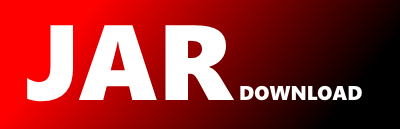
integraal.binding.BRunnerToInteGraalTranslator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brunner-integraal Show documentation
Show all versions of brunner-integraal Show documentation
InteGraal module for the BRunner project
package integraal.binding;
import org.jfree.util.Log;
import api.definition.config.IToolParameters;
import api.definition.config.inputdata.IKBScenario;
import configuration_file_parser.ParserConstants;
import constants.BRunnerKeywords;
import fr.boreal.component_builder.AlgorithmParameters;
import fr.boreal.component_builder.InputDataScenario;
import fr.boreal.component_builder.api.IAlgorithmParameters;
import fr.boreal.component_builder.api.IInputDataScenario;
import fr.boreal.component_builder.api.InteGraalKeywords;
import tools.utils.EnumerationUtils;
class BRunnerToInteGraalTranslator {
/**
*
* Translation from BRunner to InteGraal objects.
*
* @param input kb scenario
* @return the input kb scenario converted into the corresponding InteGraal
* object
*/
static IInputDataScenario translateScenario(IKBScenario input) {
IInputDataScenario scenario = new InputDataScenario("Scenario for InteGraal Created via BRunner ");
var properties = input.getProperties();
for (String propertyName : properties) {
String value = input.getValues(propertyName).stream().findAny().get();
String trimmed = propertyName.replaceFirst(BRunnerKeywords.OuterLevel.INPUTDATA.kw + ".", "");
var e = EnumerationUtils.getEnumFromString(BRunnerKeywords.InnerLevel.class, trimmed);
switch (e) {
case DATA -> {
scenario.setFactbasePath(value);
}
case RULES -> {
scenario.setRulebasePath(value);
}
case WORKLOAD -> {
scenario.setQuerybasePath(value);
}
case MAPPINGS -> {
scenario.setMappingbasePath(value);
}
default -> {
}
}
;
}
return scenario;
}
/**
*
* Internal method to translate from BRunner to InteGraal objects.
*
* @param input algorithm parameters
* @return the input algorithm parameters converted into the corresponding
* InteGraal object
*/
static IAlgorithmParameters translateAlgorithmParameters(IToolParameters params) {
String serviceName = params.getValues(BRunnerKeywords.InnerLevel.SERVICE.full).stream().findAny().get();
InteGraalKeywords.Algorithm service = EnumerationUtils.getEnumFromString(InteGraalKeywords.Algorithm.class,
serviceName);
IAlgorithmParameters algoParam = new AlgorithmParameters(params.getName(), service);
var properties = params.getProperties();
for (String propertyName : properties) {
String trimmed = propertyName.replaceFirst(BRunnerKeywords.OuterLevel.TOOL_PARAMETERS.kw + ".", "");
// service name which is handled apart
if (trimmed.equalsIgnoreCase(BRunnerKeywords.InnerLevel.SERVICE.kw)) {
continue;
}
// parameter id is preferably hidden
if (trimmed.equalsIgnoreCase(BRunnerKeywords.InnerLevel.PARAMETER_ID.kw)) {
continue;
}
// set timeout
if (trimmed.equalsIgnoreCase(BRunnerKeywords.InnerLevel.ALGORITHM_TIMEOUT.kw)) {
algoParam.setTimeout(Integer.parseInt(params.getValues(propertyName).stream().findAny().get()));
continue;
}
// answer cout
if (trimmed.equalsIgnoreCase(InteGraalKeywords.Algorithm.Answers.ANSWER_COUNT_ONLY.toString())) {
algoParam.setAsksCountOnly(
Boolean.parseBoolean(params.getValues(propertyName).stream().findAny().get()));
continue;
}
if (!trimmed.contains(ParserConstants.KEY_DELIMITER)) {
// case of a simple property
@SuppressWarnings("unchecked")
Class extends Enum>> property = (Class extends Enum>>) EnumerationUtils
.findEnumClass(InteGraalKeywords.class, trimmed);
if (params.getValues(propertyName).stream().count() > 1) {
Log.warn("Multiple values for " + propertyName + " : " + params.getValues(propertyName)
+ "\n only the first will be considered");
}
Enum> value = EnumerationUtils.getEnumFromString(property,
params.getValues(propertyName).stream().findAny().get());
algoParam.setParameter(value);
}
if (trimmed.contains(ParserConstants.KEY_DELIMITER)) {
String[] parts = trimmed.split("\\.");
Class extends Enum>> previous = InteGraalKeywords.class;
Class extends Enum>> className = previous;
for (int i = 0; i < parts.length - 1; i++) {
className = (Class extends Enum>>) EnumerationUtils.findEnumClass(previous, parts[i]);
previous = className;
}
var value = params.getValues(propertyName).stream().findAny().get();
try {
// the suffix denotes an enumerated value
Enum> vv = EnumerationUtils.getEnumFromString(className, value);
algoParam.setParameter(vv);
} catch (IllegalArgumentException e) {
// the suffix denotes an enumerated value
algoParam.setParameter(className, parts[parts.length - 1], value);
}
}
}
return algoParam;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy