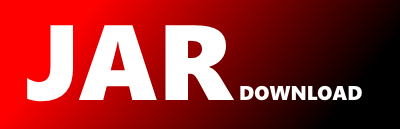
fr.ird.observe.toolkit.templates.entity.GroupBySqlHelper Maven / Gradle / Ivy
package fr.ird.observe.toolkit.templates.entity;
/*-
* #%L
* Toolkit :: Templates
* %%
* Copyright (C) 2017 - 2024 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.dto.data.DataGroupByDefinition;
import org.nuiton.eugene.GeneratorUtil;
import org.nuiton.eugene.models.object.ObjectModel;
import org.nuiton.eugene.models.object.ObjectModelAttribute;
import org.nuiton.eugene.models.object.ObjectModelClass;
import org.nuiton.eugene.models.object.ObjectModelClassifier;
import org.nuiton.eugene.models.object.ObjectModelPackage;
import org.nuiton.topia.service.sql.metadata.TopiaMetadataEntity;
import org.nuiton.topia.service.sql.metadata.TopiaMetadataModel;
import org.nuiton.topia.templates.EntityHibernateMappingTransformer;
import org.nuiton.topia.templates.TopiaHibernateTagValues;
import org.nuiton.topia.templates.TopiaTemplateHelper;
import java.io.IOException;
import java.io.Writer;
import java.sql.Timestamp;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* Helper to generate group by sql request.
*
* Created on 10/05/2022.
*
* @author Tony Chemit - [email protected]
* @since 2.0.0
*/
public class GroupBySqlHelper {
protected final TopiaHibernateTagValues topiaHibernateTagValues;
private final TopiaMetadataModel metadataModel;
private final ObjectModel model;
protected final TopiaTemplateHelper templateHelper;
protected final EntityHibernateMappingTransformer generator;
public GroupBySqlHelper(TopiaHibernateTagValues topiaHibernateTagValues, TopiaMetadataModel metadataModel, ObjectModel model, TopiaTemplateHelper templateHelper, EntityHibernateMappingTransformer generator) {
this.topiaHibernateTagValues = topiaHibernateTagValues;
this.metadataModel = metadataModel;
this.model = model;
this.templateHelper = templateHelper;
this.generator = generator;
}
public void addRootGetMultipleParentEntity(Writer output, ObjectModelPackage aPackage, ObjectModelClass objectModelClass, String input, TopiaMetadataEntity table, ObjectModelAttribute attribute, String entityProperty, String property, String groupByPredicate, String groupBySecond) throws IOException {
ObjectModelClassifier classifier = attribute.getClassifier();
TopiaMetadataEntity parentTable = classifier == null ? null : metadataModel.getEntity(templateHelper.getEntityEnumLiteralName(classifier));
if (parentTable == null) {
addRootQuantitativeMultipleParentEntity(output, aPackage, objectModelClass, input, table, attribute, entityProperty, property);
} else {
if (groupByPredicate != null) {
addQualitativeRootGetMultipleParentEntityWithPredicate(output, input, table, entityProperty, property, groupByPredicate, parentTable);
} else if (groupBySecond != null) {
addQualitativeRootGetMultipleParentEntityWithSecond(output, input, table, attribute, entityProperty, property, groupBySecond, parentTable);
} else {
addQualitativeRootGetMultipleParentEntity(output, input, table, entityProperty, property, parentTable);
}
}
}
protected void addQualitativeRootGetMultipleParentEntityWithSecond(Writer output,
String input,
TopiaMetadataEntity table,
ObjectModelAttribute attribute,
String entityProperty,
String property,
String groupBySecond,
TopiaMetadataEntity parentTable) throws IOException {
String propertyWithSecond = property + "." + groupBySecond;
String countOneQueryName = getCountOneQueryName(input, propertyWithSecond);
String countNullQueryName = getCountNullQueryName(input, propertyWithSecond);
String countAllQueryName = getCountAllQueryName(input, propertyWithSecond);
ObjectModelClassifier classifier = attribute.getClassifier();
ObjectModelAttribute secondClassifierAttribute = classifier.getAttribute(groupBySecond);
TopiaMetadataEntity secondParentTable = metadataModel.getEntity(templateHelper.getEntityEnumLiteralName(secondClassifierAttribute.getClassifier()));
String secondEntityProperty = templateHelper.getDbName(secondClassifierAttribute);
String countAllNoEmptyQueryName = getCountAllNoEmptyQueryName(countAllQueryName);
String countAllNoEmptyNoDisabledQueryName = getCountAllNoEmptyNoDisabledQueryName(countAllNoEmptyQueryName);
String countAllNoDisabledQueryName = getCountAllNoEmptyNoDisabledQueryName(countAllQueryName);
String fromRightJoin = String.format("FROM %1$s e RIGHT JOIN %2$s p ON e.%3$s = p.topiaId",
table.getSchemaAndTableName(),
parentTable.getSchemaAndTableName(),
entityProperty);
String fromLeftJoin = String.format("FROM %1$s e LEFT JOIN %2$s p ON e.%3$s = p.topiaId",
table.getSchemaAndTableName(),
parentTable.getSchemaAndTableName(),
entityProperty);
String rightSecondJoin = String.format("RIGHT JOIN %1$s f ON p.%2$s = f.topiaId",
secondParentTable.getSchemaAndTableName(),
secondEntityProperty);
String leftSecondJoin = String.format("LEFT JOIN %1$s f ON p.%2$s = f.topiaId",
secondParentTable.getSchemaAndTableName(),
secondEntityProperty);
{
String query = String.format("SELECT f.topiaId, COUNT(e.topiaId) %1$s %2$s GROUP BY f.topiaId ORDER BY f.topiaId;",
fromRightJoin,
rightSecondJoin);
boolean added = addSqlQuery(output, countAllQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllQueryName));
}
}
{
String query = String.format("SELECT f.topiaId, COUNT(e.topiaId) %1$s %2$s WHERE f.status = 1 GROUP BY f.topiaId ORDER BY f.topiaId;",
fromRightJoin,
rightSecondJoin);
boolean added = addSqlQuery(output, countAllNoDisabledQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoDisabledQueryName));
}
}
{
String query = String.format("SELECT p.%2$s, COUNT(e.topiaId) %1$s WHERE p.%2$s IS NOT NULL GROUP BY p.%2$s ORDER BY p.%2$s;",
fromLeftJoin,
secondEntityProperty);
boolean added = addSqlQuery(output, countAllNoEmptyQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoEmptyQueryName));
}
}
{
String query = String.format("SELECT f.topiaId, COUNT(e.topiaId) %1$s %2$s WHERE f.status = 1 GROUP BY f.topiaId ORDER BY f.topiaId;",
fromLeftJoin,
leftSecondJoin);
boolean added = addSqlQuery(output, countAllNoEmptyNoDisabledQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoEmptyNoDisabledQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) %1$s WHERE p.%2$s = ?;",
fromLeftJoin,
secondEntityProperty);
boolean added = addSqlQuery(output, countOneQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countOneQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) %1$s WHERE p.%2$s IS NULL;",
fromLeftJoin,
secondEntityProperty);
boolean added = addSqlQuery(output, countNullQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countNullQueryName));
}
}
}
protected void addQualitativeRootGetMultipleParentEntity(Writer output,
String input,
TopiaMetadataEntity table,
String entityProperty,
String property,
TopiaMetadataEntity parentTable) throws IOException {
String countOneQueryName = getCountOneQueryName(input, property);
String countNullQueryName = getCountNullQueryName(input, property);
String countAllQueryName = getCountAllQueryName(input, property);
String countAllNoEmptyQueryName = getCountAllNoEmptyQueryName(countAllQueryName);
String countAllNoEmptyNoDisabledQueryName = getCountAllNoEmptyNoDisabledQueryName(countAllNoEmptyQueryName);
String countAllNoDisabledQueryName = getCountAllNoEmptyNoDisabledQueryName(countAllQueryName);
String groupByExtraWithDisabled = "";
String groupByExtraNoDisabled = "WHERE p.status = 1 ";
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e RIGHT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraWithDisabled);
boolean added = addSqlQuery(output, countAllQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllQueryName));
}
}
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e RIGHT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraNoDisabled);
boolean added = addSqlQuery(output, countAllNoDisabledQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoDisabledQueryName));
}
}
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e LEFT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraWithDisabled);
boolean added = addSqlQuery(output, countAllNoEmptyQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoEmptyQueryName));
}
}
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e LEFT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraNoDisabled);
boolean added = addSqlQuery(output, countAllNoEmptyNoDisabledQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoEmptyNoDisabledQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE e.%3$s = ?;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countOneQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countOneQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE e.%3$s IS NULL;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countNullQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countNullQueryName));
}
}
addUpdateQuery(output, input, table, entityProperty, property, parentTable);
}
protected void addUpdateQuery(Writer output,
String input,
TopiaMetadataEntity table,
String entityProperty,
String property,
TopiaMetadataEntity parentTable) throws IOException {
String updateQueryName = getUpdateQueryName(input, property);
String query = String.format("UPDATE %1$s.%2$s SET %3$s = ?, topiaVersion = topiaVersion + 1, lastUpdateDate = ? WHERE topiaId = ?;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
Map parameters = new LinkedHashMap<>();
parameters.put("property", String.class.getName());
parameters.put("lastUpdateDate", Timestamp.class.getName());
parameters.put("id", String.class.getName());
boolean added = generator.addSqlQuery(output,
updateQueryName,
query,
" read-only=\"true\"",
null,
parameters);
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", updateQueryName));
}
}
protected void addQualitativeRootGetMultipleParentEntityWithPredicate(Writer output, String input, TopiaMetadataEntity table, String entityProperty, String property, String groupByPredicate, TopiaMetadataEntity parentTable) throws IOException {
String countOneQueryName = getCountOneQueryName(input, property);
String countNullQueryName = getCountNullQueryName(input, property);
String countAllQueryName = getCountAllQueryName(input, property);
String countAllNoEmptyQueryName = getCountAllNoEmptyQueryName(countAllQueryName);
String countAllNoEmptyNoDisabledQueryName = getCountAllNoEmptyNoDisabledQueryName(countAllNoEmptyQueryName);
String countAllNoDisabledQueryName = getCountAllNoEmptyNoDisabledQueryName(countAllQueryName);
String groupByExtraWithDisabled = "WHERE " + groupByPredicate + " ";
String groupByExtraNoDisabled = "WHERE p.status = 1 " + (("AND " + groupByPredicate)) + " ";
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e RIGHT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraWithDisabled);
boolean added = addSqlQuery(output, countAllQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllQueryName));
}
}
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e RIGHT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraNoDisabled);
boolean added = addSqlQuery(output, countAllNoDisabledQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoDisabledQueryName));
}
}
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e LEFT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraWithDisabled);
boolean added = addSqlQuery(output, countAllNoEmptyQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoEmptyQueryName));
}
}
{
String query = String.format("SELECT p.topiaId, COUNT(e.topiaId) FROM %1$s.%2$s e LEFT JOIN %3$s.%4$s p ON e.%5$s = p.topiaId %6$sGROUP BY p.topiaId ORDER BY p.topiaId;", table.getDbSchemaName(), table.getDbTableName(), parentTable.getDbSchemaName(), parentTable.getDbTableName(), entityProperty, groupByExtraNoDisabled);
boolean added = addSqlQuery(output, countAllNoEmptyNoDisabledQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllNoEmptyNoDisabledQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE e.%3$s = ?;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countOneQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countOneQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE e.%3$s IS NULL;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countNullQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countNullQueryName));
}
}
addUpdateQuery(output, input, table, entityProperty, property, parentTable);
}
protected void addRootQuantitativeMultipleParentEntity(Writer output, ObjectModelPackage aPackage, ObjectModelClass objectModelClass, String input, TopiaMetadataEntity table, ObjectModelAttribute attribute, String entityProperty, String property) throws IOException {
String countOneQueryName = getCountOneQueryName(input, property);
String countNullQueryName = getCountNullQueryName(input, property);
String countAllQueryName = getCountAllQueryName(input, property);
String attributeType = GeneratorUtil.getSimpleName(attribute.getType());
String temporalType = null;
if ("Date".equals(attributeType)) {
temporalType = topiaHibernateTagValues.getHibernateAttributeType(attribute, objectModelClass, aPackage, model);
if (temporalType == null) {
temporalType = "timestamp";
}
}
String nullQuery = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE e.%3$s IS NULL;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
if (temporalType == null) {
// simple quantitative groupBy
{
String query = String.format("SELECT e.%1$s || '', COUNT(e.topiaId) FROM %2$s.%3$s e GROUP BY e.%1$s ORDER BY e.%1$s;", entityProperty, table.getDbSchemaName(), table.getDbTableName());
boolean added = addSqlQuery(output, countAllQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE e.%3$s || '' = ?;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countOneQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countOneQueryName));
}
}
{
boolean added = addSqlQuery(output, countNullQueryName, nullQuery, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countNullQueryName));
}
}
} else {
// temporal quantitative groupBy
{
String query = String.format("SELECT TO_CHAR(e.%1$s, 'YYYY-MM-DD'), COUNT(e.topiaId) FROM %2$s.%3$s e GROUP BY TO_CHAR(e.%1$s, 'YYYY-MM-DD') ORDER BY TO_CHAR(e.%1$s, 'YYYY-MM-DD');", entityProperty, table.getDbSchemaName(), table.getDbTableName());
boolean added = addSqlQuery(output, countAllQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllQueryName));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE e.%3$s || '' = ?;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countOneQueryName, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countOneQueryName));
}
}
{
boolean added = addSqlQuery(output, countNullQueryName, nullQuery, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countNullQueryName));
}
}
{ // Add by Year
String countAllQueryNameByYear = countAllQueryName + "-year";
String countOneQueryNameByYear = countOneQueryName + "-year";
String countNullQueryNameByYear = countNullQueryName + "-year";
{
String query = String.format("SELECT TO_CHAR(e.%1$s, 'YYYY'), COUNT(e.topiaId) FROM %2$s.%3$s e GROUP BY TO_CHAR(e.%1$s, 'YYYY') ORDER BY TO_CHAR(e.%1$s, 'YYYY');", entityProperty, table.getDbSchemaName(), table.getDbTableName());
boolean added = addSqlQuery(output, countAllQueryNameByYear, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllQueryNameByYear));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE TO_CHAR(e.%3$s, 'YYYY') = ?;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countOneQueryNameByYear, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countOneQueryNameByYear));
}
}
{
boolean added = addSqlQuery(output, countNullQueryNameByYear, nullQuery, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countNullQueryNameByYear));
}
}
}
{
// Add by Month
String countAllQueryNameByMonth = countAllQueryName + "-month";
String countOneQueryNameByMonth = countOneQueryName + "-month";
String countNullQueryNameByMonth = countNullQueryName + "-month";
{
String query = String.format("SELECT TO_CHAR(e.%1$s, 'YYYY-MM'), COUNT(e.topiaId) FROM %2$s.%3$s e GROUP BY TO_CHAR(e.%1$s, 'YYYY-MM') ORDER BY TO_CHAR(e.%1$s, 'YYYY-MM');", entityProperty, table.getDbSchemaName(), table.getDbTableName());
boolean added = addSqlQuery(output, countAllQueryNameByMonth, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countAllQueryNameByMonth));
}
}
{
String query = String.format("SELECT COUNT(e.topiaId) FROM %1$s.%2$s e WHERE TO_CHAR(e.%3$s, 'YYYY-MM') = ?;", table.getDbSchemaName(), table.getDbTableName(), entityProperty);
boolean added = addSqlQuery(output, countOneQueryNameByMonth, query, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countOneQueryNameByMonth));
}
}
{
boolean added = addSqlQuery(output, countNullQueryNameByMonth, nullQuery, " read-only=\"true\"");
if (!added) {
throw new IllegalStateException(String.format("Can't add query: %s", countNullQueryNameByMonth));
}
}
}
}
}
protected boolean addSqlQuery(Writer output, String queryName, String queryCode, String extraParameters) throws IOException {
return generator.addSqlQuery(output, queryName, queryCode, extraParameters);
}
private String getUpdateQueryName(String input, String propertyWithSecond) {
return input + "::groupBy::" + propertyWithSecond + "::update";
}
private String getCountAllQueryName(String input, String propertyWithSecond) {
return input + "::groupBy::" + propertyWithSecond + "::count::all";
}
private String getCountOneQueryName(String input, String propertyWithSecond) {
return input + "::groupBy::" + propertyWithSecond + "::count::one";
}
private String getCountNullQueryName(String input, String property) {
return input + "::groupBy::" + property + "::count::null";
}
private String getCountAllNoEmptyNoDisabledQueryName(String countAllNoEmptyQueryName) {
return countAllNoEmptyQueryName + DataGroupByDefinition.FLAVOR_NO_DISABLED;
}
private String getCountAllNoEmptyQueryName(String countAllQueryName) {
return countAllQueryName + DataGroupByDefinition.FLAVOR_NO_EMPTY;
}
}