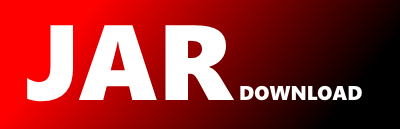
fr.lecomptoirdespharmacies.offisante.esignature.model.CreateDocumentRequest Maven / Gradle / Ivy
The newest version!
/*
* OffiSanté ESignature API
* OffiSanté Electronic Signature API Documentation
*
* The version of the OpenAPI document: 1.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package fr.lecomptoirdespharmacies.offisante.esignature.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* CreateDocumentRequest
*/
@JsonPropertyOrder({
CreateDocumentRequest.JSON_PROPERTY_EMAIL,
CreateDocumentRequest.JSON_PROPERTY_FIRST_NAME,
CreateDocumentRequest.JSON_PROPERTY_LAST_NAME,
CreateDocumentRequest.JSON_PROPERTY_PHONE,
CreateDocumentRequest.JSON_PROPERTY_PHARMACY_NAME,
CreateDocumentRequest.JSON_PROPERTY_ADRESS,
CreateDocumentRequest.JSON_PROPERTY_FINESS,
CreateDocumentRequest.JSON_PROPERTY_SOCIAL_FORM,
CreateDocumentRequest.JSON_PROPERTY_RCS,
CreateDocumentRequest.JSON_PROPERTY_CIP,
CreateDocumentRequest.JSON_PROPERTY_SIRET,
CreateDocumentRequest.JSON_PROPERTY_LGO,
CreateDocumentRequest.JSON_PROPERTY_TYPOLOGY,
CreateDocumentRequest.JSON_PROPERTY_GROUP,
CreateDocumentRequest.JSON_PROPERTY_RPPS
})
@JsonTypeName("createDocument_request")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.5.0")
public class CreateDocumentRequest {
public static final String JSON_PROPERTY_EMAIL = "email";
private String email;
public static final String JSON_PROPERTY_FIRST_NAME = "first_name";
private String firstName;
public static final String JSON_PROPERTY_LAST_NAME = "last_name";
private String lastName;
public static final String JSON_PROPERTY_PHONE = "phone";
private String phone;
public static final String JSON_PROPERTY_PHARMACY_NAME = "pharmacy_name";
private String pharmacyName;
public static final String JSON_PROPERTY_ADRESS = "adress";
private String adress;
public static final String JSON_PROPERTY_FINESS = "finess";
private String finess;
public static final String JSON_PROPERTY_SOCIAL_FORM = "social_form";
private String socialForm;
public static final String JSON_PROPERTY_RCS = "rcs";
private String rcs;
public static final String JSON_PROPERTY_CIP = "cip";
private String cip;
public static final String JSON_PROPERTY_SIRET = "siret";
private String siret;
public static final String JSON_PROPERTY_LGO = "lgo";
private String lgo;
public static final String JSON_PROPERTY_TYPOLOGY = "typology";
private String typology;
public static final String JSON_PROPERTY_GROUP = "group";
private String group;
public static final String JSON_PROPERTY_RPPS = "rpps";
private String rpps;
public CreateDocumentRequest() {
}
public CreateDocumentRequest email(String email) {
this.email = email;
return this;
}
/**
* Email of the signer
* @return email
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_EMAIL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEmail() {
return email;
}
@JsonProperty(JSON_PROPERTY_EMAIL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEmail(String email) {
this.email = email;
}
public CreateDocumentRequest firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* First name of the signer
* @return firstName
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_FIRST_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getFirstName() {
return firstName;
}
@JsonProperty(JSON_PROPERTY_FIRST_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public CreateDocumentRequest lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Last name of the signer
* @return lastName
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_LAST_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getLastName() {
return lastName;
}
@JsonProperty(JSON_PROPERTY_LAST_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLastName(String lastName) {
this.lastName = lastName;
}
public CreateDocumentRequest phone(String phone) {
this.phone = phone;
return this;
}
/**
* Phone number of the signer
* @return phone
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_PHONE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getPhone() {
return phone;
}
@JsonProperty(JSON_PROPERTY_PHONE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPhone(String phone) {
this.phone = phone;
}
public CreateDocumentRequest pharmacyName(String pharmacyName) {
this.pharmacyName = pharmacyName;
return this;
}
/**
* Name of the pharmacy for pharmacy templates
* @return pharmacyName
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PHARMACY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPharmacyName() {
return pharmacyName;
}
@JsonProperty(JSON_PROPERTY_PHARMACY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPharmacyName(String pharmacyName) {
this.pharmacyName = pharmacyName;
}
public CreateDocumentRequest adress(String adress) {
this.adress = adress;
return this;
}
/**
* Adress of the pharmacy for pharmacy templates
* @return adress
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ADRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAdress() {
return adress;
}
@JsonProperty(JSON_PROPERTY_ADRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAdress(String adress) {
this.adress = adress;
}
public CreateDocumentRequest finess(String finess) {
this.finess = finess;
return this;
}
/**
* FINESS number of the pharmacy for pharmacy templates
* @return finess
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FINESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFiness() {
return finess;
}
@JsonProperty(JSON_PROPERTY_FINESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFiness(String finess) {
this.finess = finess;
}
public CreateDocumentRequest socialForm(String socialForm) {
this.socialForm = socialForm;
return this;
}
/**
* Social form of the pharmacy for pharmacy templates
* @return socialForm
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SOCIAL_FORM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSocialForm() {
return socialForm;
}
@JsonProperty(JSON_PROPERTY_SOCIAL_FORM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSocialForm(String socialForm) {
this.socialForm = socialForm;
}
public CreateDocumentRequest rcs(String rcs) {
this.rcs = rcs;
return this;
}
/**
* RCS number of the pharmacy for pharmacy templates
* @return rcs
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RCS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRcs() {
return rcs;
}
@JsonProperty(JSON_PROPERTY_RCS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRcs(String rcs) {
this.rcs = rcs;
}
public CreateDocumentRequest cip(String cip) {
this.cip = cip;
return this;
}
/**
* CIP of the pharmacy for pharmacy templates
* @return cip
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CIP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCip() {
return cip;
}
@JsonProperty(JSON_PROPERTY_CIP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCip(String cip) {
this.cip = cip;
}
public CreateDocumentRequest siret(String siret) {
this.siret = siret;
return this;
}
/**
* SIRET number of the pharmacy for pharmacy templates
* @return siret
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SIRET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSiret() {
return siret;
}
@JsonProperty(JSON_PROPERTY_SIRET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSiret(String siret) {
this.siret = siret;
}
public CreateDocumentRequest lgo(String lgo) {
this.lgo = lgo;
return this;
}
/**
* LGO of the pharmacy (LGPI, WINPHARMA, LEO, etc) for pharmacy templates
* @return lgo
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_LGO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getLgo() {
return lgo;
}
@JsonProperty(JSON_PROPERTY_LGO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLgo(String lgo) {
this.lgo = lgo;
}
public CreateDocumentRequest typology(String typology) {
this.typology = typology;
return this;
}
/**
* Typology of the pharmacy (rurale, centre ville, zone commerciale, etc) for pharmacy templates
* @return typology
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TYPOLOGY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTypology() {
return typology;
}
@JsonProperty(JSON_PROPERTY_TYPOLOGY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTypology(String typology) {
this.typology = typology;
}
public CreateDocumentRequest group(String group) {
this.group = group;
return this;
}
/**
* Groupement of the pharmacy for pharmacy templates
* @return group
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GROUP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getGroup() {
return group;
}
@JsonProperty(JSON_PROPERTY_GROUP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGroup(String group) {
this.group = group;
}
public CreateDocumentRequest rpps(String rpps) {
this.rpps = rpps;
return this;
}
/**
* RPPS number of the pharmacy for medecin templates
* @return rpps
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_RPPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRpps() {
return rpps;
}
@JsonProperty(JSON_PROPERTY_RPPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRpps(String rpps) {
this.rpps = rpps;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateDocumentRequest createDocumentRequest = (CreateDocumentRequest) o;
return Objects.equals(this.email, createDocumentRequest.email) &&
Objects.equals(this.firstName, createDocumentRequest.firstName) &&
Objects.equals(this.lastName, createDocumentRequest.lastName) &&
Objects.equals(this.phone, createDocumentRequest.phone) &&
Objects.equals(this.pharmacyName, createDocumentRequest.pharmacyName) &&
Objects.equals(this.adress, createDocumentRequest.adress) &&
Objects.equals(this.finess, createDocumentRequest.finess) &&
Objects.equals(this.socialForm, createDocumentRequest.socialForm) &&
Objects.equals(this.rcs, createDocumentRequest.rcs) &&
Objects.equals(this.cip, createDocumentRequest.cip) &&
Objects.equals(this.siret, createDocumentRequest.siret) &&
Objects.equals(this.lgo, createDocumentRequest.lgo) &&
Objects.equals(this.typology, createDocumentRequest.typology) &&
Objects.equals(this.group, createDocumentRequest.group) &&
Objects.equals(this.rpps, createDocumentRequest.rpps);
}
@Override
public int hashCode() {
return Objects.hash(email, firstName, lastName, phone, pharmacyName, adress, finess, socialForm, rcs, cip, siret, lgo, typology, group, rpps);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateDocumentRequest {\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" pharmacyName: ").append(toIndentedString(pharmacyName)).append("\n");
sb.append(" adress: ").append(toIndentedString(adress)).append("\n");
sb.append(" finess: ").append(toIndentedString(finess)).append("\n");
sb.append(" socialForm: ").append(toIndentedString(socialForm)).append("\n");
sb.append(" rcs: ").append(toIndentedString(rcs)).append("\n");
sb.append(" cip: ").append(toIndentedString(cip)).append("\n");
sb.append(" siret: ").append(toIndentedString(siret)).append("\n");
sb.append(" lgo: ").append(toIndentedString(lgo)).append("\n");
sb.append(" typology: ").append(toIndentedString(typology)).append("\n");
sb.append(" group: ").append(toIndentedString(group)).append("\n");
sb.append(" rpps: ").append(toIndentedString(rpps)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy