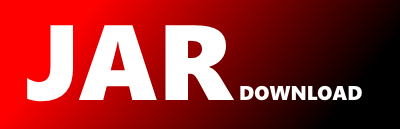
fr.lip6.move.pnml.symmetricnet.partitions.impl.PartitionImpl Maven / Gradle / Ivy
/**
* Copyright 2009-2016 Université Paris Ouest and Sorbonne Universités,
* Univ. Paris 06 - CNRS UMR 7606 (LIP6)
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Project leader / Initial Contributor:
* Lom Messan Hillah -
*
* Contributors:
* ${ocontributors} - <$oemails}>
*
* Mailing list:
* [email protected]
*/
/**
* (C) Sorbonne Universités, UPMC Univ Paris 06, UMR CNRS 7606 (LIP6/MoVe)
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Lom HILLAH (LIP6) - Initial models and implementation
* Rachid Alahyane (UPMC) - Infrastructure and continuous integration
* Bastien Bouzerau (UPMC) - Architecture
* Guillaume Giffo (UPMC) - Code generation refactoring, High-level API
*/
package fr.lip6.move.pnml.symmetricnet.partitions.impl;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.charset.Charset;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import javax.xml.namespace.QName;
import org.apache.axiom.om.OMElement;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.common.util.DiagnosticChain;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.util.EObjectContainmentWithInverseEList;
import org.eclipse.emf.ecore.util.InternalEList;
import fr.lip6.move.pnml.framework.general.PnmlExport;
import fr.lip6.move.pnml.framework.utils.IdRefLinker;
import fr.lip6.move.pnml.framework.utils.ModelRepository;
import fr.lip6.move.pnml.framework.utils.PNMLEncoding;
import fr.lip6.move.pnml.framework.utils.PrettyPrintData;
import fr.lip6.move.pnml.framework.utils.exception.InnerBuildException;
import fr.lip6.move.pnml.framework.utils.exception.InvalidIDException;
import fr.lip6.move.pnml.framework.utils.exception.VoidRepositoryException;
import fr.lip6.move.pnml.symmetricnet.booleans.Bool;
import fr.lip6.move.pnml.symmetricnet.booleans.BooleansFactory;
import fr.lip6.move.pnml.symmetricnet.cyclicEnumerations.CyclicEnumeration;
import fr.lip6.move.pnml.symmetricnet.cyclicEnumerations.CyclicEnumerationsFactory;
import fr.lip6.move.pnml.symmetricnet.dots.Dot;
import fr.lip6.move.pnml.symmetricnet.dots.DotsFactory;
import fr.lip6.move.pnml.symmetricnet.finiteEnumerations.FiniteEnumeration;
import fr.lip6.move.pnml.symmetricnet.finiteEnumerations.FiniteEnumerationsFactory;
import fr.lip6.move.pnml.symmetricnet.finiteIntRanges.FiniteIntRange;
import fr.lip6.move.pnml.symmetricnet.finiteIntRanges.FiniteIntRangesFactory;
import fr.lip6.move.pnml.symmetricnet.integers.HLInteger;
import fr.lip6.move.pnml.symmetricnet.integers.IntegersFactory;
import fr.lip6.move.pnml.symmetricnet.integers.Natural;
import fr.lip6.move.pnml.symmetricnet.integers.Positive;
import fr.lip6.move.pnml.symmetricnet.partitions.Partition;
import fr.lip6.move.pnml.symmetricnet.partitions.PartitionElement;
import fr.lip6.move.pnml.symmetricnet.partitions.PartitionsFactory;
import fr.lip6.move.pnml.symmetricnet.partitions.PartitionsPackage;
import fr.lip6.move.pnml.symmetricnet.terms.MultisetSort;
import fr.lip6.move.pnml.symmetricnet.terms.ProductSort;
import fr.lip6.move.pnml.symmetricnet.terms.Sort;
import fr.lip6.move.pnml.symmetricnet.terms.TermsFactory;
import fr.lip6.move.pnml.symmetricnet.terms.TermsPackage;
import fr.lip6.move.pnml.symmetricnet.terms.UserSort;
import fr.lip6.move.pnml.symmetricnet.terms.impl.SortDeclImpl;
import fr.lip6.move.pnml.symmetricnet.terms.util.TermsValidator;
/**
*
* An implementation of the model object 'Partition'.
*
*
* The following features are implemented:
*
* - {@link fr.lip6.move.pnml.symmetricnet.partitions.impl.PartitionImpl#getDef Def}
* - {@link fr.lip6.move.pnml.symmetricnet.partitions.impl.PartitionImpl#getPartitionelements Partitionelements}
*
*
*
* @generated
*/
public class PartitionImpl extends SortDeclImpl implements Partition {
/**
* The cached value of the '{@link #getDef() Def}' containment reference.
*
*
* @see #getDef()
* @generated
* @ordered
*/
protected Sort def;
/**
* The cached value of the '{@link #getPartitionelements() Partitionelements}' containment reference list.
*
*
* @see #getPartitionelements()
* @generated
* @ordered
*/
protected EList partitionelements;
/**
*
*
* @generated
*/
protected PartitionImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return PartitionsPackage.Literals.PARTITION;
}
/**
*
*
* @generated
*/
@Override
public Sort getDef() {
return def;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetDef(Sort newDef, NotificationChain msgs) {
Sort oldDef = def;
def = newDef;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET,
PartitionsPackage.PARTITION__DEF, oldDef, newDef);
if (msgs == null)
msgs = notification;
else
msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setDef(Sort newDef) {
if (newDef != def) {
NotificationChain msgs = null;
if (def != null)
msgs = ((InternalEObject) def).eInverseRemove(this, TermsPackage.SORT__CONTAINER_PARTITION, Sort.class,
msgs);
if (newDef != null)
msgs = ((InternalEObject) newDef).eInverseAdd(this, TermsPackage.SORT__CONTAINER_PARTITION, Sort.class,
msgs);
msgs = basicSetDef(newDef, msgs);
if (msgs != null)
msgs.dispatch();
} else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, PartitionsPackage.PARTITION__DEF, newDef, newDef));
}
/**
*
*
* @generated
*/
@Override
public List getPartitionelements() {
if (partitionelements == null) {
partitionelements = new EObjectContainmentWithInverseEList(PartitionElement.class, this,
PartitionsPackage.PARTITION__PARTITIONELEMENTS, PartitionsPackage.PARTITION_ELEMENT__REFPARTITION);
}
return partitionelements;
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
@Override
public NotificationChain eInverseAdd(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case PartitionsPackage.PARTITION__DEF:
if (def != null)
msgs = ((InternalEObject) def).eInverseRemove(this, EOPPOSITE_FEATURE_BASE
- PartitionsPackage.PARTITION__DEF, null, msgs);
return basicSetDef((Sort) otherEnd, msgs);
case PartitionsPackage.PARTITION__PARTITIONELEMENTS:
return ((InternalEList) (InternalEList>) getPartitionelements())
.basicAdd(otherEnd, msgs);
}
return super.eInverseAdd(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case PartitionsPackage.PARTITION__DEF:
return basicSetDef(null, msgs);
case PartitionsPackage.PARTITION__PARTITIONELEMENTS:
return ((InternalEList>) getPartitionelements()).basicRemove(otherEnd, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType) {
switch (featureID) {
case PartitionsPackage.PARTITION__DEF:
return getDef();
case PartitionsPackage.PARTITION__PARTITIONELEMENTS:
return getPartitionelements();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
@Override
public void eSet(int featureID, Object newValue) {
switch (featureID) {
case PartitionsPackage.PARTITION__DEF:
setDef((Sort) newValue);
return;
case PartitionsPackage.PARTITION__PARTITIONELEMENTS:
getPartitionelements().clear();
getPartitionelements().addAll((Collection extends PartitionElement>) newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID) {
switch (featureID) {
case PartitionsPackage.PARTITION__DEF:
setDef((Sort) null);
return;
case PartitionsPackage.PARTITION__PARTITIONELEMENTS:
getPartitionelements().clear();
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID) {
switch (featureID) {
case PartitionsPackage.PARTITION__DEF:
return def != null;
case PartitionsPackage.PARTITION__PARTITIONELEMENTS:
return partitionelements != null && !partitionelements.isEmpty();
}
return super.eIsSet(featureID);
}
/**
* Return the string containing the pnml output
*/
@Override
public String toPNML() {
//id 1
//idref 0
//attributes 1
//sons 2
Boolean prettyPrintStatus = ModelRepository.getInstance().isPrettyPrintActive();
String retline = "";
String headline = "";
PrettyPrintData prpd = null;
if (prettyPrintStatus) {
retline = "\n";
prpd = ModelRepository.getInstance().getPrettyPrintData();
headline = prpd.getCurrentLineHeader();
}
StringBuilder sb = new StringBuilder();
sb.append(headline);
sb.append("");
sb.append(retline);
//sons, follow processing
if (getDef() != null) {
String top = getDef().toPNML();
if (!top.equals("")) {
sb.append(top);
haveSons = true;
}
}
if (getPartitionelements() != null) {
java.util.List items = getPartitionelements();
for (Iterator iterator = items.iterator(); iterator.hasNext();) {
PartitionElement item = iterator.next();
sb.append(item.toPNML());
haveSons = true;
}
}
/****/
if (prettyPrintStatus) {
headline = prpd.decreaseLineHeaderLevel();
}
if (haveSons) {
sb.append(headline);
sb.append(" ");
sb.append(retline);
} else {
sb.delete(sb.lastIndexOf(">"), sb.length());
sb.append("/>");
}
return sb.toString();
}
@Override
@SuppressWarnings("unchecked")
public void fromPNML(OMElement locRoot, IdRefLinker idr) throws InnerBuildException, InvalidIDException,
VoidRepositoryException {
//1
//0
//1
//2
@SuppressWarnings("unused")
PartitionsFactory fact = PartitionsFactory.eINSTANCE;
//processing id
if (locRoot.getAttributeValue(new QName("id")) != null) {
this.setId(new java.lang.String(locRoot.getAttributeValue(new QName("id"))));
ModelRepository.getInstance().getCurrentIdRepository()
.checkId(new java.lang.String(locRoot.getAttributeValue(new QName("id"))).toString(), this);
}
//processing idref
//processing attributes
if (locRoot.getAttributeValue(new QName("name")) != null) {
try {
this.setName(new String(locRoot.getAttributeValue(new QName("name"))));
} catch (Exception e) {
throw new fr.lip6.move.pnml.framework.utils.exception.InnerBuildException(e);
}
}
//processing sons
for (Iterator iterator = locRoot.getChildElements(); iterator.hasNext();) {
@SuppressWarnings("unused")
OMElement type = (OMElement) iterator.next();
if (type.getLocalName().equals("null")) {
Bool item;
item = BooleansFactory.eINSTANCE.createBool();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("cyclicenumeration")) {
CyclicEnumeration item;
item = CyclicEnumerationsFactory.eINSTANCE.createCyclicEnumeration();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("dot")) {
Dot item;
item = DotsFactory.eINSTANCE.createDot();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("finiteenumeration")) {
FiniteEnumeration item;
item = FiniteEnumerationsFactory.eINSTANCE.createFiniteEnumeration();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("finiteintrange")) {
FiniteIntRange item;
item = FiniteIntRangesFactory.eINSTANCE.createFiniteIntRange();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("natural")) {
Natural item;
item = IntegersFactory.eINSTANCE.createNatural();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("positive")) {
Positive item;
item = IntegersFactory.eINSTANCE.createPositive();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("integer")) {
HLInteger item;
item = IntegersFactory.eINSTANCE.createHLInteger();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("multisetsort")) {
MultisetSort item;
item = TermsFactory.eINSTANCE.createMultisetSort();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("productsort")) {
ProductSort item;
item = TermsFactory.eINSTANCE.createProductSort();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("usersort")) {
UserSort item;
item = TermsFactory.eINSTANCE.createUserSort();
item.fromPNML(type, idr);
item.setContainerPartition(this);
continue;
}//end if
if (type.getLocalName().equals("partitionelement")) {
PartitionElement item;
item = PartitionsFactory.eINSTANCE.createPartitionElement();
item.fromPNML(type, idr);
item.setRefpartition(this);
continue;
}//end if
}
}
/**
* Return the string containing the pnml output
*/
@Override
public void toPNML(FileChannel fc) {
//id 1
//idref 0
//attributes 1
//sons 2
final int bufferSizeKB = 8;
final int bufferSize = bufferSizeKB * 1024;
final ByteBuffer bytebuf = ByteBuffer.allocateDirect(bufferSize);
final String charsetEncoding = PNMLEncoding.ISO_8859_1.getName();
Boolean prettyPrintStatus = ModelRepository.getInstance().isPrettyPrintActive();
String retline = "";
String headline = "";
PrettyPrintData prpd = null;
if (prettyPrintStatus) {
retline = "\n";
prpd = ModelRepository.getInstance().getPrettyPrintData();
headline = prpd.getCurrentLineHeader();
}
StringBuilder sb = new StringBuilder();
sb.append(headline);
sb.append("");
sb.append(retline);
//sons, follow processing
if (getDef() != null) {
String top = getDef().toPNML();
if (!top.equals("")) {
sb.append(top);
haveSons = true;
}
}
if (getPartitionelements() != null) {
try {
writeIntoStream(bytebuf, fc, sb.toString().getBytes(Charset.forName(charsetEncoding)));
} catch (IOException io) {
io.printStackTrace();
// fail fast
return;
}
sb.delete(0, sb.length());
java.util.List items = getPartitionelements();
for (Iterator iterator = items.iterator(); iterator.hasNext();) {
PartitionElement item = iterator.next();
item.toPNML(fc);
haveSons = true;
}
}
/****/
if (prettyPrintStatus) {
headline = prpd.decreaseLineHeaderLevel();
}
sb.append(headline);
sb.append(" ");
sb.append(retline);
try {
writeIntoStream(bytebuf, fc, sb.toString().getBytes(Charset.forName(charsetEncoding)));
} catch (IOException io) {
io.printStackTrace();
// fail fast
return;
}
sb = null;
}
/**
* Writes buffer of a given max size into file channel.
*/
private static final void writeIntoStream(final ByteBuffer bytebuf, final FileChannel fc, final byte[] contents)
throws IOException {
final int chopSize = 6 * 1024;
if (contents.length >= bytebuf.capacity()) {
List chops = PnmlExport.chopBytes(contents, chopSize);
for (byte[] buf : chops) {
bytebuf.put(buf);
bytebuf.flip();
fc.write(bytebuf);
bytebuf.clear();
}
} else {
bytebuf.put(contents);
bytebuf.flip();
fc.write(bytebuf);
bytebuf.clear();
}
}
/**
* -
*/
@Override
public boolean validateOCL(DiagnosticChain diagnostics) {
TermsValidator val = new TermsValidator();
boolean retour = true;
if (getDef() != null) {
retour &= getDef().validateOCL(diagnostics);
}
if (getPartitionelements() != null) {
java.util.List items = getPartitionelements();
for (Iterator iterator = items.iterator(); iterator.hasNext();) {
PartitionElement item = iterator.next();
retour &= item.validateOCL(diagnostics);
}
}
retour &= val.validate(this, diagnostics, null);
return retour;
}
} //PartitionImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy