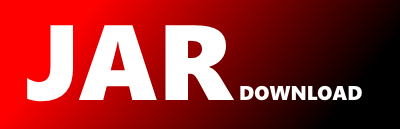
fr.lirmm.boreal.util.evaluator.LazyIterator Maven / Gradle / Ivy
package fr.lirmm.boreal.util.evaluator;
import java.util.Collection;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.function.Function;
/**
* The LazyIterator is a generic iterable that provides a way to lazily process
* elements of a collection using a specified transformation function.
* This allows for deferred execution of the transformation logic, which can be
* beneficial for performance in certain scenarios.
*
* @param the type of elements in the source collection
* @param the type of elements to be returned after transformation
*/
public class LazyIterator implements Iterable {
private final Iterator sourceIterator;
private final Function lazyTransformationFunction;
/**
* Constructs a LazyIterator with the given source collection and transformation function.
*
* @param source the source collection containing elements of type InputType
* @param lazyTransformationFunction the function to apply to each element of the source,
* which transforms an element of type InputType into type ReturnType
*/
public LazyIterator(Collection source, Function lazyTransformationFunction) {
this.sourceIterator = source.iterator();
this.lazyTransformationFunction = lazyTransformationFunction;
}
/**
* Returns an iterator over elements of type ReturnType, each transformed from elements
* of type InputType using the provided transformation function.
*
* @return an Iterator over elements of type ReturnType
*/
@Override
public Iterator iterator() {
return new Iterator() {
/**
* Checks if there are more elements to process.
*
* @return true if the iteration has more elements
*/
@Override
public boolean hasNext() {
return sourceIterator.hasNext();
}
/**
* Returns the next element in the iteration, transformed by the transformation function.
*
* @return the next transformed element of type ReturnType
* @throws NoSuchElementException if the iteration has no more elements
*/
@Override
public ReturnType next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
InputType sourceItem = sourceIterator.next();
return lazyTransformationFunction.apply(sourceItem);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy