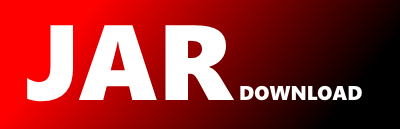
fr.ird.observe.toolkit.templates.entity.ReplaceReferentialScriptGenerator Maven / Gradle / Ivy
package fr.ird.observe.toolkit.templates.entity;
/*-
* #%L
* Toolkit :: Templates
* %%
* Copyright (C) 2017 - 2024 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import fr.ird.observe.spi.referential.SqlStatements;
import org.nuiton.topia.service.sql.metadata.TopiaMetadataAssociation;
import org.nuiton.topia.service.sql.metadata.TopiaMetadataComposition;
import org.nuiton.topia.service.sql.metadata.TopiaMetadataEntity;
import org.nuiton.topia.service.sql.metadata.TopiaMetadataModel;
import org.nuiton.topia.service.sql.script.ReplaceReferentialInDataScript;
import org.nuiton.topia.service.sql.script.ReplaceReferentialInReferentialScript;
import java.util.LinkedList;
import java.util.List;
import java.util.Objects;
import java.util.Set;
/**
* Generate replace script for a referential type.
*
* It will
*
* - replace only business data many-to-many associations
* - replace any many-to-one relations
*
*
* Created on 04/07/16.
*
* @author Tony Chemit - [email protected]
* @see ReplaceReferentialInDataScript
* @see ReplaceReferentialInReferentialScript
* @since 5.0
*/
public class ReplaceReferentialScriptGenerator {
/**
* To update a reference of a composition relation.
*/
public static final String COMPOSITION_UPDATE_STATEMENT = "UPDATE %1$s.%2$s SET %3$s = '%4$s', topiaVersion = topiaVersion + 1, lastUpdateDate = '%5$s'::timestamp WHERE %3$s = '%6$s';";
/**
* To update a reference of an association relation.
*/
private static final String ASSOCIATION_UPDATE_STATEMENT = "UPDATE %1$s.%2$s SET %3$s = '%4$s' WHERE %3$s = '%5$s' AND (SELECT COUNT(s.%3$s) FROM %1$s.%2$s s WHERE s.%3$s = '%4$s') = 0;";
/**
* All reverse relation many-to-one.
*/
private final Set compositions;
/**
* All reverse relation many-to-many.
*/
private final Set associations;
public ReplaceReferentialScriptGenerator(TopiaMetadataModel topiaMetadataModel, TopiaMetadataEntity metadataEntity) {
this.compositions = Objects.requireNonNull(topiaMetadataModel).getReverseCompositions(Objects.requireNonNull(metadataEntity));
this.associations = topiaMetadataModel.getReverseManyToManyAssociations(metadataEntity);
}
public List generateSql(String sourceId, String replacementId, String lastUpdateDate, boolean forData) {
List builder = new LinkedList<>();
for (TopiaMetadataComposition replacementStruct : compositions) {
if (replacementStruct.getOwner().getFullyQualifiedName().contains(".referential") && forData) {
continue;
}
if (!replacementStruct.getOwner().getFullyQualifiedName().contains(".referential") && !forData) {
continue;
}
String sql = generateCompositionUpdateStatement(replacementStruct, sourceId, replacementId, lastUpdateDate);
builder.add(sql);
}
for (TopiaMetadataAssociation replacementStruct : associations) {
if (replacementStruct.getOwner().getFullyQualifiedName().contains(".referential")) {
// do not update referential associations (see https://gitlab.com/ultreiaio/ird-observe/issues/1065)
continue;
}
if (!forData) {
continue;
}
String sql = generateAssociationUpdateStatement(replacementStruct, sourceId, replacementId);
builder.add(sql);
sql = generateAssociationDeleteStatement(replacementStruct, sourceId);
builder.add(sql);
}
return builder;
}
protected String generateCompositionUpdateStatement(TopiaMetadataComposition composition, String sourceId, String replacementId, String lastUpdateDate) {
return String.format(COMPOSITION_UPDATE_STATEMENT,
composition.getOwner().getDbSchemaName(),
composition.getTableName(),
composition.getTargetDbName(),
replacementId,
lastUpdateDate,
sourceId);
}
protected String generateAssociationUpdateStatement(TopiaMetadataAssociation association, String sourceId, String replacementId) {
return String.format(ASSOCIATION_UPDATE_STATEMENT,
association.getOwner().getDbSchemaName(),
association.getTableName(),
association.getTargetDbName(),
replacementId,
sourceId);
}
private String generateAssociationDeleteStatement(TopiaMetadataAssociation association, String sourceId) {
return String.format(SqlStatements.ASSOCIATION_DELETE_STATEMENT,
association.getOwner().getDbSchemaName(),
association.getTableName(),
association.getTargetDbName(),
sourceId).trim();
}
}