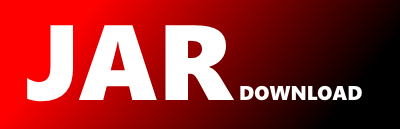
fr.ird.observe.services.service.referential.ObserveReferentialCache Maven / Gradle / Ivy
Show all versions of toolkit-service Show documentation
package fr.ird.observe.services.service.referential;
/*-
* #%L
* ObServe Toolkit :: Service
* %%
* Copyright (C) 2017 - 2021 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.dto.BusinessDto;
import fr.ird.observe.dto.form.FormDefinition;
import fr.ird.observe.dto.reference.ReferentialDtoReference;
import fr.ird.observe.dto.reference.ReferentialDtoReferenceSet;
import fr.ird.observe.dto.referential.ReferentialDto;
import fr.ird.observe.spi.decoration.DecoratorService;
import fr.ird.observe.spi.module.BusinessProject;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.io.Closeable;
import java.io.Serializable;
import java.util.Comparator;
import java.util.Date;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.TreeMap;
/**
* Un cache de référentiels.
*
* Created on 10/11/15.
*
* @author Tony Chemit - [email protected]
*/
public class ObserveReferentialCache implements Closeable, Serializable {
private static final long serialVersionUID = 1L;
private static final Logger log = LogManager.getLogger(ObserveReferentialCache.class);
private final Map, ReferentialDtoReferenceSet>> cache;
private final BusinessProject businessProject;
private final DecoratorService decoratorService;
public ObserveReferentialCache(BusinessProject businessProject, DecoratorService decoratorService) {
this.businessProject = Objects.requireNonNull(businessProject);
this.decoratorService = decoratorService;
this.cache = new TreeMap<>(Comparator.comparing(Object::toString));
}
public ReferentialDtoReferenceSet getReferentialReferenceSet(ReferentialService referentialService, Class type) {
@SuppressWarnings("unchecked") ReferentialDtoReferenceSet referenceSetDto = (ReferentialDtoReferenceSet) cache.get(type);
Date lastUpdate = null;
if (referenceSetDto != null) {
lastUpdate = referenceSetDto.getLastUpdate();
}
ReferentialDtoReferenceSet lastReferenceSetDto = referentialService.getReferenceSet(type, lastUpdate);
if (lastReferenceSetDto != null) {
cache.put(type, lastReferenceSetDto);
referenceSetDto = lastReferenceSetDto;
}
return referenceSetDto;
}
public Map, ReferentialDtoReferenceSet>> loadReferenceSets(ReferentialService referentialService, Class dtoType) {
Map, ReferentialDtoReferenceSet>> result = new LinkedHashMap<>();
if (dtoType != null) {
Optional> optionalRequestDefinition = businessProject.getOptionalFormDefinition(dtoType);
if (optionalRequestDefinition.isEmpty()) {
return result;
}
FormDefinition formDefinition = optionalRequestDefinition.get();
log.info(String.format("Loading %d referential dependencies for: %s", formDefinition.getProperties().size(), dtoType.getName()));
Map, Date> lastUpdateDates = getLastUpdateDates(formDefinition);
ReferenceSetsRequest request = new ReferenceSetsRequest<>();
request.setDtoType(dtoType);
request.setLastUpdateDates(lastUpdateDates);
Set> referenceSetResult = referentialService.getReferentialReferenceSets(request);
for (ReferentialDtoReferenceSet> referentialReferenceSet : referenceSetResult) {
decoratorService.installDecorator(referentialReferenceSet);
cache.put(referentialReferenceSet.getType(), referentialReferenceSet);
}
for (Class extends ReferentialDtoReference> type : formDefinition.getPropertiesReferenceTypes()) {
ReferentialDtoReferenceSet> referentialReferenceSet = cache.get(type);
result.put(type, referentialReferenceSet);
}
}
return result;
}
/**
* Pour récupérer les dates de dernières mises à jour des ensembles de références utiliées par la requète.
*
* @param formDefinition la définition de requète
* @return le dictionnaire des dates de dernières mises à jour pour chaque ensemble de référentiels
*/
private Map, Date> getLastUpdateDates(FormDefinition> formDefinition) {
Map, Date> result = new LinkedHashMap<>();
for (Class extends ReferentialDto> type : formDefinition.getPropertiesTypes()) {
ReferentialDtoReferenceSet> referenceSetDto = cache.get(type);
if (referenceSetDto != null) {
Date lastUpdate = referenceSetDto.getLastUpdate();
result.put(type, lastUpdate);
}
}
return result;
}
@Override
public void close() {
cache.clear();
}
}