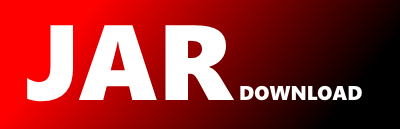
fr.boreal.backward_chaining.pure.PureRewriter Maven / Gradle / Ivy
package fr.boreal.backward_chaining.pure;
import fr.boreal.backward_chaining.api.BackwardChainingAlgorithm;
import fr.boreal.backward_chaining.core.QueryCoreProcessor;
import fr.boreal.backward_chaining.core.QueryCoreProcessorImpl;
import fr.boreal.backward_chaining.cover.CoverFunction;
import fr.boreal.backward_chaining.cover.QueryCover;
import fr.boreal.backward_chaining.homomorphism.QueryHomomorphism;
import fr.boreal.backward_chaining.pure.rewriting_operator.RewritingOperator;
import fr.boreal.backward_chaining.pure.rewriting_operator.SingleRuleAggregator;
import fr.boreal.model.formula.api.FOFormula;
import fr.boreal.model.kb.api.RuleBase;
import fr.boreal.model.query.api.FOQuery;
import fr.boreal.model.query.impl.UnionFOQuery;
import fr.boreal.model.ruleCompilation.NoRuleCompilation;
import fr.boreal.model.ruleCompilation.api.RuleCompilation;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
import java.util.stream.Collectors;
/**
* From Melanie Konïg's thesis, algorithm 1 implementation on Graal v1.3
* Produces a cover of the set of all rewritings of a query Q with a set of
* rules R using piece unifiers.
*
* The given query must represent a CQ or UCQ. That is, a conjunction of atoms without negation (CQ) or a union of CQ (UCQ) with the same answer variables.
*/
public class PureRewriter implements BackwardChainingAlgorithm {
static final Logger LOG = LoggerFactory.getLogger(PureRewriter.class);
private final RewritingOperator rew;
private final CoverFunction coverFct;
private final QueryCoreProcessor core;
private final QueryHomomorphism queryHomomorphism;
/**
* Creates a new PureRewriter using default parameters Rewriting operator SRA
* Cover function query cover
*/
public PureRewriter() {
this(new SingleRuleAggregator(), new QueryCover(), new QueryCoreProcessorImpl());
}
/**
* Creates a new PureRewriter using the given parameters
* @param compilation the rule compilation to use
*/
public PureRewriter(RuleCompilation compilation) {
this(new SingleRuleAggregator(compilation), new QueryCover(compilation), new QueryCoreProcessorImpl(compilation), compilation);
}
/**
* Creates a new PureRewriter using the given parameters
*
* @param rew the rewriting operator to use
* @param coverFct the cover function to use
* @param core the core processor to use
*/
public PureRewriter(RewritingOperator rew, CoverFunction coverFct, QueryCoreProcessor core) {
this(rew, coverFct, core, NoRuleCompilation.instance());
}
/**
* Creates a new PureRewriter using the given parameters
*
* @param rew the rewriting operator to use
* @param coverFct the cover function to use
* @param core the core processor to use
* @param compilation the rule compilation to use
*/
public PureRewriter(RewritingOperator rew, CoverFunction coverFct, QueryCoreProcessor core,
RuleCompilation compilation) {
this.rew = rew;
this.coverFct = coverFct;
this.core = core;
this.queryHomomorphism = new QueryHomomorphism(compilation);
}
@Override
public UnionFOQuery rewrite(UnionFOQuery queries, RuleBase rules) {
Set> finalRewritings = new HashSet<>(queries.getQueries());
Queue> toExploreRewritings = new LinkedList<>(queries.getQueries());
int count = 0;
while (!toExploreRewritings.isEmpty()) {
Set> currentRewritings = toExploreRewritings.parallelStream()
.flatMap(qq -> this.rew.rewrite(qq, rules).stream()).collect(Collectors.toSet());
toExploreRewritings.clear();
currentRewritings = this.coverFct.cover(currentRewritings);
currentRewritings = currentRewritings.parallelStream().map(this.core::computeCore).collect(Collectors.toSet());
// Remove from currentRewritings the queries that are more specific than all the
// final queries
this.removeMoreSpecificQueries(currentRewritings, finalRewritings);
toExploreRewritings.addAll(currentRewritings);
// Remove from finalRewriting the queries that are more specific than all the
// queries just computed
this.removeMoreSpecificQueries(finalRewritings, currentRewritings);
finalRewritings.addAll(currentRewritings);
LOG.debug("rewriting step : {} \tadded rewriting {} ", count, currentRewritings.size());
LOG.debug("added : {}", currentRewritings);
count++;
}
LOG.debug(" total number of rewritings : {} ", finalRewritings.size());
return new UnionFOQuery(finalRewritings);
}
/**
* Removes queries in from that are more specific than queries in filter
*/
private void removeMoreSpecificQueries(Collection> from, Collection> filter) {
from.removeIf(query -> this.containMoreGeneral(filter, query));
}
/**
* return true iff filter contains a query that is more general than the given
* query
*/
private boolean containMoreGeneral(Collection> filter, FOQuery extends FOFormula> query) {
for (FOQuery extends FOFormula> q : filter) {
if (this.queryHomomorphism.exists(q, query)) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy