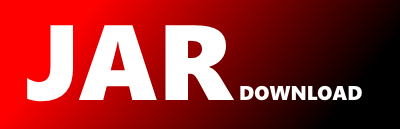
fr.lirmm.boreal.util.converter.TermConverter Maven / Gradle / Ivy
package fr.lirmm.boreal.util.converter;
import fr.boreal.model.logicalElements.api.Constant;
import fr.boreal.model.logicalElements.api.FunctionalTerm;
import fr.boreal.model.logicalElements.api.Literal;
import fr.boreal.model.logicalElements.api.Term;
import fr.boreal.model.logicalElements.api.Variable;
import fr.boreal.model.logicalElements.factory.impl.SameObjectTermFactory;
/**
* Converts Term to and from Graal's Term
*
* This is used for compatibility with Graal 1.3 version.
*
* @author Florent Tornil
*
*/
public class TermConverter {
private static boolean WARNING_PRINTED = false;
/**
* Converts the given Term into a Graal Term
*
* @param t the term to convert
* @return the converted term
*/
public static fr.lirmm.graphik.integraal.api.core.Term convert(Term t) {
if (t instanceof Constant) {
Constant c = (Constant) t;
return fr.lirmm.graphik.integraal.core.term.DefaultTermFactory.instance().createConstant(c.getLabel());
} else if (t instanceof Variable) {
Variable v = (Variable) t;
return fr.lirmm.graphik.integraal.core.term.DefaultTermFactory.instance().createVariable(v.getLabel());
} else if (t instanceof Literal>) {
Literal> l = (Literal>) t;
return fr.lirmm.graphik.integraal.core.term.DefaultTermFactory.instance().createLiteral(l.getValue());
} else if (t instanceof FunctionalTerm) {
if (!WARNING_PRINTED) {
System.err.println(
"[WARNING] : Converted a functional term to a Graal constant : result may not be exact.");
WARNING_PRINTED = true;
}
return fr.lirmm.graphik.integraal.core.term.DefaultTermFactory.instance()
.createConstant("integraal_function");
} else {
return null;
}
}
/**
* Converts the given Term into a Graal Term
*
* @param t the term to convert
* @return the converted term
*/
public static fr.lirmm.graphik.graal.api.core.Term convert2(Term t) {
if (t instanceof Constant) {
Constant c = (Constant) t;
return fr.lirmm.graphik.graal.core.term.DefaultTermFactory.instance().createConstant(c.getLabel());
} else if (t instanceof Variable) {
Variable v = (Variable) t;
return fr.lirmm.graphik.graal.core.term.DefaultTermFactory.instance().createVariable(v.getLabel());
} else if (t instanceof Literal>) {
Literal> l = (Literal>) t;
return fr.lirmm.graphik.graal.core.term.DefaultTermFactory.instance().createLiteral(l.getValue());
} else if (t instanceof FunctionalTerm) {
if (!WARNING_PRINTED) {
System.err.println(
"[WARNING] : Converted a functional term to a Graal constant : result may not be exact.");
WARNING_PRINTED = true;
}
return fr.lirmm.graphik.graal.core.term.DefaultTermFactory.instance().createConstant("integraal_function");
} else {
return null;
}
}
/**
* Converts the given Graal Term into a Term
*
* @param t the term to convert
* @return the converted term
*/
public static Term reverse(fr.lirmm.graphik.integraal.api.core.Term t) {
if (t instanceof fr.lirmm.graphik.integraal.api.core.Constant) {
fr.lirmm.graphik.integraal.api.core.Constant c = (fr.lirmm.graphik.integraal.api.core.Constant) t;
return SameObjectTermFactory.instance().createOrGetConstant(c.getLabel());
} else if (t instanceof fr.lirmm.graphik.integraal.api.core.Variable) {
fr.lirmm.graphik.integraal.api.core.Variable v = (fr.lirmm.graphik.integraal.api.core.Variable) t;
return SameObjectTermFactory.instance().createOrGetVariable(v.getLabel());
} else if (t instanceof fr.lirmm.graphik.integraal.api.core.Literal) {
fr.lirmm.graphik.integraal.api.core.Literal l = (fr.lirmm.graphik.integraal.api.core.Literal) t;
return SameObjectTermFactory.instance().createOrGetLiteral(l.getValue());
} else {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy