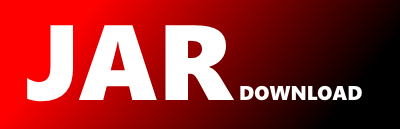
logManager.LogManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brunner-basic-app Show documentation
Show all versions of brunner-basic-app Show documentation
Default application for the BRunner project
package logManager;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Iterator;
import org.slf4j.ILoggerFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.helpers.NOPLoggerFactory;
import app.BRunnerApplication;
import ch.qos.logback.classic.LoggerContext;
import ch.qos.logback.classic.spi.ILoggingEvent;
import ch.qos.logback.core.Appender;
import ch.qos.logback.core.FileAppender;
/**
* Handles the log for the demo.
*/
public class LogManager {
/**
* Message when starting the log.
*/
public static void logStart() {
Logger LOG = LoggerFactory.getLogger(BRunnerApplication.class);
File basePath = new File(".");
String baseAbsolutePath = basePath.getAbsolutePath();
LOG.debug("Base path for execution: " + baseAbsolutePath);
}
/**
* Returns log file name.
*/
public static String getLogFileName() throws Exception {
ILoggerFactory context = LoggerFactory.getILoggerFactory();
switch (context) {
case LoggerContext c -> {
for (ch.qos.logback.classic.Logger logger : c.getLoggerList()) {
Iterator> x = logger.iteratorForAppenders();
while (x.hasNext()) {
Appender appender = x.next();
if (appender instanceof FileAppender) {
return ((FileAppender>) appender).getFile();
}
}
}
}
case NOPLoggerFactory o -> throw new Exception("No log implementation found");
default -> {
return null;
}
};
return null;
}
/**
* Deletes all lines from the log.
*
* @throws Exception
*/
public static void clearLog() throws Exception {
Logger LOG = LoggerFactory.getLogger(BRunnerApplication.class);
try (FileOutputStream fos = new FileOutputStream(getLogFileName(), false)) {
LOG.debug("Log file cleared.");
} catch (IOException e) {
System.err.println("An error occurred while clearing the log file: " + e.getMessage());
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy