConfiguration()) {
factory = getFactory(observeServiceInitializer.getConfiguration(), serviceType);
} else {
throw new IllegalStateException("No dataSourceConnection, nor dataSourceConfiguration given.");
}
Objects.requireNonNull(factory, "factory can't be null.");
log.trace(String.format("Using factory: %s", factory));
S service = factory.newService(observeServiceInitializer, serviceType);
log.debug(String.format("New service created: %s", service));
return service;
}
@Override
public void close() {
for (ObserveServiceFactory delegateFactory : delegateFactories) {
try {
delegateFactory.close();
} catch (Exception e) {
log.error(String.format("Could not close factory: %s", delegateFactory), e);
}
}
if (EXECUTOR_SERVICE != null) {
log.info(String.format("Close executor service: %s", EXECUTOR_SERVICE));
EXECUTOR_SERVICE.shutdown();
EXECUTOR_SERVICE = null;
}
}
private ObserveServiceFactory getFactory(ObserveDataSourceConfiguration dataSourceConfiguration, Class serviceType) {
Objects.requireNonNull(dataSourceConfiguration);
Objects.requireNonNull(serviceType);
return delegateFactories.stream()
.filter(f -> f.accept(dataSourceConfiguration, serviceType))
.findFirst().orElseThrow(() -> new IllegalStateException(String.format("No factory found for dataSourceConfiguration: %s and serviceType: %s", dataSourceConfiguration, serviceType.getName())));
}
private ObserveServiceFactory getFactory(ObserveDataSourceConnection dataSourceConnection, Class serviceType) {
Objects.requireNonNull(dataSourceConnection);
Objects.requireNonNull(serviceType);
return delegateFactories.stream()
.filter(f -> f.accept(dataSourceConnection, serviceType))
.findFirst().orElseThrow(() -> new IllegalStateException(String.format("No factory found for dataSourceConnection: %s and serviceType: %s", dataSourceConnection, serviceType.getName())));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy