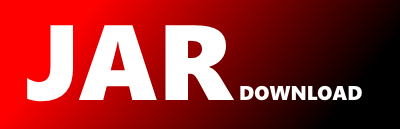
fr.lirmm.boreal.util.evaluator.CallableWithTimeout Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of integraal-util Show documentation
Show all versions of integraal-util Show documentation
Util objects for integraal
package fr.lirmm.boreal.util.evaluator;
import java.time.Duration;
import java.util.Objects;
import java.util.concurrent.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A utility class to execute a Callable with a specified timeout.
* If the timeout expires before the Callable completes, it returns null.
*/
public class CallableWithTimeout {
private static final Logger LOG = LoggerFactory.getLogger(CallableWithTimeout.class);
/**
* Executes a Callable with a specified timeout.
*
* @param task The Callable to execute.
* @param timeout The duration of the timeout ; must not be null;
* @return The result of the Callable, or null if the timeout expires.
*/
public static T execute(Callable task, Duration timeout) {
Objects.requireNonNull(timeout);
ExecutorService executor = Executors.newVirtualThreadPerTaskExecutor();
Future future = executor.submit(task);
try {
return future.get(timeout.toMillis(), TimeUnit.MILLISECONDS);
} catch (TimeoutException e) {
LOG.warn("Task timed out after {} milliseconds", timeout.toMillis());
future.cancel(true);
return null;
} catch (InterruptedException | ExecutionException e) {
LOG.error("An error occurred during task execution", e);
throw new RuntimeException("Error during task execution: " + e.getMessage(), e);
} finally {
executor.shutdownNow();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy