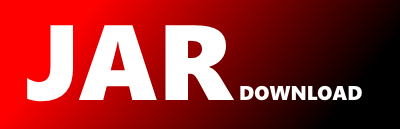
fr.lirmm.boreal.util.converter.QueryConverter Maven / Gradle / Ivy
package fr.lirmm.boreal.util.converter;
import java.util.stream.Collectors;
import com.google.common.collect.BiMap;
import com.google.common.collect.HashBiMap;
import fr.boreal.model.logicalElements.api.Variable;
import fr.boreal.model.query.api.FOQuery;
import fr.boreal.model.query.factory.FOQueryFactory;
import fr.lirmm.graphik.integraal.api.core.ConjunctiveQuery;
/**
* Converts FOQuery to and from Graal's ConjunctiveQuery
*
* This is used for compatibility with Graal 1.3 version.
*
* @author Florent Tornil
*
*/
public class QueryConverter {
private static BiMap cache = HashBiMap.create();
/**
* Converts the given FOQuery into a Graal ConjunctiveQuery
*
* @param query the query to convert
* @return the converted query
*/
public static ConjunctiveQuery convert(FOQuery query) {
ConjunctiveQuery q = cache.get(query);
if (q == null) {
q = fr.lirmm.graphik.integraal.core.factory.DefaultConjunctiveQueryFactory.instance().create(
AtomSetConverter.convert(query.getFormula()),
query.getAnswerVariables().stream().map(TermConverter::convert).collect(Collectors.toList()));
cache.put(query, q);
}
return q;
}
/**
* Converts the given FOQuery into a Graal ConjunctiveQuery
*
* @param query the query to convert
* @return the converted query
*/
public static fr.lirmm.graphik.graal.api.core.ConjunctiveQuery convert2(FOQuery query) {
return fr.lirmm.graphik.graal.core.factory.DefaultConjunctiveQueryFactory.instance().create(
AtomSetConverter.convert2(query.getFormula()),
query.getAnswerVariables().stream().map(TermConverter::convert2).collect(Collectors.toList()));
}
/**
* Converts the given ConjunctiveQuery into a FOQuery
*
* @param query the query to convert
* @return the converted query
*/
public static FOQuery reverse(ConjunctiveQuery query) {
FOQuery q = cache.inverse().get(query);
if (q == null) {
q = FOQueryFactory.instance()
.createOrGetQuery(
AtomSetConverter.reverse(query.getAtomSet()), query.getAnswerVariables().stream()
.map(TermConverter::reverse).map(t -> (Variable) t).collect(Collectors.toList()),
null);
// cache.inverse().put(query, q);
// cannot use cache in this way because hashCode on ConjunctiveQuery is not
// defined
}
return q;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy