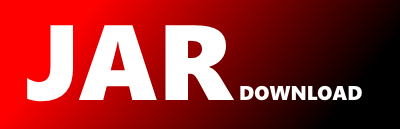
fr.ird.observe.services.service.referential.SynchronizeEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of toolkit-service Show documentation
Show all versions of toolkit-service Show documentation
ObServe Toolkit Service module
The newest version!
package fr.ird.observe.services.service.referential;
/*-
* #%L
* ObServe Toolkit :: Service
* %%
* Copyright (C) 2017 - 2021 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.dto.ToolkitIdLabel;
import fr.ird.observe.dto.referential.ReferentialDto;
import fr.ird.observe.spi.referential.synchro.BothSideSqlResult;
import fr.ird.observe.spi.referential.synchro.BothSidesSqlRequest;
import fr.ird.observe.spi.referential.synchro.OneSideSqlResult;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.nuiton.topia.persistence.script.TopiaSqlScript;
import java.nio.file.Path;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
/**
* Created on 15/07/2021.
*
* @author Tony Chemit - [email protected]
* @since 5.0.39
*/
public class SynchronizeEngine {
private static final Logger log = LogManager.getLogger(SynchronizeEngine.class);
/**
* Where to generate sql scripts.
*/
private final Path temporaryPath;
/**
* Left service.
*/
private final SynchronizeService leftService;
/**
* Right service.
*/
private final SynchronizeService rightService;
public SynchronizeEngine(Path temporaryPath, SynchronizeService leftService, SynchronizeService rightService) {
this.temporaryPath = Objects.requireNonNull(temporaryPath).resolve(getClass().getName());
this.leftService = Objects.requireNonNull(leftService);
this.rightService = Objects.requireNonNull(rightService);
}
public BothSideSqlResult produceSql(BothSidesSqlRequest sqlRequest) {
OneSideSqlResult leftResult = leftService.produceSqlResult(sqlRequest.getLeft());
OneSideSqlResult rightResult = rightService.produceSqlResult(sqlRequest.getRight());
return new BothSideSqlResult(leftResult, rightResult);
}
public void executeSql(BothSideSqlResult sqlResult) {
String filePrefix = String.format("SynchronizeEngine-%d-", System.nanoTime());
Path leftScriptPath = temporaryPath.resolve(filePrefix + "left.sql");
log.info(String.format("Generate left side script at: %s", leftScriptPath));
Optional leftScript = sqlResult.toLeftSqlScript(leftScriptPath);
leftScript.ifPresent(s -> executeSql(s, true));
Path rightScriptPath = temporaryPath.resolve(filePrefix + "right.sql");
log.info(String.format("Generate right side script at: %s", rightScriptPath));
Optional rightScript = sqlResult.toRightSqlScript(rightScriptPath);
rightScript.ifPresent(s -> executeSql(s, false));
}
public void executeSql(TopiaSqlScript sqlResult, boolean left) {
if (left) {
log.info("Execute left side script...");
leftService.applySql(sqlResult, SynchronizeService.ADVANCED_REFERENTIAL_SYNCHRONISATION);
} else {
log.info("Execute right side script...");
rightService.applySql(sqlResult, SynchronizeService.ADVANCED_REFERENTIAL_SYNCHRONISATION);
}
}
public Path getTemporaryPath() {
return temporaryPath;
}
public Set getLocalSourceReferentialToDelete(Class dtoType, Set blockingIdsToRemove) {
return leftService.getReferentialToDelete(dtoType, blockingIdsToRemove);
}
public Set filterIdsUsedInLocalSource(Class dtoType, Set idsToRemove) {
return leftService.filterIdsUsed(dtoType, idsToRemove);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy