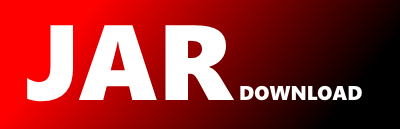
sim.app.wcss.tutorial12.Students Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mason Show documentation
Show all versions of mason Show documentation
MASON is a fast discrete-event multiagent simulation library core in Java, designed to be the foundation for large custom-purpose Java simulations, and also to provide more than enough functionality for many lightweight simulation needs. MASON contains both a model library and an optional suite of visualization tools in 2D and 3D.
The newest version!
/*
Copyright 2006 by Sean Luke and George Mason University
Licensed under the Academic Free License version 3.0
See the file "LICENSE" for more information
*/
package sim.app.wcss.tutorial12;
import sim.engine.*;
import sim.util.*;
import sim.field.continuous.*;
import sim.field.network.*;
public class Students extends SimState
{
private static final long serialVersionUID = 1;
public Continuous2D yard = new Continuous2D(1.0,100,100);
public double TEMPERING_CUT_DOWN = 0.99;
public double TEMPERING_INITIAL_RANDOM_MULTIPLIER = 10.0;
public boolean tempering = true;
public boolean isTempering() { return tempering; }
public void setTempering(boolean val) { tempering = val; }
public int numStudents = 50;
double forceToSchoolMultiplier = 0.01;
double randomMultiplier = 0.1;
public int getNumStudents() { return numStudents; }
public void setNumStudents(int val) { if (val > 0) numStudents = val; }
public double getForceToSchoolMultiplier() { return forceToSchoolMultiplier; }
public void setForceToSchoolMultiplier(double val) { if (forceToSchoolMultiplier >= 0.0) forceToSchoolMultiplier = val; }
public double getRandomMultiplier() { return randomMultiplier; }
public void setRandomMultiplier(double val) { if (randomMultiplier >= 0.0) randomMultiplier = val; }
public Object domRandomMultiplier() { return new sim.util.Interval(0.0, 100.0); }
public double[] getAgitationDistribution()
{
Bag students = buddies.getAllNodes();
double[] distro = new double[students.numObjs];
int len = students.size();
for(int i = 0; i < len; i++)
distro[i] = ((Student)(students.get(i))).getAgitation();
return distro;
}
public Network buddies = new Network(false);
public Students(long seed)
{
super(seed);
}
public void start()
{
super.start();
// add the tempering agent
if (tempering)
{
randomMultiplier = TEMPERING_INITIAL_RANDOM_MULTIPLIER;
schedule.scheduleRepeating(schedule.EPOCH, 1, new Steppable()
{ public void step(SimState state) { if (tempering) randomMultiplier *= TEMPERING_CUT_DOWN; } });
}
// clear the yard
yard.clear();
// clear the buddies
buddies.clear();
// add some students to the yard
for(int i = 0; i < numStudents; i++)
{
Student student = new Student();
yard.setObjectLocation(student,
new Double2D(yard.getWidth() * 0.5 + random.nextDouble() - 0.5,
yard.getHeight() * 0.5 + random.nextDouble() - 0.5));
buddies.addNode(student);
schedule.scheduleRepeating(student);
}
// define like/dislike relationships
Bag students = buddies.getAllNodes();
for(int i = 0; i < students.size(); i++)
{
Object student = students.get(i);
// who does he like?
Object studentB = null;
do
{
studentB = students.get(random.nextInt(students.numObjs));
} while (student == studentB);
double buddiness = random.nextDouble();
buddies.addEdge(student, studentB, new Double(buddiness));
// who does he dislike?
do
{
studentB = students.get(random.nextInt(students.numObjs));
} while (student == studentB);
buddiness = random.nextDouble();
buddies.addEdge(student, studentB, new Double( -buddiness));
}
}
public static void main(String[] args)
{
doLoop(Students.class, args);
System.exit(0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy