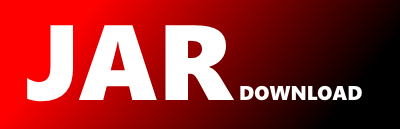
sim.app.mav.Mav Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mason Show documentation
Show all versions of mason Show documentation
MASON is a fast discrete-event multiagent simulation library core in Java, designed to be the foundation for large custom-purpose Java simulations, and also to provide more than enough functionality for many lightweight simulation needs. MASON contains both a model library and an optional suite of visualization tools in 2D and 3D.
The newest version!
/*
Copyright 2006 by Sean Luke and George Mason University
Licensed under the Academic Free License version 3.0
See the file "LICENSE" for more information
*/
package sim.app.mav;
import sim.portrayal.*;
import sim.engine.*;
import sim.util.*;
// we extend OvalPortrayal2D to steal its hitObjects() code -- but
// we override the draw(...) code to draw our own oval with a little line...
public /*strictfp*/ class Mav implements Steppable, Oriented2D
{
private static final long serialVersionUID = 1;
final static double[] theta = new double[/* 8 */]
{
0*(/*Strict*/Math.PI/180),
45*(/*Strict*/Math.PI/180),
90*(/*Strict*/Math.PI/180),
135*(/*Strict*/Math.PI/180),
180*(/*Strict*/Math.PI/180),
225*(/*Strict*/Math.PI/180),
270*(/*Strict*/Math.PI/180),
315*(/*Strict*/Math.PI/180)
};
final static double[] xd = new double[/* 8 */]
{
/*Strict*/Math.cos(theta[0]),
/*Strict*/Math.cos(theta[1]),
/*Strict*/Math.cos(theta[2]),
/*Strict*/Math.cos(theta[3]),
/*Strict*/Math.cos(theta[4]),
/*Strict*/Math.cos(theta[5]),
/*Strict*/Math.cos(theta[6]),
/*Strict*/Math.cos(theta[7]),
};
final static double[] yd = new double[/* 8 */]
{
/*Strict*/Math.sin(theta[0]),
/*Strict*/Math.sin(theta[1]),
/*Strict*/Math.sin(theta[2]),
/*Strict*/Math.sin(theta[3]),
/*Strict*/Math.sin(theta[4]),
/*Strict*/Math.sin(theta[5]),
/*Strict*/Math.sin(theta[6]),
/*Strict*/Math.sin(theta[7]),
};
public int orientation = 0;
public double x;
public double y;
public double orientation2D() { return theta[orientation]; }
public Mav(int orientation, double x, double y)
{
this.orientation = orientation; this.x = x; this.y = y;
}
public void step(SimState state)
{
final MavDemo mavdemo = (MavDemo)state;
orientation += mavdemo.random.nextInt(3) - 1;
if (orientation > 7) orientation = 0;
if (orientation < 0) orientation = 7;
x += xd[orientation];
y += yd[orientation];
if (x >= mavdemo.width) x = mavdemo.width - 1;
else if (x < 0) x = 0;
if (y >= mavdemo.height) y = mavdemo.height - 1;
else if (y < 0) y = 0;
mavdemo.mavs.setObjectLocation(this,new Double2D(x,y));
act(nearbyMAVs(mavdemo), currentSurface(mavdemo));
}
public void act(double[] sensorReading, int currentSurface)
{
if (currentSurface == 100) System.out.println("Acting");
}
double[] proximitySensors = new double[8]; // all squared values
/** Re-uses the double[], so don't hang onto it */
public double[] nearbyMAVs(MavDemo mavdemo)
{
for(int i=0;i<8;i++) proximitySensors[i] = Double.MAX_VALUE;
final double d = mavdemo.sensorRangeDistance * mavdemo.sensorRangeDistance;
final Bag nearbyMavs = mavdemo.mavs.getNeighborsWithinDistance(new Double2D(x,y),16,false,false);
for(int i=0;i 0) // right side
{
if (yy > 0) // quadrant 1
{
if (xx > yy) o = 0;
else o = 1;
}
else // quadrant 4
{
if (xx > -yy) o = 7;
else o = 6;
}
}
else // left side
{
if (yy > 0) // quadrant 2
{
if (-xx > yy) o = 3;
else o = 2;
}
else // quadrant 3
{
if (-xx > -yy) o = 4;
else o = 5;
}
} // hope I got that right!
}
// now rotate to be relative to MAV's orientation
o += orientation;
if (o >= 8) o = o % 8;
return o;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy