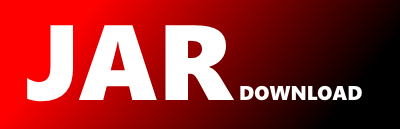
org.nuiton.topia.service.sql.metadata.TopiaMetadataModel Maven / Gradle / Ivy
package org.nuiton.topia.service.sql.metadata;
/*
* #%L
* Toolkit :: Templates
* %%
* Copyright (C) 2017 - 2024 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.TreeSet;
import java.util.concurrent.ExecutionException;
import java.util.stream.Stream;
/**
* Méta-modèle topia simplifié qui contient des informations utile pour des algorithmes générique sur les entités.
*
* Ce méta-modèle est juste un conteneur de méta-modèle d'entités.
*
* Created on 03/01/16.
*
* @author Tony Chemit - [email protected]
* @since 5.0
*/
@SuppressWarnings("NullableProblems")
public class TopiaMetadataModel implements Iterable {
/**
* Metadata for all entities of the model.
*/
protected final Map entities;
/**
* To get all compositions for each entity type.
*/
private final transient LoadingCache> compositions = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity source = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Map.Entry link : source.getManyToOneAssociations().entrySet()) {
TopiaMetadataEntity target = Objects.requireNonNull(getEntity(link.getValue()));
builder.add(new TopiaMetadataComposition(source, link.getKey(), target));
}
return builder;
}
});
/**
* To get all reverse compositions for each entity type.
*/
private final transient LoadingCache> reverseCompositions = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity target = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Iterator it = streamWithoutAbstract().iterator(); it.hasNext(); ) {
TopiaMetadataEntity source = it.next();
for (Map.Entry entry : source.getManyToOneAssociations().entrySet()) {
if (entry.getValue().equals(key)) {
builder.add(new TopiaMetadataComposition(source, entry.getKey(), target));
}
}
}
return builder;
}
});
/**
* To get all reverse many to many associations for each entity type.
*/
private final transient LoadingCache> reverseManyToManyAssociations = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity target = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Iterator it = streamWithoutAbstract().iterator(); it.hasNext(); ) {
TopiaMetadataEntity source = it.next();
for (Map.Entry entry : source.getManyToManyAssociations().entrySet()) {
if (entry.getValue().equals(key)) {
builder.add(new TopiaMetadataAssociation(source, entry.getKey(), target));
}
}
}
return builder;
}
});
/**
* To get all reverse one to many associations for each entity type.
*/
private final transient LoadingCache> reverseOneToManyAssociations = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity target = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Iterator it = streamWithoutAbstract().iterator(); it.hasNext(); ) {
TopiaMetadataEntity source = it.next();
for (Map.Entry entry : source.getOneToManyAssociations().entrySet()) {
if (entry.getValue().equals(key)) {
builder.add(new TopiaMetadataAssociation(source, entry.getKey(), target));
}
}
}
return builder;
}
});
/**
* To get all reverse one to one associations for each entity type.
*/
private final transient LoadingCache> reverseOneToOneAssociations = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity target = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Iterator it = streamWithoutAbstract().iterator(); it.hasNext(); ) {
TopiaMetadataEntity source = it.next();
for (Map.Entry entry : source.getOneToOneCompositions().entrySet()) {
if (entry.getValue().equals(key)) {
builder.add(new TopiaMetadataOneToOneComposition(source, entry.getKey(), target));
}
}
}
return builder;
}
});
/**
* To get all associations for each entity type.
*/
private final transient LoadingCache> associations = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity source = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Map.Entry link : source.getManyToManyAssociations().entrySet()) {
TopiaMetadataEntity target = Objects.requireNonNull(getEntity(link.getValue()));
builder.add(new TopiaMetadataAssociation(source, link.getKey(), target));
}
return builder;
}
});
/**
* To get all associations for each entity type.
*/
private final transient LoadingCache> simpleAssociations = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity source = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Map.Entry link : source.getManyAssociations().entrySet()) {
String propertyName = link.getKey();
String tableName = source.getBdManyAssociationTableName(propertyName);
String joinColumnName = source.getDbColumnName(tableName);
builder.add(new TopiaMetadataSimpleAssociation(source, tableName, propertyName, joinColumnName, link.getValue()));
}
return builder;
}
});
/**
* To get all reverse associations for each entity type.
*/
private final transient LoadingCache> reverseAssociations = CacheBuilder.newBuilder().build(new CacheLoader<>() {
@Override
public Set load(String key) {
TopiaMetadataEntity source = Objects.requireNonNull(getEntity(Objects.requireNonNull(key)));
Set builder = new LinkedHashSet<>();
for (Map.Entry link : source.getReversedAssociations().entrySet()) {
TopiaMetadataEntity target = Objects.requireNonNull(getEntity(link.getValue()));
builder.add(new TopiaMetadataReverseAssociation(source, link.getKey(), target));
}
return builder;
}
});
public TopiaMetadataModel(Map entities) {
this.entities = Objects.requireNonNull(entities);
}
public TopiaMetadataModel() {
this.entities = new LinkedHashMap<>();
}
public void applyInheritance() {
Set done = new TreeSet<>();
for (TopiaMetadataEntity metadataEntity : this) {
applyInheritance(metadataEntity, done);
}
}
public Collection getEntities() {
return entities.values();
}
public TopiaMetadataEntity getEntity(String type) {
return entities.get(type);
}
public Optional getOptionalEntity(String type) {
return Optional.ofNullable(getEntity(type));
}
public void accept(TopiaMetadataModelVisitor visitor) {
visitor.visitModelStart(this);
for (TopiaMetadataEntity entity : entities.values()) {
entity.accept(visitor, this);
}
visitor.visitModelEnd(this);
}
public Set getReverseCompositions(TopiaMetadataEntity type) {
return getReverseCompositions(Objects.requireNonNull(type).getType());
}
public Set getReverseCompositions(String type) {
try {
return reverseCompositions.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get reverse compositions for type: " + type, e);
}
}
public Set getCompositions(TopiaMetadataEntity type) {
return getCompositions(Objects.requireNonNull(type).getType());
}
public Set getCompositions(String type) {
try {
return compositions.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get compositions for type: " + type, e);
}
}
public Set getReverseManyToManyAssociations(TopiaMetadataEntity type) {
return getReverseManyToManyAssociations(Objects.requireNonNull(type).getType());
}
public Set getReverseManyToManyAssociations(String type) {
try {
return reverseManyToManyAssociations.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get reverse many to many associations for type: " + type, e);
}
}
public Set getReverseAssociations(TopiaMetadataEntity type) {
return getReverseAssociations(Objects.requireNonNull(type).getType());
}
public Set getReverseAssociations(String type) {
try {
return reverseAssociations.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get reverse associations for type: " + type, e);
}
}
public Set getReverseOneToManyAssociations(TopiaMetadataEntity type) {
return getReverseOneToManyAssociations(Objects.requireNonNull(type).getType());
}
public Set getReverseOneToManyAssociations(String type) {
try {
return reverseOneToManyAssociations.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get reverse one to many associations for type: " + type, e);
}
}
public Set getReverseOneToOneAssociations(TopiaMetadataEntity type) {
return getReverseOneToOneAssociations(Objects.requireNonNull(type).getType());
}
public Set getReverseOneToOneAssociations(String type) {
try {
return reverseOneToOneAssociations.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get reverse one to one associations for type: " + type, e);
}
}
public Set getAssociations(TopiaMetadataEntity type) {
return getAssociations(Objects.requireNonNull(type).getType());
}
public Set getSimpleAssociations(TopiaMetadataEntity type) {
return getSimpleAssociations(Objects.requireNonNull(type).getType());
}
public Set getSimpleAssociations(String type) {
try {
return simpleAssociations.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get simple associations for type: " + type, e);
}
}
public Set getAssociations(String type) {
try {
return associations.get(Objects.requireNonNull(type));
} catch (ExecutionException e) {
throw new RuntimeException("Can't get associations for type: " + type, e);
}
}
@Override
public Iterator iterator() {
return entities.values().iterator();
}
public Stream stream() {
return entities.values().stream();
}
public Stream streamWithoutAbstract() {
return stream().filter(e -> !e.isAbstract());
}
public Stream streamWithEntryPoint() {
return streamWithoutAbstract().filter(TopiaMetadataEntity::isEntryPoint);
}
public Stream streamWithStandalone() {
return streamWithoutAbstract().filter(TopiaMetadataEntity::isStandalone);
}
private void applyInheritance(TopiaMetadataEntity metadataEntity, Set done) {
if (!done.add(metadataEntity.getType())) {
return;
}
String parentName = metadataEntity.getParent();
boolean haveSuper = parentName != null;
if (haveSuper) {
Optional optionalEntity = getOptionalEntity(parentName);
if (optionalEntity.isPresent()) {
TopiaMetadataEntity parentMetadataEntity = optionalEntity.get();
applyInheritance(parentMetadataEntity, done);
metadataEntity.putAll(parentMetadataEntity);
}
}
}
}