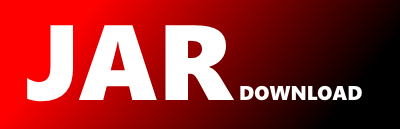
sim.portrayal3d.grid.ValueGridPortrayal3D Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mason Show documentation
Show all versions of mason Show documentation
MASON is a fast discrete-event multiagent simulation library core in Java, designed to be the foundation for large custom-purpose Java simulations, and also to provide more than enough functionality for many lightweight simulation needs. MASON contains both a model library and an optional suite of visualization tools in 2D and 3D.
The newest version!
/*
Copyright 2006 by Sean Luke and George Mason University
Licensed under the Academic Free License version 3.0
See the file "LICENSE" for more information
*/
package sim.portrayal3d.grid;
import sim.field.grid.*;
import sim.portrayal.*;
import sim.portrayal3d.*;
import sim.portrayal3d.simple.*;
import sim.util.*;
import sim.util.gui.*;
import javax.vecmath.*;
import javax.media.j3d.*;
import com.sun.j3d.utils.picking.*;
import java.awt.*;
public class ValueGridPortrayal3D extends FieldPortrayal3D
{
String valueName;
double scale;
ColorMap map = new SimpleColorMap();
int width = 0;
int height = 0;
int length = 0;
final MutableDouble valueToPass = new MutableDouble(0);
public ColorMap getMap() { return map;}
public void setMap(ColorMap m) { map = m; }
public String getValueName () { return valueName; }
public void setValueName(String name) { valueName = name; }
boolean dirtyScale = false;
public double getScale () { return scale; }
public void setScale(double val) { scale = val; dirtyScale = true; }
ValuePortrayal3D defaultPortrayal = new ValuePortrayal3D();
public Portrayal getDefaultPortrayal()
{
return defaultPortrayal;
}
public void setField(Object field)
{
if (field instanceof IntGrid3D || field instanceof DoubleGrid3D ||
field instanceof IntGrid2D || field instanceof DoubleGrid2D) super.setField(field);
else throw new RuntimeException("Invalid field for ValueGridPortrayal3D: " + field);
}
public Object getField() { return field; }
public ValueGridPortrayal3D()
{
this("Value", 1);
}
public ValueGridPortrayal3D(String valueName)
{
this(valueName, 1);
}
public ValueGridPortrayal3D(double s)
{
this("Value", s);
}
public ValueGridPortrayal3D(String valueName, double scale)
{
this.valueName = valueName;
this.scale = scale;
}
public PolygonAttributes polygonAttributes()
{
return ((Portrayal3D)(getPortrayalForObject(new ValueWrapper(0.0, new Int3D(), this)))).polygonAttributes();
}
/** This method is called by the default inspector to filter new values set by the user.
You should return the "corrected" value if the given value is invalid. The default version
of this method bases values on the values passed into the setLevels() and setColorTable() methods. */
public double newValue(int x, int y, int z, double value)
{
if (field instanceof IntGrid2D || field instanceof IntGrid3D) value = (int) value;
if (map.validLevel(value)) return value;
if (field != null)
{
if (field instanceof DoubleGrid3D)
return ((DoubleGrid3D)field).field[x][y][z];
else if (field instanceof IntGrid3D)
return ((IntGrid3D)field).field[x][y][z];
else if (field instanceof DoubleGrid2D)
return ((DoubleGrid2D)field).field[x][y];
else //if (field instanceof IntGrid2D)
return ((IntGrid2D)field).field[x][y];
}
else return map.defaultValue();
}
// returns the value at the given grid position
double gridValue(int x, int y, int z)
{
if (field instanceof DoubleGrid3D)
return ((DoubleGrid3D)field).field[x][y][z];
else if (field instanceof IntGrid3D)
return ((IntGrid3D)field).field[x][y][z];
else if (field instanceof DoubleGrid2D)
return ((DoubleGrid2D)field).field[x][y];
else //if (field instanceof IntGrid2D)
return ((IntGrid2D)field).field[x][y];
}
public TransformGroup createModel()
{
TransformGroup globalTG = new TransformGroup();
globalTG.setCapability(TransformGroup.ALLOW_CHILDREN_READ);
if (field == null) return globalTG;
dirtyScale = false; // we'll be revising the scale entirely
Switch localSwitch = new Switch(Switch.CHILD_MASK);
localSwitch.setCapability(Switch.ALLOW_SWITCH_READ);
localSwitch.setCapability(Switch.ALLOW_SWITCH_WRITE);
localSwitch.setCapability(Group.ALLOW_CHILDREN_READ);
globalTG.addChild(localSwitch);
extractDimensions(); // set the width, height, and length based on the underlying grid
java.util.BitSet childMask = new java.util.BitSet(width*height*length);
Transform3D trans = new Transform3D();
Portrayal p = getPortrayalForObject(new ValueWrapper(0.0, new Int3D(), this));
if (!(p instanceof SimplePortrayal3D))
throw new RuntimeException("Unexpected Portrayal " + p + "for object " +
valueToPass + " -- expected a SimplePortrayal3D");
SimplePortrayal3D portrayal = (SimplePortrayal3D) p;
portrayal.setCurrentFieldPortrayal(this);
int i = 0;
int width = this.width;
int height = this.height;
int length = this.length;
for (int x=0;x 2) // nontransparent
childMask.set(i);
else
childMask.clear(i);
i++;
}
localSwitch.setChildMask(childMask);
return globalTG;
}
public void updateModel(TransformGroup modelTG)
{
if (field == null) return;
extractDimensions();
Switch localSwitch = (Switch) modelTG.getChild(0);
java.util.BitSet childMask = localSwitch.getChildMask();
Portrayal p = getPortrayalForObject(valueToPass);
if (!(p instanceof SimplePortrayal3D))
throw new RuntimeException("Unexpected Portrayal " + p + "for object " +
valueToPass + " -- expected a SimplePortrayal3D");
SimplePortrayal3D portrayal = (SimplePortrayal3D) p;
portrayal.setCurrentFieldPortrayal(this);
if (dirtyScale || isDirtyField())
reviseScale(localSwitch); // sizes may have changed
int i = 0;
int width = this.width;
int height = this.height;
int length = this.length;
for (int x=0;x 2) // nontransparent
{
childMask.set(i);
wrapper.lastValue = value;
portrayal.getModel(wrapper, tg);
}
else childMask.clear(i);
i++; // next index
}
localSwitch.setChildMask(childMask);
}
void reviseScale(Switch localSwitch)
{
Transform3D trans = new Transform3D();
int i = 0;
int width = this.width;
int height = this.height;
int length = this.length;
for (int x=0;x
© 2015 - 2025 Weber Informatics LLC | Privacy Policy