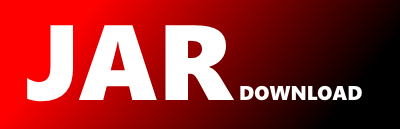
sim.app.celegans.Cells Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mason Show documentation
Show all versions of mason Show documentation
MASON is a fast discrete-event multiagent simulation library core in Java, designed to be the foundation for large custom-purpose Java simulations, and also to provide more than enough functionality for many lightweight simulation needs. MASON contains both a model library and an optional suite of visualization tools in 2D and 3D.
The newest version!
/*
Copyright 2006 by Sean Luke and George Mason University
Licensed under the Academic Free License version 3.0
See the file "LICENSE" for more information
*/
package sim.app.celegans;
import java.util.*;
import java.io.*;
import java.util.zip.*;
import sim.util.*;
/** Cells is the database of cell information in the program. It loads and parses
cells from the data file, creating five database items: a cell dictionary (looked
up by the cell's official name), a group dictionary, an expression pattern
dictionary, a cell fate dictionary, and a ArrayList of lineage roots. Ordinarily,
P0' would be the only root in a lineage tree, but the data is flawed and in fact
several cells don't have parents and get put in as roots. Hence the need for
a vector rather than a single root.
Most of Cells' items are public; there's little real encapsulation here, in the
name of getting the job done.
*/
public class Cells extends Object
{
private static final long serialVersionUID = 1;
public HashMap cell_dictionary;
// public HashMap group_dictionary;
// public HashMap pattern_dictionary;
// public HashMap fate_dictionary;
public ArrayList roots; // Cells with no parent. There should only be one, but what the heck.
int num_processed_cells;
public Cell P0;
public Cells()
{
cell_dictionary = new HashMap(300);
// group_dictionary = new HashMap();
// pattern_dictionary = new HashMap();
// fate_dictionary = new HashMap();
roots = new ArrayList();
num_processed_cells=0;
try
{
Reader r = new InputStreamReader(new GZIPInputStream(Cells.class.getResourceAsStream("cells.ace4.gz")));
readCells(r);
r.close();
postProcess();
}
catch (IOException e) { throw new RuntimeException(e); }
}
/** PostProcesses the cells after their basic information has been loaded and
parsed from the input file. A lot of derived information is formed
during postProcess(), as you can see from the method code. */
public void postProcess()
{
Iterator cells;
/* Assign Types; report everyone who doesn't have a parent (there should only be one: P0) */
System.out.println("-----Assigning Cell Types, and Parents to equivalent-origin cells");
cells= cell_dictionary.values().iterator();
while(cells.hasNext())
{
Cell cell=(Cell)cells.next();
if (cell.official_name.equals("P0"))
P0 = cell;
if (cell.parent==null && !cell.official_name.equals("P0") && cell.num_equivalence_origin==0)
System.out.println("Whoa! This ain't right: " +cell.official_name+ "Has no parent.");
if (cell.parent==null && cell.num_equivalence_origin==0) roots.add(cell);
if (cell.num_equivalence_origin!=0)
{
cell.type=Cell.cell_type_postembryonic_dual_origin;
cell.parent=cell.equivalence_origin[0]; // determining equivalence junk
if (cell.parent.equivalence_fate[0]!=cell) // determining equivalence junk
cell.parent.equivalence_fate[0].parent=cell.equivalence_origin[1];
else cell.parent.equivalence_fate[1].parent=cell.equivalence_origin[1];
}
else if (cell.official_name.equals("P0") ||
cell.official_name.equals("P1'") ||
cell.official_name.equals("P2'") ||
cell.official_name.equals("AB") ||
cell.official_name.equals("P3'") ||
cell.official_name.equals("P4'"))
cell.type=Cell.cell_type_preembryonic_unknown_position;
else if (cell.official_name.equals("Z3") ||
cell.official_name.equals("Z2"))
cell.type=Cell.cell_type_postembryonic_unknown_position;
else if (cell.birthday=size) // Bad Index, nothing left
break;
if (newpos==pos) v.add("");
else v.add(s.substring(pos,newpos));
pos=newpos+1;
}
if (pos!=size)
{
// Grab last one if any
v.add(s.substring(pos,size)); // is that right?
}
return 1;
}
/** processCellLine is used by readCells() to process a single cell line from the input stream. As you can tell, this is a big method, doing a lot of processing.*/
public Cell processCellLine(Cell current, ArrayList v) throws NumberFormatException
{
if (v==null) return current;
if (v.size()==0) return current;
String title=(String)v.get(0);
if (title.equals("Cell"))
{
current=fetchCell((String)v.get(2));
if (num_processed_cells%100 == 0)
System.out.println((num_processed_cells+1) + ": " + current.official_name);
num_processed_cells++;
}
else if (current==null) return current;
else
{
// here we go!
if (title.equals("Parent"))
{
current.parent=fetchCell((String)v.get(1));
if (current.parent.num_children>=2)
/* We've got a problem.
Extend the array for this
exceptional situation */
{
Cell tmp[] =
new Cell[current.parent.num_children+1];
/* Yeah, yeah, it's a linear increase,
which is O(n^2) in the worst case,
but this is an exceptional situation
hopefully. */
System.arraycopy(current.parent.daughters,0,tmp,0,current.parent.num_children);
current.parent.daughters=tmp;
System.out.print(current.parent.official_name + " has more than 2 children: ");
for(int zz=0;zz
© 2015 - 2025 Weber Informatics LLC | Privacy Policy