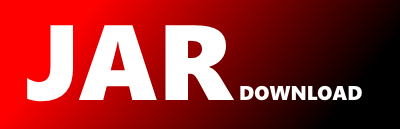
fr.landel.utils.model.mapper.ReflectiveMapperIDO Maven / Gradle / Ivy
/*
* #%L
* utils-model
* %%
* Copyright (C) 2016 Gilandel
* %%
* Authors: Gilles Landel
* URL: https://github.com/Gilandel
*
* This file is under Apache License, version 2.0 (2004).
* #L%
*/
package fr.landel.utils.model.mapper;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.springframework.stereotype.Component;
import fr.landel.utils.commons.asserts.AssertUtils;
import fr.landel.utils.mapper.DTOIdentifier;
import fr.landel.utils.mapper.EnumMode;
import fr.landel.utils.mapper.MapperException;
import fr.landel.utils.mapper.core.AbstractReflectiveMapper;
import fr.landel.utils.model.AbstractDTO;
import fr.landel.utils.model.AbstractEntity;
/**
* Reflective mapper (entity <-> DTO)
*
* @since 29 juil. 2015
* @author Gilles
*
*/
@Component
public class ReflectiveMapperIDO extends AbstractReflectiveMapper {
private static final String ERROR_PARAMETER_MISSING = "%s, The parameter %s is null";
private static final String ERROR_PARAMETER_EMPTY = "%s, The parameter %s is empty";
private static final String ERROR_IDENTIFIER_UNKNOWN = "%s, The identifier %s is unknown";
private static final String ERROR_PARAMETERS_LENGTH = "%s, the parameters %s and %s haven't the same length";
private static final String METHOD_MAP_TO_DTO = "mapToDTO";
private static final String METHOD_MAP_TO_ENTITY = "mapToEntity";
private static final String PARAM_DTO = "dto";
private static final String PARAM_DTO_LIST = "dtoList";
private static final String PARAM_ENTITY = "entity";
private static final String PARAM_ENTITIES = "entities";
private static final String PARAM_IDENTIIER = "identifier";
private static final String PARAM_PREVIOUS_DTO = "previousDTO";
private static final String PARAM_PREVIOUS_DTO_LIST = "previousDTOList";
/**
* Transform entity to DTO.
*
* @param entity
* The entity
* @param identifierKey
* The identifier key
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The dto
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> D mapToDTO(final E entity,
final String identifierKey) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(entity, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_ENTITY);
AssertUtils.isNotNull(identifierKey, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_IDENTIIER);
AssertUtils.isTrue(this.getDtoIdentifierManager().containsKey(identifierKey), ERROR_IDENTIFIER_UNKNOWN, METHOD_MAP_TO_DTO,
PARAM_IDENTIIER);
final DTOIdentifier identifier = this.getDtoIdentifierManager().get(identifierKey);
return this.map(entity, null, identifier, identifier.deep(), EnumMode.LOAD);
}
/**
* Transform entity to DTO.
*
* @param entity
* The entity
* @param identifier
* The identifier
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The dto
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> D mapToDTO(final E entity,
final DTOIdentifier identifier) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(entity, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_ENTITY);
AssertUtils.isNotNull(identifier, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_IDENTIIER);
return this.map(entity, null, identifier, identifier.deep(), EnumMode.LOAD);
}
/**
* Transform entity to DTO.
*
* @param entity
* The entity
* @param previousDTO
* The previous DTO
* @param identifierKey
* The identifier key
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The DTO
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> D mapToDTO(final E entity,
final D previousDTO, final String identifierKey) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(entity, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_ENTITY);
AssertUtils.isNotNull(previousDTO, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_PREVIOUS_DTO);
AssertUtils.isNotNull(identifierKey, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_IDENTIIER);
AssertUtils.isTrue(this.getDtoIdentifierManager().containsKey(identifierKey), ERROR_IDENTIFIER_UNKNOWN, METHOD_MAP_TO_DTO,
PARAM_IDENTIIER);
final DTOIdentifier identifier = this.getDtoIdentifierManager().get(identifierKey);
return this.map(entity, previousDTO, identifier, identifier.deep(), EnumMode.LOAD);
}
/**
* Transform entity to DTO.
*
* @param entity
* The entity
* @param previousDTO
* The previous DTO
* @param identifier
* The identifier
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The dto
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> D mapToDTO(final E entity,
final D previousDTO, final DTOIdentifier identifier) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(entity, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_ENTITY);
AssertUtils.isNotNull(previousDTO, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_PREVIOUS_DTO);
AssertUtils.isNotNull(identifier, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_IDENTIIER);
return this.map(entity, previousDTO, identifier, identifier.deep(), EnumMode.LOAD);
}
/**
* Transform entities to a list of DTO.
*
* @param entities
* The entities
* @param identifierKey
* The identifier key
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The list of dto
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> List mapToDTO(
final List entities, final String identifierKey) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(identifierKey, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_IDENTIIER);
AssertUtils.isTrue(this.getDtoIdentifierManager().containsKey(identifierKey), ERROR_IDENTIFIER_UNKNOWN, METHOD_MAP_TO_DTO,
PARAM_IDENTIIER);
final DTOIdentifier identifier = this.getDtoIdentifierManager().get(identifierKey);
return this.mapToDTO(entities, identifier);
}
/**
* Transform entities to a list of DTO.
*
* @param entities
* The entities
* @param identifier
* The identifier
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The list of dto
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> List mapToDTO(
final List entities, final DTOIdentifier identifier) throws MapperException, IllegalArgumentException {
AssertUtils.isNotEmpty(entities, ERROR_PARAMETER_EMPTY, METHOD_MAP_TO_DTO, PARAM_ENTITIES);
AssertUtils.isNotNull(identifier, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_IDENTIIER);
final List dtos = new ArrayList<>();
for (E entity : entities) {
AssertUtils.isNotNull(entity, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_ENTITY);
dtos.add(this.map(entity, (D) null, identifier, identifier.deep(), EnumMode.LOAD));
}
return dtos;
}
/**
* Transform entities into a list of DTO.
*
* @param entities
* The entities
* @param previousDTOList
* The previous DTO list
* @param identifierKey
* The identifier key
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The DTO
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> List mapToDTO(
final List entities, final List previousDTOList, final String identifierKey)
throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(identifierKey, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_IDENTIIER);
AssertUtils.isTrue(this.getDtoIdentifierManager().containsKey(identifierKey), ERROR_IDENTIFIER_UNKNOWN, METHOD_MAP_TO_DTO,
PARAM_IDENTIIER);
final DTOIdentifier identifier = this.getDtoIdentifierManager().get(identifierKey);
return this.mapToDTO(entities, previousDTOList, identifier);
}
/**
* Transform entities to a list of DTO.
*
* @param entities
* The entities
* @param previousDTOList
* The previous DTO list
* @param identifier
* The identifier
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return The DTO
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> List mapToDTO(
final List entities, final List previousDTOList, final DTOIdentifier identifier)
throws MapperException, IllegalArgumentException {
AssertUtils.isNotEmpty(entities, ERROR_PARAMETER_EMPTY, METHOD_MAP_TO_DTO, PARAM_ENTITIES);
AssertUtils.isNotEmpty(previousDTOList, ERROR_PARAMETER_EMPTY, METHOD_MAP_TO_DTO, PARAM_PREVIOUS_DTO_LIST);
AssertUtils.isEqual(entities.size(), previousDTOList.size(), ERROR_PARAMETERS_LENGTH, METHOD_MAP_TO_DTO, PARAM_ENTITIES,
PARAM_PREVIOUS_DTO_LIST);
final List dtos = new ArrayList<>();
final Iterator itEntity = entities.iterator();
final Iterator itDTO = previousDTOList.iterator();
while (itEntity.hasNext() && itDTO.hasNext()) {
final E entity = itEntity.next();
final D previousDTO = itDTO.next();
AssertUtils.isNotNull(entity, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_ENTITY);
AssertUtils.isNotNull(previousDTO, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_PREVIOUS_DTO);
dtos.add(this.map(entity, previousDTO, identifier, identifier.deep(), EnumMode.LOAD));
}
return dtos;
}
/**
* Map DTO to entity.
*
* @param dto
* the DTO
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return the entity
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> E mapToEntity(final D dto)
throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(dto, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_DTO);
return this.map(dto, null, null, DTOIdentifier.MAX_DEEP, EnumMode.SAVE);
}
/**
* Map DTO to entity.
*
* @param dto
* the DTO
* @param entity
* The destination entity
* @param identifierKey
* The identifier key to load
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return the entity
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> E mapToEntity(final D dto,
final E entity, final String identifierKey) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(dto, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_DTO);
AssertUtils.isNotNull(identifierKey, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_IDENTIIER);
AssertUtils.isTrue(this.getDtoIdentifierManager().containsKey(identifierKey), ERROR_IDENTIFIER_UNKNOWN, METHOD_MAP_TO_ENTITY,
PARAM_IDENTIIER);
final DTOIdentifier identifier = this.getDtoIdentifierManager().get(identifierKey);
return this.map(dto, entity, identifier, identifier.deep(), EnumMode.SAVE);
}
/**
* Map DTO to entity.
*
* @param dto
* the DTO
* @param entity
* The destination entity
* @param identifier
* The identifier to load
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return the entity
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> E mapToEntity(final D dto,
final E entity, final DTOIdentifier identifier) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(dto, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_DTO);
AssertUtils.isNotNull(identifier, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_IDENTIIER);
return this.map(dto, entity, identifier, identifier.deep(), EnumMode.SAVE);
}
/**
* Map a DTO list into entities.
*
* @param dtoList
* the DTO list
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return the entities
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> List mapToEntity(
final List dtoList) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(dtoList, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_DTO_LIST);
AssertUtils.isNotEmpty(dtoList, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_DTO_LIST);
final List entities = new ArrayList<>();
for (D dto : dtoList) {
AssertUtils.isNotNull(dto, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_DTO);
entities.add(this.map(dto, (E) null, null, DTOIdentifier.MAX_DEEP, EnumMode.SAVE));
}
return entities;
}
/**
* Map a DTO list into entities.
*
* @param dtoList
* the DTO list
* @param entities
* The destination entities
* @param identifierKey
* The identifier key to load
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return the entity
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> List mapToEntity(
final List dtoList, final List entities, final String identifierKey) throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(identifierKey, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_IDENTIIER);
AssertUtils.isTrue(this.getDtoIdentifierManager().containsKey(identifierKey), ERROR_IDENTIFIER_UNKNOWN, METHOD_MAP_TO_ENTITY,
PARAM_IDENTIIER);
final DTOIdentifier identifier = this.getDtoIdentifierManager().get(identifierKey);
return this.mapToEntity(dtoList, entities, identifier);
}
/**
* Map a DTO list into entities.
*
* @param dtoList
* the DTO list
* @param entities
* The destination entities
* @param identifier
* The identifier to load
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
* @return the entity
* @throws MapperException
* If mapping failed
* @throws IllegalArgumentException
* If parameters are not correctly set
*/
public , D extends AbstractDTO, K extends Serializable & Comparable> List mapToEntity(
final List dtoList, final List entities, final DTOIdentifier identifier)
throws MapperException, IllegalArgumentException {
AssertUtils.isNotNull(dtoList, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_DTO_LIST);
AssertUtils.isNotNull(entities, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_ENTITIES);
AssertUtils.isEqual(dtoList.size(), entities.size(), ERROR_PARAMETERS_LENGTH, METHOD_MAP_TO_ENTITY, PARAM_DTO_LIST, PARAM_ENTITIES);
AssertUtils.isNotNull(identifier, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_ENTITY, PARAM_IDENTIIER);
final List entitiesOut = new ArrayList<>();
final Iterator itEntity = entities.iterator();
final Iterator itDTO = dtoList.iterator();
while (itEntity.hasNext() && itDTO.hasNext()) {
final E entity = itEntity.next();
final D dto = itDTO.next();
AssertUtils.isNotNull(entity, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_ENTITY);
AssertUtils.isNotNull(dto, ERROR_PARAMETER_MISSING, METHOD_MAP_TO_DTO, PARAM_PREVIOUS_DTO);
entitiesOut.add(this.map(dto, entity, identifier, identifier.deep(), EnumMode.SAVE));
}
return entitiesOut;
}
/**
* Prepare the mapping of the entity into DTO
*
* @param entity
* The entity
* @param dto
* the DTO
* @param identifier
* The identifier
* @param deep
* The current deep
* @param mode
* The current mapping mode
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
*/
protected , D extends AbstractDTO, K extends Serializable & Comparable> void prepareEntityToDTO(
E entity, D dto, DTOIdentifier identifier, int deep, EnumMode mode) {
if (dto != null) {
dto.setPrimaryKey(entity.getPrimaryKey());
if (EnumMode.LOAD.equals(mode) || EnumMode.DEFAULT.equals(mode)) {
dto.setLoaded(true);
}
}
}
/**
* Prepare the mapping of the DTO into entity
*
* @param entity
* The entity
* @param dto
* the DTO
* @param identifier
* The identifier
* @param deep
* The current deep
* @param mode
* The current mapping mode
* @param
* The DTO type
* @param
* The entity type
* @param
* The primary key type
*/
protected , D extends AbstractDTO, K extends Serializable & Comparable> void prepareDTOToEntity(
E entity, D dto, DTOIdentifier identifier, int deep, EnumMode mode) {
if (entity != null) {
entity.setPrimaryKey(dto.getPrimaryKey());
}
}
@SuppressWarnings({"unchecked", "rawtypes"})
@Override
protected void prepareMapping(Object sourceObject, Object targetObject, DTOIdentifier identifier, int deep, EnumMode mode) {
if (AbstractEntity.class.isAssignableFrom(sourceObject.getClass())) {
this.prepareEntityToDTO((AbstractEntity) sourceObject, (AbstractDTO) targetObject, identifier, deep, mode);
} else {
this.prepareDTOToEntity((AbstractEntity) targetObject, (AbstractDTO) sourceObject, identifier, deep, mode);
}
}
@Override
protected void postMapping(Object sourceObject, Object targetObject, DTOIdentifier identifier, int deep, EnumMode mode) {
// DO nothing
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy