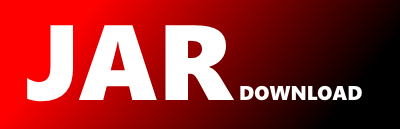
fr.lirmm.boreal.util.externalHaltingConditions.ExternalAlgorithmHaltingConditions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of integraal-util Show documentation
Show all versions of integraal-util Show documentation
Util objects for integraal
package fr.lirmm.boreal.util.externalHaltingConditions;
import java.time.Duration;
import java.util.Arrays;
import java.util.List;
import fr.lirmm.boreal.util.keywords.InteGraalKeywords.Algorithms.ExternalHaltingConditions;
/**
* Defines conditions that must be respected in case of a non-terminating
* algorithm.
*
* @param rank the maximum depth of the reasoning level (e.g., for chase or
* rewriting, the max number of breadth-first reasoning steps).
* Defaults to a fixed value if null.
* @param timeout the maximum duration before the algorithm is forcibly stopped.
* Defaults to a fixed value if null.
*/
public record ExternalAlgorithmHaltingConditions(Integer rank, Duration timeout) {
// Default values
static final Integer DEFAULT_RANK = 1_000_000;
static final Duration DEFAULT_TIMEOUT = Duration.ofHours(1);
/**
* Custom constructor with default handling
*
* @param rank
* @param timeout
*/
public ExternalAlgorithmHaltingConditions(Integer rank, Duration timeout) {
this.rank = (rank == null || rank < 0) ? DEFAULT_RANK : rank;
this.timeout = (timeout == null) ? DEFAULT_TIMEOUT : timeout;
}
/**
* Custom constructor which handles a list of external handling conditions
*
* @param conditions
*/
public ExternalAlgorithmHaltingConditions(List conditions) {
this(extractFirstValue(conditions, ExternalHaltingConditions.RANK),
extractFirstValue(conditions, ExternalHaltingConditions.TIMEOUT));
}
/**
* Custom constructor which handles an array of external handling conditions
*
* @param conds
*/
public ExternalAlgorithmHaltingConditions(ExternalHaltingCondition[] conds) {
this(Arrays.asList(conds));
}
/**
* Returns a new instance of {@code ExternalAlgorithmHaltingConditions} with
* optional modifications.
*
* @param newRank the new rank to set, or {@code null} to keep the existing
* rank.
* @param newTimeout the new timeout to set, or {@code null} to keep the
* existing timeout.
* @return a new {@code ExternalAlgorithmHaltingConditions} instance with the
* modified values.
*/
public ExternalAlgorithmHaltingConditions withModified(Integer newRank, Duration newTimeout) {
return new ExternalAlgorithmHaltingConditions((newRank == null) ? this.rank : newRank,
(newTimeout == null) ? this.timeout : newTimeout);
}
/**
* Returns the default {@code ExternalAlgorithmHaltingConditions} instance with
* default rank and timeout values.
*
* @return a default instance of {@code ExternalAlgorithmHaltingConditions}.
*/
public static ExternalAlgorithmHaltingConditions defaultConditions() {
return new ExternalAlgorithmHaltingConditions(DEFAULT_RANK, DEFAULT_TIMEOUT);
}
/**
* Extracts the (first!) value from the list of conditions.
*
* @param conditions the list of conditions
* @return the extracted rank as a Long, or null if not found
*/
private static T extractFirstValue(List conditions,
ExternalHaltingConditions enumValue) {
if (conditions == null) {
return null;
}
for (ExternalHaltingCondition condition : conditions) {
if (condition.keyword() == enumValue) {
return condition.getValue();
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy