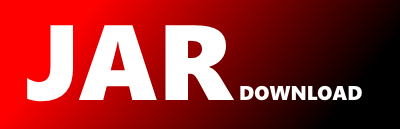
com.veraxsystems.vxipmi.connection.SessionManager Maven / Gradle / Ivy
The newest version!
/*
* SessionManager.java
* Created on 13.06.2017
*
* Copyright (c) Sonalake 2017.
* All rights reserved.
*
* This software is furnished under a license. Use, duplication,
* disclosure and all other uses are restricted to the rights
* specified in the written license agreement.
*/
package com.veraxsystems.vxipmi.connection;
import com.veraxsystems.vxipmi.api.async.ConnectionHandle;
import com.veraxsystems.vxipmi.api.sol.CipherSuiteSelectionHandler;
import com.veraxsystems.vxipmi.api.sync.IpmiConnector;
import com.veraxsystems.vxipmi.coding.commands.PrivilegeLevel;
import com.veraxsystems.vxipmi.coding.security.CipherSuite;
import org.apache.log4j.Logger;
import java.net.InetAddress;
import java.util.List;
import java.util.concurrent.ConcurrentHashMap;
/**
* Manages multiple {@link Session}s.
*/
public class SessionManager {
private static final Logger logger = Logger.getLogger(SessionManager.class);
private static Integer sessionId = 100;
/**
* The session ID generated by the {@link SessionManager}.
* Auto-incremented.
*/
public static synchronized int generateSessionId() {
sessionId %= (Integer.MAX_VALUE / 4);
return sessionId++;
}
public static Session establishSession(IpmiConnector connector, String remoteHost, int remotePort, String user, String password,
CipherSuiteSelectionHandler cipherSuiteSelectionHandler) throws SessionException {
ConnectionHandle handle = null;
try {
handle = connector.createConnection(InetAddress.getByName(remoteHost), remotePort);
List availableCipherSuites = connector.getAvailableCipherSuites(handle);
CipherSuite cipherSuite = cipherSuiteSelectionHandler.choose(availableCipherSuites);
if (cipherSuite == null) {
cipherSuite = CipherSuite.getEmpty();
}
connector.getChannelAuthenticationCapabilities(handle, cipherSuite, PrivilegeLevel.Administrator);
return connector.openSession(handle, user, password, null);
} catch (Exception e) {
closeConnection(connector, handle);
throw new SessionException("Cannot create new session due to exception", e);
}
}
private static void closeConnection(IpmiConnector connector, ConnectionHandle handle) {
try {
if (connector != null && handle != null) {
connector.closeSession(handle);
connector.tearDown();
}
} catch (Exception e) {
logger.error("Cannot close connection after exception thrown during session establishment.", e);
}
}
private final ConcurrentHashMap sessionsPerConnectionHandle;
public SessionManager() {
this.sessionsPerConnectionHandle = new ConcurrentHashMap();
}
/**
* Either returns the {@link Session} registered for given connection, or creates new IPMI {@link Session}
* and registers it for the given connection handle, if no session was found for the connection.
*
* @param connectionHandle
* handle of the connection, for which this session should be registered.
* @return newly created session object or session already registered for the connection
*/
public Session registerSession(int sessionId, ConnectionHandle connectionHandle) {
if (connectionHandle.getUser() == null || connectionHandle.getRemoteAddress() == null) {
throw new IllegalArgumentException("Given connection handle is incomplete (lacks user or remote address)");
}
Session newSession = new Session(sessionId, connectionHandle);
Session currentSession = sessionsPerConnectionHandle.putIfAbsent(connectionHandle.getHandle(), newSession);
return currentSession != null ? currentSession : newSession;
}
/**
* Unregisters session for given connection handle.
*
* @param connectionHandle
* handle of the connection, for which the session should be unregistered.
*/
public void unregisterSession(ConnectionHandle connectionHandle) {
sessionsPerConnectionHandle.remove(connectionHandle.getHandle());
}
/**
* Returns session already bound to given connection handle fulfilling given criteria.
*
* @param remoteAddress
* IP addres of the managed system
* @param remotePort
* UDP port of the managed system
* @param user
* IPMI user for whom the connection is established
* @return session object fulfilling given criteria, or null if no session was registered for such connection.
*/
public Session getSessionForCriteria(InetAddress remoteAddress, int remotePort, String user) {
for (Session session : sessionsPerConnectionHandle.values()) {
ConnectionHandle sessionConnectionHandle = session.getConnectionHandle();
if (sessionConnectionHandle.getUser().equals(user)
&& sessionConnectionHandle.getRemoteAddress().equals(remoteAddress)
&& sessionConnectionHandle.getRemotePort() == remotePort) {
return session;
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy