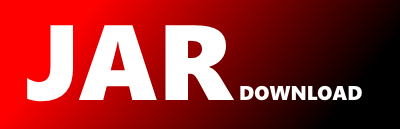
cli.BRunnerCLI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brunner-basic-app Show documentation
Show all versions of brunner-basic-app Show documentation
Default application for the BRunner project
The newest version!
package cli;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import api.running.IBenchmarkRunner;
import runners.basic.running.BasicBenchmarkRunner;
import runners.jmh.running.JMHBenchmarkRunner;
public class BRunnerCLI {
public record CLIInput(List files, IBenchmarkRunner runner) {
};
public static CLIInput processInputs(String[] args) {
CommandLineParser parser = new DefaultParser();
Options options = BRunnerCLIOptions.getOptions();
options.addOption("help", false, "Print this message");
boolean basic_runner = false;
List files = new ArrayList<>();
try {
// Parse the command-line arguments
CommandLine line = parser.parse(options, args);
if (line.hasOption("help")) {
printHelp(options);
return null; // Stop further execution or handle differently
}
if (line.hasOption("basic")) {
basic_runner = true;
}
// Remaining arguments that were not parsed as part of the options
for (String arg : line.getArgs()) {
files.add(arg);
}
} catch (ParseException exp) {
System.err.println("Parsing failed. Reason: " + exp.getMessage());
printHelp(options);
return null;
}
IBenchmarkRunner runner = basic_runner ? new BasicBenchmarkRunner() : new JMHBenchmarkRunner();
return new CLIInput(files, runner);
}
private static void printHelp(Options options) {
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp("BRunnerCLI", options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy